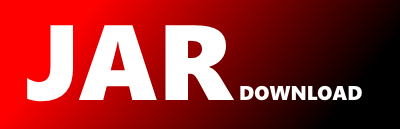
cn.tenfell.tools.nocontroller.component.UriHandComponent Maven / Gradle / Ivy
The newest version!
package cn.tenfell.tools.nocontroller.component;
import cn.hutool.core.thread.ThreadUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.json.JSONUtil;
import cn.tenfell.tools.nocontroller.annotation.InterfaceDoc;
import cn.tenfell.tools.nocontroller.annotation.InterfaceModule;
import cn.tenfell.tools.nocontroller.annotation.NeedLogin;
import cn.tenfell.tools.nocontroller.inface.NoControllerInterface;
import cn.tenfell.tools.nocontroller.utils.AopTargetUtils;
import cn.tenfell.tools.nocontroller.utilsentity.*;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Service;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.lang.reflect.Method;
import java.text.SimpleDateFormat;
import java.util.*;
@Component
public class UriHandComponent implements ApplicationContextAware {
private static List interfaceList;
private static String interfaceCreateTime;
public static final Map handMap = new HashMap<>();
private static NoControllerInterface noControllerInterface;
private static ApplicationContext applicationContext;
private static Map serviceCacheDataMap = new HashMap<>();
@Autowired
private NoControllerInterface noControllerInterfaceService;
public static NoControllerInterface getNoControllerInterface(){
return noControllerInterface;
}
public static PoData getDocData(){
PoData data = PoData.create()
.set("list",UriHandComponent.interfaceList)
.set("time",UriHandComponent.interfaceCreateTime);
return data;
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
UriHandComponent.applicationContext=applicationContext;
UriHandComponent.noControllerInterface = noControllerInterfaceService;
updateHandMap(null);
}
private static void updateHandMapOneService(Object service,String key){
String[] conMappings = {"json","jsonp"};
//原对象,用于获取注解
Object serviceTarget = AopTargetUtils.getTarget(service);
ServiceCacheData serviceCacheData = new ServiceCacheData();
serviceCacheData.setService(serviceTarget);
serviceCacheData.setServiceName(key);
List methodList = new ArrayList();
Method[] methods = serviceTarget.getClass().getDeclaredMethods();
for(Method method:methods){
Class>[] types = method.getParameterTypes();
boolean hasMethod = false;
int type = getType(types);
if(type == 1){
hasMethod = true;
//表示要处理的json,jsonp
UriMap uriMap = new UriMap();
uriMap.setService(service);
uriMap.setMethod(method);
uriMap.setParams(types);
NeedLogin needLogin = method.getAnnotation(NeedLogin.class);
uriMap.setNeedLogin(needLogin != null);
for(String conMapping:conMappings){
String mapKey = "/"+conMapping+"/"+key+"/"+method.getName();
handMap.put(mapKey,uriMap);
}
}else if(type == 2){
hasMethod = true;
//表示要处理的selevt
UriMap uriMap = new UriMap();
uriMap.setService(service);
uriMap.setMethod(method);
uriMap.setParams(types);
NeedLogin needLogin = method.getAnnotation(NeedLogin.class);
uriMap.setNeedLogin(needLogin != null);
String mapKey = "/selevt/"+key+"/"+method.getName();
handMap.put(mapKey,uriMap);
}
if(hasMethod){
methodList.add(method);
}
}
serviceCacheData.setMethods(methodList);
serviceCacheDataMap.put(key,serviceCacheData);
}
public static void updateHandMap(String serviceName){
Map serviceMap = applicationContext.getBeansWithAnnotation(Service.class);
if(StrUtil.isBlank(serviceName)){
/*初始化*/
Set keys = serviceMap.keySet();
for(String key:keys){
Object service = serviceMap.get(key);
updateHandMapOneService(service,key);
}
}else{
/*更新*/
Object service = serviceMap.get(serviceName);
if(service == null){
return;
}
updateHandMapOneService(service,serviceName);
}
ThreadUtil.execute(new Runnable() {
@Override
public void run() {
updateInterfaceList();
}
});
}
private static void updateInterfaceList(){
interfaceList = new ArrayList<>();
String[] conMappings = {"json","jsonp"};
Set keys = serviceCacheDataMap.keySet();
for(String key:keys){
ServiceCacheData serviceCacheData = serviceCacheDataMap.get(key);
InterfaceDocEntity interfaceDocEntity = null;
List interfaceDocChildren = null;
Object service = serviceCacheData.getService();
List methodList = serviceCacheData.getMethods();
for(Method method:methodList){
Class>[] types = method.getParameterTypes();
InterfaceDoc interfaceDoc = method.getAnnotation(InterfaceDoc.class);
int type = getType(types);
boolean hasDoc = interfaceDoc != null && (type==1 || type==2);
if(!hasDoc){
continue;
}
if(interfaceDocEntity == null){
interfaceDocEntity = new InterfaceDocEntity();
interfaceDocChildren = new ArrayList();
String moduleName = serviceCacheData.getServiceName();
InterfaceModule interfaceModule = service.getClass().getAnnotation(InterfaceModule.class);
if(interfaceModule != null && !"".equals(interfaceModule.value())){
moduleName = interfaceModule.value();
}
interfaceDocEntity.setName(moduleName);
}
InterfaceDocChild interfaceDocChild = new InterfaceDocChild();
try{
Object returnObj = method.getReturnType().newInstance();
interfaceDocChild.setReturnData(JSONUtil.toJsonStr(returnObj));
}catch (Exception e){
}
String interfaceName = method.getName();
if(interfaceDoc != null && !"".equals(interfaceDoc.name())){
interfaceName = interfaceDoc.name();
}
interfaceDocChild.setName(interfaceName);
List interfaceParamsList = new ArrayList();
String[] params = interfaceDoc.params();
for(String param:params){
if(param == null || "".equals(param.trim())){
continue;
}
String[] paramValues = param.split(interfaceDoc.separator(),3);
if(paramValues.length != 3){
continue;
}
InterfaceParams interfaceParams = new InterfaceParams();
interfaceParams.setParam(paramValues[0]);
interfaceParams.setIsNeed(paramValues[1]);
interfaceParams.setParamDesc(paramValues[2]);
interfaceParamsList.add(interfaceParams);
}
interfaceDocChild.setParams(interfaceParamsList);
List urlList = new ArrayList();
if(type == 1){
//表示要处理的json,jsonp
for(String conMapping:conMappings){
String mapKey = "/"+conMapping+"/"+serviceCacheData.getServiceName()+"/"+method.getName();
urlList.add(mapKey);
}
}else if(type == 2){
//表示要处理的selevt
String mapKey = "/selevt/"+serviceCacheData.getServiceName()+"/"+method.getName();
urlList.add(mapKey);
}
interfaceDocChild.setUrlList(urlList);
interfaceDocChildren.add(interfaceDocChild);
}
if(interfaceDocEntity != null){
interfaceDocEntity.setList(interfaceDocChildren);
interfaceList.add(interfaceDocEntity);
}
}
interfaceCreateTime = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date());
}
private static int getType(Class>[] types){
int type = 0;
if((types.length == 1 || types.length == 2) && types[0] == PoData.class){
type = 1;
}else if((types.length == 2 || types.length == 3) && types[0] == HttpServletRequest.class && types[1] == HttpServletResponse.class){
type = 2;
}
return type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy