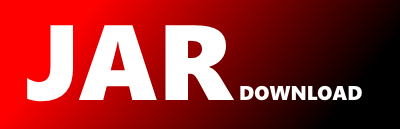
io.vertx.up.atom.agent.Depot Maven / Gradle / Ivy
package io.vertx.up.atom.agent;
import io.vertx.up.eon.ID;
import io.vertx.up.uca.rs.Filler;
import io.vertx.up.util.Ut;
import java.io.Serializable;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.concurrent.ConcurrentMap;
/**
* FeignDepot to extract the method parameters
*/
public class Depot implements Serializable {
private final List paramNames = new ArrayList<>();
private final Event event;
private final List> paramAnnos = new ArrayList<>();
private List> paramTypes = new ArrayList<>();
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy