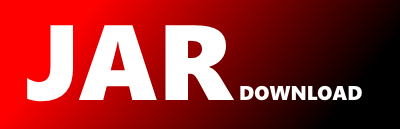
infra.beans.factory.config.ServiceLocatorFactoryBean Maven / Gradle / Ivy
/*
* Copyright 2017 - 2024 the original author or authors.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see [https://www.gnu.org/licenses/]
*/
package infra.beans.factory.config;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.util.Properties;
import infra.beans.BeanUtils;
import infra.beans.BeansException;
import infra.beans.factory.BeanFactory;
import infra.beans.factory.BeanFactoryAware;
import infra.beans.factory.FactoryBean;
import infra.beans.factory.InitializingBean;
import infra.beans.factory.NoSuchBeanDefinitionException;
import infra.lang.Assert;
import infra.lang.Constant;
import infra.lang.Nullable;
import infra.util.ReflectionUtils;
import infra.util.StringUtils;
/**
* A {@link FactoryBean} implementation that takes an interface which must have one or more
* methods with the signatures {@code MyType xxx()} or {@code MyType xxx(MyIdType id)}
* (typically, {@code MyService getService()} or {@code MyService getService(String id)})
* and creates a dynamic proxy which implements that interface, delegating to an
* underlying {@link BeanFactory}.
*
* Such service locators permit the decoupling of calling code from
* the {@link BeanFactory} API, by using an
* appropriate custom locator interface. They will typically be used for
* prototype beans, i.e. for factory methods that are supposed to
* return a new instance for each call. The client receives a reference to the
* service locator via setter or constructor injection, to be able to invoke
* the locator's factory methods on demand. For singleton beans, direct
* setter or constructor injection of the target bean is preferable.
*
*
On invocation of the no-arg factory method, or the single-arg factory
* method with a String id of {@code null} or empty String, if exactly
* one bean in the factory matches the return type of the factory
* method, that bean is returned, otherwise a {@link NoSuchBeanDefinitionException}
* is thrown.
*
*
On invocation of the single-arg factory method with a non-null (and
* non-empty) argument, the proxy returns the result of a
* {@link BeanFactory#getBean(String)} call, using a stringified version
* of the passed-in id as bean name.
*
*
A factory method argument will usually be a String, but can also be an
* int or a custom enumeration type, for example, stringified via
* {@code toString}. The resulting String can be used as bean name as-is,
* provided that corresponding beans are defined in the bean factory.
* Alternatively, {@linkplain #setServiceMappings(java.util.Properties) a custom
* mapping} between service IDs and bean names can be defined.
*
*
By way of an example, consider the following service locator interface.
* Note that this interface is not dependent on any Framework APIs.
*
*
package a.b.c;
*
* public interface ServiceFactory {
*
* public MyService getService();
* }
*
* A sample config in an XML-based {@link BeanFactory} might look as follows:
*
*
<beans>
*
* <!-- Prototype bean since we have state -->
* <bean id="myService" class="a.b.c.MyService" singleton="false"/>
*
* <!-- will lookup the above 'myService' bean by *TYPE* -->
* <bean id="myServiceFactory"
* class="infra.beans.factory.config.ServiceLocatorFactoryBean">
* <property name="serviceLocatorInterface" value="a.b.c.ServiceFactory"/>
* </bean>
*
* <bean id="clientBean" class="a.b.c.MyClientBean">
* <property name="myServiceFactory" ref="myServiceFactory"/>
* </bean>
*
* </beans>
*
* The attendant {@code MyClientBean} class implementation might then
* look something like this:
*
*
package a.b.c;
*
* public class MyClientBean {
*
* private ServiceFactory myServiceFactory;
*
* // actual implementation provided by the IoC
* public void setServiceFactory(ServiceFactory myServiceFactory) {
* this.myServiceFactory = myServiceFactory;
* }
*
* public void someBusinessMethod() {
* // get a 'fresh', brand new MyService instance
* MyService service = this.myServiceFactory.getService();
* // use the service object to effect the business logic...
* }
* }
*
* By way of an example that looks up a bean by name, consider
* the following service locator interface. Again, note that this
* interface is not dependent on any Framework APIs.
*
*
package a.b.c;
*
* public interface ServiceFactory {
*
* public MyService getService (String serviceName);
* }
*
* A sample config in an XML-based {@link BeanFactory} might look as follows:
*
*
<beans>
*
* <!-- Prototype beans since we have state (both extend MyService) -->
* <bean id="specialService" class="a.b.c.SpecialService" singleton="false"/>
* <bean id="anotherService" class="a.b.c.AnotherService" singleton="false"/>
*
* <bean id="myServiceFactory"
* class="infra.beans.factory.config.ServiceLocatorFactoryBean">
* <property name="serviceLocatorInterface" value="a.b.c.ServiceFactory"/>
* </bean>
*
* <bean id="clientBean" class="a.b.c.MyClientBean">
* <property name="myServiceFactory" ref="myServiceFactory"/>
* </bean>
*
* </beans>
*
* The attendant {@code MyClientBean} class implementation might then
* look something like this:
*
*
package a.b.c;
*
* public class MyClientBean {
*
* private ServiceFactory myServiceFactory;
*
* // actual implementation provided by the IoC
* public void setServiceFactory(ServiceFactory myServiceFactory) {
* this.myServiceFactory = myServiceFactory;
* }
*
* public void someBusinessMethod() {
* // get a 'fresh', brand new MyService instance
* MyService service = this.myServiceFactory.getService("specialService");
* // use the service object to effect the business logic...
* }
*
* public void anotherBusinessMethod() {
* // get a 'fresh', brand new MyService instance
* MyService service = this.myServiceFactory.getService("anotherService");
* // use the service object to effect the business logic...
* }
* }
*
* See {@link SupplierFactoryCreatingFactoryBean} for an alternate approach.
*
* @author Colin Sampaleanu
* @author Juergen Hoeller
* @author Harry Yang
* @see #setServiceLocatorInterface
* @see #setServiceMappings
* @see SupplierFactoryCreatingFactoryBean
* @since 4.0 2021/11/30 14:26
*/
public class ServiceLocatorFactoryBean implements FactoryBean