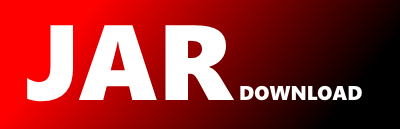
cn.twelvet.idempotent.aspect.IdempotentAspect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of idempotent-spring-boot-starter Show documentation
Show all versions of idempotent-spring-boot-starter Show documentation
Importing and exporting Excel based on easyexcel annotation
package cn.twelvet.idempotent.aspect;
import cn.twelvet.idempotent.annotation.Idempotent;
import cn.twelvet.idempotent.exception.IdempotentException;
import cn.twelvet.idempotent.expression.KeyResolver;
import javax.servlet.http.HttpServletRequest;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.redisson.Redisson;
import org.redisson.api.RMapCache;
import org.redisson.api.RedissonClient;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import java.lang.reflect.Method;
import java.time.LocalDateTime;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
/**
* The Idempotent Aspect
*
* @author twelvet
*/
@Aspect
public class IdempotentAspect {
private static final Logger LOGGER = LoggerFactory.getLogger(IdempotentAspect.class);
private static final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy