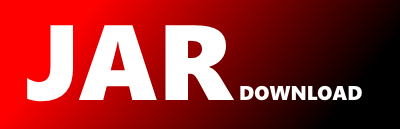
cn.tangjiabao.halodb.utils.file.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of haloDb Show documentation
Show all versions of haloDb Show documentation
Orm whitch The highest development efficiency and Efficiency is the fastest
package cn.tangjiabao.halodb.utils.file;
import java.io.File;
import java.io.UnsupportedEncodingException;
import java.net.URL;
import java.net.URLDecoder;
import org.apache.log4j.Logger;
import cn.tangjiabao.halodb.core.exception.MyRuntimeException;
import cn.tangjiabao.halodb.utils.exception.ExceptionUtils;
import cn.tangjiabao.halodb.utils.logger.LogUtils;
import cn.tangjiabao.halodb.utils.string.StringUtils;
/**
* 文件组件
* @author [email protected]
* @date 2015-6-14 下午10:33:39
*/
public class FileUtils {
private static Logger logger= Logger.getLogger(FileUtils.class);
private ClassLoader classLoader;
public ClassLoader getClassLoader() {
return classLoader;
}
public void setClassLoader(ClassLoader classLoader) {
this.classLoader = classLoader;
}
public static String getEncoding() {
return ENCODING;
}
private static final String ENCODING = "UTF-8";
private static String getFilePath(String packageName, String name) {
String path = packageName.replaceAll("\\.", "\\"+File.separator);
return path + File.separator + name;//"/" +
}
public static File getClassPath(String packageName, String name) {
String path =Thread.currentThread().getContextClassLoader()
.getResource("").getPath();
try {
path=URLDecoder.decode(path,ENCODING);
} catch (UnsupportedEncodingException e) {
logger.error(ExceptionUtils.getStackTrace(e));
}
File file = new File(path+getFilePath(packageName, name));
return file;
}
/**
* 获得类文件
* @param clazz
* @return File
*/
public static File getClassPath(Class> clazz) {
String className=clazz.getSimpleName()+".class";
String path =clazz.getResource(className).getPath();
logger.debug(LogUtils.format("classpath",path));
try {
path=URLDecoder.decode(path,ENCODING);
} catch (UnsupportedEncodingException e) {
logger.error(ExceptionUtils.getStackTrace(e));
}
File file = new File(path);
return file;
}
public static File getClassPath(Class> clazz,String fileName) {
URL pathUrl =clazz.getResource(fileName);
if(null==pathUrl){
throw MyRuntimeException.Instance(fileName,"文件不存在!");
}
String path=pathUrl.getPath();
logger.debug(LogUtils.format("classpath",path));
try {
path=URLDecoder.decode(path,ENCODING);
} catch (UnsupportedEncodingException e) {
logger.error(ExceptionUtils.getStackTrace(e));
}
File file = new File(path);
return file;
}
/**
* 过滤输出文件:如果没有路径文件夹 创建之
* @param outFile
* @return File
*/
public static File newOutFile(File outFile) {
return createFolder(outFile);
}
public static File newOutFile(String outfilePath) {
return createFolder(new File(outfilePath));
}
public static File createFolder(File targetFile) {
String path = targetFile.getAbsolutePath();
String folder = StringUtils.substringBefore(path, targetFile.getName());
File folderFile = new File(folder);
if (!folderFile.exists()) {
folderFile.mkdirs();
}
return targetFile;
}
/**
* 创建文件夹
* @param folderPath
*/
public static void newFolder(String folderPath) {
try {
File folder = new File(folderPath);
if (!folder.exists()) {
folder.mkdir();
}
} catch (Exception e) {
logger.error(ExceptionUtils.getStackTrace(e));
}
}
/**
* 创建并获得路径
* @param folderPath
* @return folderPath
*/
public static String getFolderPath(String folderPath) {
File folder = null;
try {
folder = new File(folderPath);
if (!folder.exists()) {
folder.mkdirs();
}
} catch (Exception e) {
logger.error(ExceptionUtils.getStackTrace(e));
}
return folder.getAbsolutePath();
}
/**
* 取指定文件的扩展名
* @param filePathName
* 文件路径
* @return 扩展名
*/
public static String getFileExt(String filePathName) {
int pos = 0;
pos = filePathName.lastIndexOf('.');
if (pos != -1)
return filePathName.substring(pos + 1, filePathName.length());
else
return "";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy