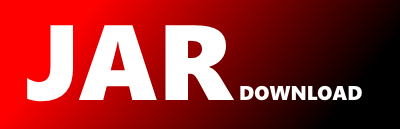
io.vertx.up.unity.JooqReader Maven / Gradle / Ivy
package io.vertx.up.unity;
import io.github.jklingsporn.vertx.jooq.future.VertxDAO;
import io.vertx.core.Future;
import io.vertx.core.json.JsonObject;
import io.vertx.tp.plugin.jooq.JooqInfix;
import org.jooq.Condition;
import org.jooq.DSLContext;
import org.jooq.Operator;
import java.util.Arrays;
import java.util.List;
/**
* Jooq Splitted Reader
* SELECT
* COUNT
* - Search, Check, Find
*/
@SuppressWarnings("all")
public class JooqReader {
private transient final VertxDAO vertxDAO;
private transient JooqAnalyzer analyzer;
private JooqReader(final VertxDAO vertxDAO) {
this.vertxDAO = vertxDAO;
}
static JooqReader create(final VertxDAO vertxDAO) {
return new JooqReader(vertxDAO);
}
JooqReader on(final JooqAnalyzer analyzer) {
this.analyzer = analyzer;
return this;
}
// ============ Fetch One Operation =============
/* Async fetch one operation: SELECT */
Future fetchOneAsync(final String field, final Object value) {
return Async.toFuture(this.vertxDAO.fetchOneAsync(this.analyzer.getColumn(field), value));
}
Future fetchOneAndAsync(final JsonObject filters) {
final Condition condition = JooqCond.transform(filters, Operator.AND, this.analyzer::getColumn);
return Async.toFuture(this.vertxDAO.fetchOneAsync(condition));
}
T fetchOneAnd(final JsonObject filters) {
final Condition condition = JooqCond.transform(filters, Operator.AND, this.analyzer::getColumn);
final DSLContext context = JooqInfix.getDSL();
return this.toResult(context.selectFrom(this.vertxDAO.getTable()).where(condition).fetchOne(this.vertxDAO.mapper()));
}
Future findByIdAsync(final Object id) {
return Async.toFuture(this.vertxDAO.findByIdAsync(id));
}
Future> findAllAsync() {
return Async.toFuture(this.vertxDAO.findAllAsync());
}
/* Sync fetch one operation: SELECT */
T fetchOne(final String field, final Object value) {
return toResult(this.vertxDAO.fetchOne(this.analyzer.getColumn(field), value));
}
T findById(final Object id) {
return toResult(this.vertxDAO.findById(id));
}
List findAll() {
return this.vertxDAO.findAll();
}
// ============ Exist Operation =============
Future existsByIdAsync(final Object id) {
return Async.toFuture(this.vertxDAO.existsByIdAsync(id));
}
Boolean existsById(final Object id) {
return this.vertxDAO.existsById(id);
}
// ============ Fetch List with Condition ===========
Future> fetchAsync(final String field, final Object value) {
return Async.toFuture(this.vertxDAO.fetchAsync(this.analyzer.getColumn(field), Arrays.asList(value)));
}
Future> fetchAsync(final Condition condition) {
return Async.toFuture(this.vertxDAO.fetchAsync(condition));
}
Future> fetchInAsync(final String field, final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy