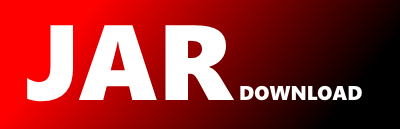
io.vertx.up.uca.web.anima.InfixScatter Maven / Gradle / Ivy
package io.vertx.up.uca.web.anima;
import io.reactivex.Observable;
import io.vertx.core.Vertx;
import io.vertx.tp.error.PluginSpecificationException;
import io.vertx.up.annotations.Plugin;
import io.vertx.up.eon.Plugins;
import io.vertx.up.eon.Values;
import io.vertx.up.fn.Fn;
import io.vertx.up.log.Annal;
import io.vertx.up.runtime.Runner;
import io.vertx.up.runtime.ZeroAmbient;
import io.vertx.up.runtime.ZeroAnno;
import io.vertx.up.uca.yaml.Node;
import io.vertx.up.uca.yaml.ZeroLime;
import io.vertx.up.util.Ut;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.ConcurrentMap;
import java.util.stream.Collectors;
/**
* Infix initialization
*/
public class InfixScatter implements Scatter {
private static final Annal LOGGER = Annal.get(InfixScatter.class);
private static final Node> node =
Ut.singleton(ZeroLime.class);
private static final Set> PLUGINS = ZeroAnno.getTps();
private static final InfixPlugin PLUGIN = InfixPlugin.create(InfixScatter.class);
@Override
@SuppressWarnings("all")
public void connect(final Vertx vertx) {
final ConcurrentMap> wholeInjections = ZeroAmbient.getInjections();
/* Enabled **/
final ConcurrentMap> enabled =
Ut.reduce(node.read().keySet(), wholeInjections);
/* Scan all Infix **/
final ConcurrentMap, Class>> injections =
Ut.reduce(Plugins.INFIX_MAP, enabled);
injections.values().forEach(item -> {
if (null != item && item.isAnnotationPresent(Plugin.class)) {
final Method method = findInit(item);
Fn.outUp(null == method, LOGGER,
PluginSpecificationException.class,
getClass(), item.getName());
Fn.safeJvm(() -> method.invoke(null, vertx), LOGGER);
}
});
/* Scan all extension Infix **/
Observable.fromIterable(wholeInjections.keySet())
.filter(key -> !Plugins.Infix.STANDAND.contains(key))
.map(wholeInjections::get)
.filter(Objects::nonNull)
.filter(item -> item.isAnnotationPresent(Plugin.class))
.subscribe(item -> {
final Method method = findInit(item);
Fn.outUp(null == method, LOGGER,
PluginSpecificationException.class,
getClass(), item.getName());
Fn.safeJvm(() -> method.invoke(null, vertx), LOGGER);
})
.dispose();
/* After infix inject plugins **/
Ut.itSet(PLUGINS, (clazz, index) -> Runner.run(() -> {
/* Instance reference **/
final Object reference = Ut.singleton(clazz);
/* Injects scanner **/
PLUGIN.inject(reference);
}, "injects-plugin-scannner"));
}
/**
* Check whether clazz has the method of name
*/
private Method findInit(final Class> clazz) {
return Fn.getNull(() -> {
final Method[] methods = clazz.getDeclaredMethods();
final List found = Arrays.stream(methods)
.filter(item -> "init".equals(item.getName()) && validMethod(item))
.collect(Collectors.toList());
return Values.ONE == found.size() ? found.get(Values.IDX) : null;
}, clazz);
}
private boolean validMethod(final Method method) {
return (void.class == method.getReturnType() || Void.class == method.getReturnType())
&& Modifier.isStatic(method.getModifiers())
&& Modifier.isPublic(method.getModifiers());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy