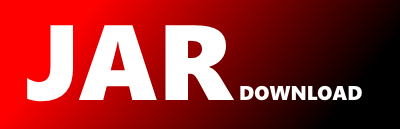
io.vertx.up.commune.ActiveRecord Maven / Gradle / Ivy
package io.vertx.up.commune;
import io.vertx.core.json.JsonObject;
import io.vertx.up.log.Annal;
import io.vertx.up.util.Ut;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
/*
* Provide abstract record to avoid more writing method of record
* Each extension record could inherit from this
*/
public abstract class ActiveRecord implements Record {
/*
* The core data structure to store data in json format
*/
private final transient JsonObject data = new JsonObject();
protected Annal getLogger() {
return Annal.get(this.getClass());
}
/*
* This method is used in sub-class only
*/
protected JsonObject data() {
return this.data;
}
// -------------- Data Checked ---------------
/*
* Whether data is empty
*/
@Override
public boolean isEmpty() {
return this.data.isEmpty();
}
@Override
public boolean isPersist() {
// TODO: This feature is for future usage
return false;
}
// -------------- Data Get/Set ---------------
/*
* Get the value by field ( field = value ) of record
*/
@Override
@SuppressWarnings("unchecked")
public T get(final String field) {
final Object value = this.data.getValue(field);
return (T) value;
}
/*
* Get sub json by fields ( field1 = value1, field2 = value2 ) of record
* Be careful: include `null`
*/
@Override
public JsonObject get(final String... fields) {
final JsonObject json = new JsonObject();
Arrays.stream(fields).forEach(each -> json.put(each, this.data.getValue(each)));
return json;
}
/*
* The same as `toJson()`, return to all data of current record
*/
@Override
public JsonObject get() {
return this.toJson();
}
/*
* Set single pair `field = value`
*/
@Override
public Record set(final String field, final V value) {
if (this.declaredFields().contains(field)) {
this.data.put(field, value);
} else {
this.getLogger().warn("[ ZERO ] The field {0} has not been declared.", field);
}
return this;
}
/*
* Set all data from `JsonObject`
*/
@Override
public Record set(final JsonObject data) {
if (!Ut.isNil(data)) {
data.stream().filter(Objects::nonNull)
.forEach(entry -> this.set(entry.getKey(), entry.getValue()));
}
return this;
}
/*
* Add `field = value` when the original data value of field is `null`
*/
@Override
public Record add(final String field, final V value) {
if (null == this.data.getValue(field)) {
this.set(field, value);
}
return this;
}
/*
* Add `JsonObject` with add
*/
@Override
public Record add(final JsonObject data) {
if (Ut.notNil(data)) {
data.stream().filter(Objects::nonNull)
.forEach(entry -> this.add(entry.getKey(), entry.getValue()));
}
return this;
}
/*
* Remove by `field`
*/
@Override
public Record remove(final String field) {
if (this.data.containsKey(field)) {
this.data.remove(field);
}
return this;
}
/*
* Remove by `fields`
*/
@Override
public Record remove(final String... fields) {
Arrays.stream(fields).forEach(this::remove);
return this;
}
// -------------- Data Clone ---------------
/*
* Clone subset of current `record`
*/
@Override
public Record createSubset(final String... fields) {
/*
* Call createNew() record here, you must set new record created instead of other
* method. Different record has different creation methods.
* */
final Record record = this.createNew();
Arrays.stream(fields).forEach(field -> record.set(field, this.get(field)));
return record;
}
/*
* Clone current record
*/
@Override
public Record createCopy() {
final Record record = this.createNew();
record.set(this.data);
return record;
}
// --------------- Json interface ------------
/*
* It does not contain `null` value here.
*/
@Override
public JsonObject toJson() {
final JsonObject json = this.data.copy();
this.data.fieldNames().stream()
.filter(field -> Objects.isNull(json.getValue(field)))
.forEach(json::remove);
return json;
}
@Override
public void fromJson(final JsonObject json) {
if (Ut.notNil(json)) {
this.data.mergeIn(json);
}
}
// ---------------- Meta Data ----------------
/*
* Get data size
*/
@Override
public int size() {
return this.data.size();
}
/*
* Get data field names
*/
@Override
public Set fields() {
return this.data.fieldNames();
}
// ---------------- Optional Overwrite ------
@Override
public Set joins() {
return new HashSet<>();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy