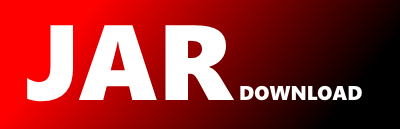
cn.sliew.carp.module.application.vela.api.v1.PipelineApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.sliew.carp.module.application.vela.api.v1;
import cn.sliew.carp.module.application.vela.api.v1.model.v1.*;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
public interface PipelineApi {
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/contexts")
ResponseEntity listContextValues(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName);
@PostMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/contexts")
ResponseEntity createContextValue(@RequestBody V1CreateContextValuesRequest body, @PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName);
@PutMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/contexts/{contextName}")
ResponseEntity updateContextValue(@RequestBody V1UpdateContextValuesRequest body, @PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("contextName") String contextName);
@DeleteMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/contexts/{contextName}")
ResponseEntity deleteContextValue(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("contextName") String contextName);
@GetMapping(value = "/api/v1/pipelines")
ResponseEntity listPipelines(@RequestParam(value = "query", required = false) String query, @RequestParam(value = "projectName", required = false) String projectName, @RequestParam(value = "detailed", required = false, defaultValue = "true") Boolean detailed);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}")
ResponseEntity getPipeline(@PathVariable("pipelineName") String pipelineName, @PathVariable("projectName") String projectName);
@PostMapping(value = "/api/v1/projects/{projectName}/pipelines")
ResponseEntity createPipeline(@RequestBody V1CreatePipelineRequest body, @PathVariable("projectName") String projectName);
@PutMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}")
ResponseEntity updatePipeline(@RequestBody V1UpdatePipelineRequest body, @PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName);
@DeleteMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}")
ResponseEntity deletePipeline(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs")
ResponseEntity listPipelineRuns(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @RequestParam(value = "status", required = false) String status);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}")
ResponseEntity getPipelineRun(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/input")
ResponseEntity getPipelineRunInput(@RequestParam(value = "step", required = true) String step, @PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/output")
ResponseEntity getPipelineRunOutput(@RequestParam(value = "step", required = true) String step, @PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/log")
ResponseEntity getPipelineRunLog(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName, @RequestParam(value = "step", required = false) String step);
@GetMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/status")
ResponseEntity getPipelineRunStatus(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
@PostMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/run")
ResponseEntity runPipeline(@RequestBody V1RunPipelineRequest body, @PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName);
@PostMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/stop")
ResponseEntity stopPipeline(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
@PostMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/resume")
ResponseEntity resumePipelineRun(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName, @RequestParam(value = "step", required = false) String step);
@PostMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}/terminate")
ResponseEntity terminatePipelineRun(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
@DeleteMapping(value = "/api/v1/projects/{projectName}/pipelines/{pipelineName}/runs/{runName}")
ResponseEntity deletePipelineRun(@PathVariable("projectName") String projectName, @PathVariable("pipelineName") String pipelineName, @PathVariable("runName") String runName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy