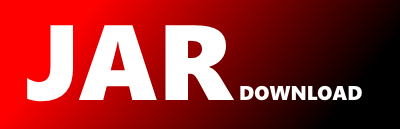
io.vertx.up.runtime.ZeroMotor Maven / Gradle / Ivy
package io.vertx.up.runtime;
import io.vertx.core.ClusterOptions;
import io.vertx.core.VertxOptions;
import io.vertx.core.eventbus.EnvelopCodec;
import io.vertx.core.eventbus.EventBus;
import io.vertx.core.spi.cluster.ClusterManager;
import io.vertx.up.commune.Envelop;
import io.vertx.up.eon.Info;
import io.vertx.up.eon.em.ServerType;
import io.vertx.up.fn.Fn;
import io.vertx.up.log.Annal;
import io.vertx.up.util.Ut;
import io.vertx.zero.exception.VertxCallbackException;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
/**
* Start up tools shared in
* Web Application & Rx Application
*/
public final class ZeroMotor {
private static final Annal LOGGER = Annal.get(ZeroMotor.class);
public static void start(
final Class> clazz,
final Consumer consumer,
final Consumer> fnSingle,
final BiConsumer> fnCluster,
final Annal logger) {
if (null == consumer) {
throw new VertxCallbackException(clazz);
}
// 1. Check if clustered mode
final ClusterOptions cluster = ZeroGrid.getClusterOption();
if (cluster.isEnabled()) {
// 2.1. Clustered
final ClusterManager manager = cluster.getManager();
logger.info(Info.APP_CLUSTERD, manager.getClass().getName(),
manager.getNodeID(), manager.isActive());
fnCluster.accept(manager, consumer);
} else {
// 2.2. Standalone
fnSingle.accept(consumer);
}
}
public static void each(
final BiConsumer consumer) {
final ConcurrentMap vertxOptions
= ZeroGrid.getVertxOptions();
vertxOptions.forEach(consumer);
}
public static void codec(final EventBus eventBus) {
eventBus.registerDefaultCodec(Envelop.class,
Ut.singleton(EnvelopCodec.class));
}
/**
* Agent calculation
*
* @param defaultAgents
* @param internals
* @return
*/
public static ConcurrentMap> agents(
final ServerType category,
final Class>[] defaultAgents,
final ConcurrentMap> internals
) {
final ConcurrentMap>> agents =
getMergedAgents(category, internals);
final ConcurrentMap defines =
ZeroHelper.isAgentDefined(agents, defaultAgents);
final ConcurrentMap> ret =
new ConcurrentHashMap<>();
// Fix Boot
// 1. If defined, use default
Ut.itMap(agents, (type, list) -> {
// 2. Defined -> You have defined
Fn.safeSemi(defines.containsKey(type) && defines.get(type), LOGGER,
() -> {
// Use user-defined Agent instead.
final Class> found = Ut.elementFind(list,
(item) -> internals.get(type) != item);
if (null != found) {
ret.put(type, found);
}
}, () -> {
// Use internal defined ( system defaults )
final Class> found = Ut.elementFind(list,
(item) -> internals.get(type) == item);
if (null != found) {
LOGGER.info(Info.AGENT_DEFINED, found, type);
ret.put(type, found);
}
});
});
// 2.Filter
return filterAgents(ret);
}
private static ConcurrentMap> filterAgents(
final ConcurrentMap> agents) {
// Check Rpc Enabled
if (ZeroGrid.getRpcOptions().isEmpty()) {
agents.remove(ServerType.IPC);
} else {
LOGGER.info(Info.RPC_ENABLED);
}
// Check Socket Enabled
if (ZeroGrid.getSockOptions().isEmpty()) {
agents.remove(ServerType.SOCK);
} else {
LOGGER.info(Info.SOCK_ENABLED);
}
return agents;
}
private static ConcurrentMap>> getMergedAgents(
final ServerType category,
final ConcurrentMap> internals
) {
final ConcurrentMap>> agents = ZeroAnno.getAgents();
if (agents.isEmpty()) {
// Inject ServerType by category input.
agents.put(category, new ArrayList<>(internals.values()));
}
return agents;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy