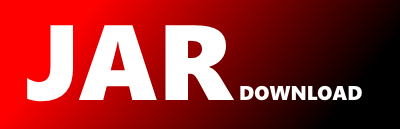
cn.starboot.socket.plugins.channels.AsynchronousSocketChannelProxy Maven / Gradle / Ivy
/*
* Copyright 2019 The aio-socket Project
*
* The aio-socket Project Licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.starboot.socket.plugins.channels;
import java.io.IOException;
import java.net.SocketAddress;
import java.net.SocketOption;
import java.nio.ByteBuffer;
import java.nio.channels.AsynchronousSocketChannel;
import java.nio.channels.CompletionHandler;
import java.util.Set;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
/**
* AIO 流控与SSL插件专用
*
* @author MDong
* @version 2.10.1.v20211002-RELEASE
*/
public class AsynchronousSocketChannelProxy extends AsynchronousSocketChannel {
protected final AsynchronousSocketChannel asynchronousSocketChannel;
public AsynchronousSocketChannelProxy(AsynchronousSocketChannel asynchronousSocketChannel) {
super(asynchronousSocketChannel.provider());
this.asynchronousSocketChannel = asynchronousSocketChannel;
}
@Override
public AsynchronousSocketChannel bind(SocketAddress local) throws IOException {
return asynchronousSocketChannel.bind(local);
}
@Override
public AsynchronousSocketChannel setOption(SocketOption name, T value) throws IOException {
return asynchronousSocketChannel.setOption(name, value);
}
@Override
public T getOption(SocketOption name) throws IOException {
return asynchronousSocketChannel.getOption(name);
}
@Override
public Set> supportedOptions() {
return asynchronousSocketChannel.supportedOptions();
}
@Override
public AsynchronousSocketChannel shutdownInput() throws IOException {
return asynchronousSocketChannel.shutdownInput();
}
@Override
public AsynchronousSocketChannel shutdownOutput() throws IOException {
return asynchronousSocketChannel.shutdownOutput();
}
@Override
public SocketAddress getRemoteAddress() throws IOException {
return asynchronousSocketChannel.getRemoteAddress();
}
@Override
public void connect(SocketAddress remote, A attachment, CompletionHandler handler) {
asynchronousSocketChannel.connect(remote, attachment, handler);
}
@Override
public Future connect(SocketAddress remote) {
return asynchronousSocketChannel.connect(remote);
}
@Override
public void read(ByteBuffer dst, long timeout, TimeUnit unit, A attachment, CompletionHandler handler) {
asynchronousSocketChannel.read(dst, timeout, unit, attachment, handler);
}
@Override
public Future read(ByteBuffer dst) {
return asynchronousSocketChannel.read(dst);
}
@Override
public void read(ByteBuffer[] dsts, int offset, int length, long timeout, TimeUnit unit, A attachment, CompletionHandler handler) {
asynchronousSocketChannel.read(dsts, offset, length, timeout, unit, attachment, handler);
}
@Override
public void write(ByteBuffer src, long timeout, TimeUnit unit, A attachment, CompletionHandler handler) {
asynchronousSocketChannel.write(src, timeout, unit, attachment, handler);
}
@Override
public Future write(ByteBuffer src) {
return asynchronousSocketChannel.write(src);
}
@Override
public void write(ByteBuffer[] srcs, int offset, int length, long timeout, TimeUnit unit, A attachment, CompletionHandler handler) {
asynchronousSocketChannel.write(srcs, offset, length, timeout, unit, attachment, handler);
}
@Override
public SocketAddress getLocalAddress() throws IOException {
return asynchronousSocketChannel.getLocalAddress();
}
@Override
public boolean isOpen() {
return asynchronousSocketChannel.isOpen();
}
@Override
public void close() throws IOException {
asynchronousSocketChannel.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy