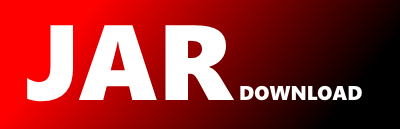
cn.tenfell.plugins.dbgenerate.entity.DefaultAnnotation Maven / Gradle / Ivy
package cn.tenfell.plugins.dbgenerate.entity;
import cn.hutool.core.util.ClassUtil;
import cn.hutool.core.util.ReflectUtil;
import cn.tenfell.plugins.dbgenerate.annotation.Table;
import cn.tenfell.plugins.dbgenerate.annotation.TableColumn;
import java.lang.reflect.Field;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.sql.JDBCType;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
@Table
public class DefaultAnnotation {
private static Map tableColumnMap;
private static Table table;
@TableColumn(type = JDBCType.TIMESTAMP,length = 26)
Date dateField;
@TableColumn(type = JDBCType.INTEGER,length = 10)
Integer integerField;
@TableColumn(type = JDBCType.FLOAT,length = 15,decimal = 5)
Float floatField;
@TableColumn(type = JDBCType.DOUBLE,length = 15,decimal = 5)
Double doubleField;
@TableColumn(type = JDBCType.DATE,length = 10)
LocalDate localDateField;
@TableColumn(type = JDBCType.TIMESTAMP,length = 26)
LocalDateTime localDateTimeField;
@TableColumn(type = JDBCType.DECIMAL,length = 19)
Long longField;
@TableColumn(type = JDBCType.SMALLINT,length = 5)
Short shortField;
@TableColumn(type = JDBCType.TINYINT,length = 3)
Byte byteField;
@TableColumn(type = JDBCType.CHAR,length = 1)
Character characterField;
@TableColumn(type = JDBCType.VARCHAR)
String stringField;
@TableColumn(type = JDBCType.BOOLEAN,length = 1)
Boolean booleanField;
@TableColumn(type = JDBCType.DECIMAL,length = 15,decimal = 5)
BigDecimal bigDecimalField;
@TableColumn(type = JDBCType.VARCHAR)
BigInteger bigIntegerField;
public static Table table(){
if(table == null){
table = DefaultAnnotation.class.getAnnotation(Table.class);
}
return table;
}
public static Map tableColumnMap(){
if(tableColumnMap == null){
tableColumnMap = new HashMap<>();
Field[] fields = ReflectUtil.getFields(DefaultAnnotation.class);
for (Field field:fields){
TableColumn tableColumn = field.getAnnotation(TableColumn.class);
if(tableColumn == null){
continue;
}
Class clazz = (Class)field.getGenericType();
tableColumnMap.put(clazz.getName(),tableColumn);
if(!ClassUtil.isBasicType(clazz)){
continue;
}
Class type = (Class)ReflectUtil.getFieldValue(clazz,"TYPE");
if(type == clazz){
continue;
}
tableColumnMap.put(type.getName(),tableColumn);
}
}
return tableColumnMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy