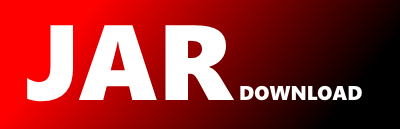
io.vertx.up.secure.provider.JwtSecurer Maven / Gradle / Ivy
package io.vertx.up.secure.provider;
import io.vertx.core.Future;
import io.vertx.core.json.JsonObject;
import io.vertx.ext.auth.User;
import io.vertx.ext.auth.jwt.impl.JWTUser;
import io.vertx.up.exception.WebException;
import io.vertx.up.exception.web._401JwtExecutorException;
import io.vertx.up.exception.web._403ForbiddenException;
import io.vertx.up.log.Annal;
import io.vertx.up.secure.Security;
import io.vertx.up.unity.Ux;
import java.util.function.Supplier;
/**
* JwtAuthProvider will call JwtSecurer here
*/
class JwtSecurer {
private static final Annal LOGGER = Annal.get(JwtSecurer.class);
private transient Security security;
private transient String permissionsClaimKey;
private JwtSecurer() {
}
static JwtSecurer create() {
return new JwtSecurer();
}
void setSecurity(final Security security) {
this.security = security;
}
void setPermissionsClaimKey(final String permissionsClaimKey) {
this.permissionsClaimKey = permissionsClaimKey;
}
/*
* 401
* After 401 workflow, system go through 403 directly.
*/
Future authenticate(final JsonObject authInfo) {
final String token = authInfo.getString("jwt");
/*
* User defined security interface and implement custom code logical
* Call custom code logical here to do 401 validation.
*/
return security.verify(authInfo)
.compose(authenticated -> next(authenticated,
/*
* 401 Passed, continue to do 403 workflow
*/
() -> authorize(authInfo),
/*
* There is no error fired by Future.failedFuture method
* But the validated result is false, it means that there is common
* Authenticate workflow.
*/
() -> new _401JwtExecutorException(getClass(), token)
));
}
/*
* 403
*/
Future authorize(final JsonObject authInfo) {
return security.access(authInfo).compose(authorized -> next(authorized,
/*
* 403 Passed, continue to do business workflow
*/
() -> Ux.toFuture(new JWTUser(authInfo, permissionsClaimKey)),
/*
* There is no error fired by Future.failedFuture method
* But the validated result is false, it means that there is common
* Authorization workflow.
*/
() -> new _403ForbiddenException(getClass())));
}
/*
* Success final steps
*/
private Future next(final boolean checked,
final Supplier> success,
final Supplier error) {
if (checked) {
final Future future = success.get();
final Throwable failure = future.cause();
return null == failure ? future : Future.failedFuture(failure);
} else {
return Future.failedFuture(error.get());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy