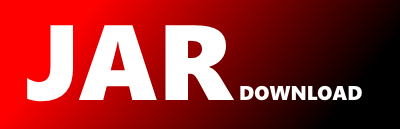
io.vertx.up.uca.micro.invoke.FutureInvoker Maven / Gradle / Ivy
package io.vertx.up.uca.micro.invoke;
import io.vertx.core.Future;
import io.vertx.core.Vertx;
import io.vertx.core.eventbus.Message;
import io.vertx.up.commune.Envelop;
import io.vertx.up.unity.Ux;
import io.vertx.up.util.Ut;
import java.lang.reflect.Method;
/**
* Future method(Envelop)
*/
public class FutureInvoker extends AbstractInvoker {
@Override
public void ensure(final Class> returnType,
final Class> paramCls) {
// Verify
final boolean valid =
Future.class.isAssignableFrom(returnType) && paramCls == Envelop.class;
InvokerUtil.verify(!valid, returnType, paramCls, getClass());
}
@Override
@SuppressWarnings("unchecked")
public void invoke(final Object proxy,
final Method method,
final Message message) {
// Invoke directly
final Envelop envelop = message.body();
// Future
final Class> returnType = method.getReturnType();
// Get T
final Class> tCls = returnType.getComponentType();
getLogger().info(Info.MSG_FUTURE, getClass(), returnType, false);
if (Envelop.class == tCls) {
final Future result = Ut.invoke(proxy, method.getName(), envelop);
result.setHandler(item -> message.reply(item.result()));
} else {
final Future tResult = Ut.invoke(proxy, method.getName(), envelop);
tResult.setHandler(Ux.handler(message));
}
}
@Override
@SuppressWarnings("unchecked")
public void next(final Object proxy,
final Method method,
final Message message,
final Vertx vertx) {
// Invoke directly
final Envelop envelop = message.body();
// Future
final Class> returnType = method.getReturnType();
// Get T
final Class> tCls = returnType.getComponentType();
getLogger().info(Info.MSG_FUTURE, getClass(), returnType, true);
if (Envelop.class == tCls) {
// Execute Future
final Future future = Ut.invoke(proxy, method.getName(), envelop);
/*
future.compose(item -> TunnelClient.create(this.getClass())
.connect(vertx)
.connect(method)
.send(item))
.setHandler(Ux.handler(message)); */
future.compose(nextEnvelop(vertx, method))
.setHandler(Ux.handler(message));
} else {
final Future future = Ut.invoke(proxy, method.getName(), envelop);
/*
future.compose(item -> TunnelClient.create(this.getClass())
.connect(vertx)
.connect(method)
.send(Ux.to(item)))
.compose(item -> Future.succeededFuture(Ux.to(item)))
.setHandler(Ux.handler(message)); */
future.compose(nextEnvelop(vertx, method))
.setHandler(Ux.handler(message));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy