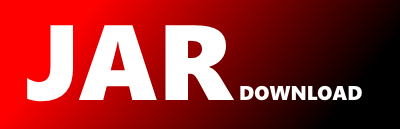
io.vertx.up.unity.UxJooq Maven / Gradle / Ivy
package io.vertx.up.unity;
import io.github.jklingsporn.vertx.jooq.future.VertxDAO;
import io.vertx.core.Future;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import io.vertx.tp.plugin.jooq.JooqInfix;
import io.vertx.up.atom.query.Inquiry;
import io.vertx.up.eon.em.Format;
import io.vertx.up.log.Annal;
import io.vertx.up.fn.Fn;
import org.jooq.Condition;
import org.jooq.Operator;
import java.util.Arrays;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Function;
@SuppressWarnings("all")
public class UxJooq {
private static final Annal LOGGER = Annal.get(UxJooq.class);
private transient final Class> clazz;
/* Analyzer */
private transient final JooqAnalyzer analyzer;
/* Writer */
private transient final JooqWriter writer;
/* Reader */
private transient final JooqReader reader;
private transient Format format = Format.JSON;
UxJooq(final Class clazz, final VertxDAO vertxDAO) {
this.clazz = clazz;
/* Analyzing column for Jooq */
this.analyzer = JooqAnalyzer.create(vertxDAO);
/* Reader connect Analayzer */
this.reader = JooqReader.create(vertxDAO)
.on(this.analyzer);
/* Writer connect Reader */
this.writer = JooqWriter.create(vertxDAO)
.on(this.analyzer).on(this.reader);
}
UxJooq(final Class clazz) {
this(clazz, (VertxDAO) JooqInfix.getDao(clazz));
}
// -------------------- Condition Transform --------------------
public static Condition transform(final JsonObject filters, final Operator operator) {
return JooqCond.transform(filters, operator, null);
}
/**
* Direct analyzing the result to condition
*/
public static Condition transform(final JsonObject filters) {
return JooqCond.transform(filters, null, null);
}
// -------------------- Bind Config --------------------
public UxJooq on(final String pojo) {
this.analyzer.bind(pojo, this.clazz);
return this;
}
public UxJooq on(final Format format) {
this.format = format;
return this;
}
// -------------------- INSERT --------------------
/* Async Only */
public Future insertReturningPrimaryAsync(final T entity, final Consumer consumer) {
return this.writer.insertReturningPrimaryAsync(entity, consumer);
}
/* (Async / Sync) Entity Insert */
public Future insertAsync(final T entity) {
return this.writer.insertAsync(entity);
}
public T insert(final T entity) {
return this.writer.insert(entity);
}
/* (Async / Sync) Collection Insert */
public Future> insertAsync(final List entities) {
return this.writer.insertAsync(entities);
}
public List insert(final List entities) {
return this.writer.insert(entities);
}
// -------------------- UPDATE --------------------
/* Async Only */
public Future upsertReturningPrimaryAsync(final JsonObject filters, final T updated, final Consumer consumer) {
return this.fetchOneAsync(filters).compose(item -> Fn.match(
Fn.fork(() -> this.updateAsync(this.analyzer.copyEntity(item, updated))),
Fn.branch(null == item, () -> this.insertReturningPrimaryAsync(updated, consumer))
));
}
/* (Async / Sync) Entity Update */
public Future updateAsync(final T entity) {
return this.writer.updateAsync(entity);
}
public T update(final T entity) {
return this.writer.update(entity);
}
/* (Async / Sync) Collection Update */
public Future> updateAsync(final List entities) {
return this.writer.updateAsync(entities);
}
public List update(final List entities) {
return this.writer.update(entities);
}
// -------------------- DELETE --------------------
/* (Async / Sync) Delete By ( ID / IDs ) */
public Future deleteByIdAsync(final ID id) {
return this.writer.deleteByIdAsync(id);
}
public Future deleteByIdAsync(final ID... ids) {
return this.writer.deleteByIdAsync(Arrays.asList(ids));
}
public Boolean deleteById(final ID id) {
return this.writer.deleteById(id);
}
public Boolean deleteById(final ID... ids) {
return this.writer.deleteById(Arrays.asList(ids));
}
/* (Async / Sync) Delete Entity */
public Future deleteAsync(final T entity) {
return this.writer.deleteAsync(entity);
}
public T delete(final T entity) {
return this.writer.delete(entity);
}
/* (Async / Sync) Delete by Filters */
public Future deleteAsync(final JsonObject filters) {
return this.writer.deleteAsync(filters, "");
}
public Boolean delete(final JsonObject filters, final String pojo) {
return this.writer.delete(filters, pojo);
}
public Future deleteAsync(final JsonObject filters, final String pojo) {
return this.writer.deleteAsync(filters, pojo);
}
public Boolean delete(final JsonObject filters) {
return this.writer.delete(filters, "");
}
// -------------------- Fetch One/All --------------------
/* (Async / Sync) Fetch One */
public Future fetchOneAsync(final String field, final Object value) {
return this.reader.fetchOneAsync(field, value);
}
public T fetchOne(final String field, final Object value) {
return this.reader.fetchOne(field, value);
}
public Future fetchOneAsync(final JsonObject filters) {
return this.reader.fetchOneAndAsync(filters);
}
public T fetchOne(final JsonObject filters) {
return this.reader.fetchOneAnd(filters);
}
/* (Async / Sync) Find By ID */
public Future findByIdAsync(final Object id) {
return this.reader.findByIdAsync(id);
}
public T findById(final Object id) {
return this.reader.findById(id);
}
/* (Async / Sync) Find All */
public Future> findAllAsync() {
return this.reader.findAllAsync();
}
public List findAll() {
return this.reader.findAll();
}
// -------------------- Save Operation --------------------
/* (Async / Sync) Save Operations */
public Future saveAsync(final Object id, final T updated) {
return this.writer.saveAsync(id, (target) -> this.analyzer.copyEntity(target, updated));
}
public T save(final Object id, final T updated) {
return this.writer.save(id, (target) -> this.analyzer.copyEntity(target, updated));
}
public Future saveAsync(final Object id, final Function copyFun) {
return this.writer.saveAsync(id, copyFun);
}
public T save(final Object id, final Function copyFun) {
return this.writer.save(id, copyFun);
}
// -------------------- Exist Operation --------------------
/* (Async / Sync) Exist By ID Operation */
public Future existsByIdAsync(final Object id) {
return this.reader.existsByIdAsync(id);
}
public Boolean existsById(final Object id) {
return this.reader.existsById(id);
}
/* (Async / Sync) Exist By Filters Operation */
public Future existsOneAsync(final JsonObject filters) {
return this.fetchOneAsync(filters)
.compose(item -> Future.succeededFuture(null != item));
}
public Boolean existsOne(final JsonObject filters) {
final T result = this.fetchOne(filters);
return null != result;
}
// -------------------- Fetch List Operation ---------------
/* (Async / Sync) Fetch to List Operation */
public Future> fetchAsync(final String field, final Object value) {
return this.reader.fetchAsync(field, value);
}
public Future> fetchInAsync(final String field, final Object... value) {
return this.reader.fetchInAsync(field, Arrays.asList(value));
}
public Future> fetchInAsync(final String field, final JsonArray values) {
return this.reader.fetchInAsync(field, values.getList());
}
public List fetch(final String field, final Object value) {
return this.reader.fetch(field, value);
}
public List fetchIn(final String field, final Object... values) {
return this.reader.fetchIn(field, Arrays.asList(values));
}
public List fetchIn(final String field, final JsonArray values) {
return this.reader.fetchIn(field, values.getList());
}
// -------------------- Search Operation -----------------
/* (Async / Sync) Find / Exist / Missing By Filters Operation */
public Future> findAsync(final JsonObject filters) {
return this.analyzer.searchAsync(filters);
}
public Future findExistingAsync(final JsonObject filters) {
return this.findAsync(filters)
.compose(item -> Future.succeededFuture(0 < item.size()));
}
public Future findMissingAsync(final JsonObject filters) {
return this.findAsync(filters)
.compose(item -> Future.succeededFuture(0 == item.size()));
}
public List find(final JsonObject filters) {
return this.analyzer.search(filters);
}
public Boolean findExisting(final JsonObject filters) {
final List list = find(filters);
return 0 < list.size();
}
public Boolean findMissing(final JsonObject filters) {
final List list = find(filters);
return 0 == list.size();
}
// -------------------- Count Operation ------------
/* (Async / Sync) Count Operation */
public Future countAsync(final JsonObject params, final String pojo) {
final Inquiry inquiry = Query.getInquiry(params, pojo);
return this.analyzer.countAsync(inquiry);
}
public Future countAsync(final JsonObject params) {
return countAsync(params, this.analyzer.getPojoFile());
}
public Integer count(final JsonObject params, final String pojo) {
final Inquiry inquiry = Query.getInquiry(params, pojo);
return this.analyzer.count(inquiry);
}
public Integer count(final JsonObject params) {
return count(params, this.analyzer.getPojoFile());
}
// -------------------- Search Operation -----------
/* (Async / Sync) Sort, Projection, Criteria, Pager Search Operations */
public Future searchAsync(final JsonObject params, final String pojo) {
final Inquiry inquiry = Query.getInquiry(params, pojo);
return searchAsync(inquiry, pojo);
}
public Future searchAsync(final JsonObject params) {
return searchAsync(params, this.analyzer.getPojoFile());
}
public Future searchAsync(final Inquiry inquiry, final String pojo) {
return this.analyzer.searchPaginationAsync(inquiry, pojo);
}
public JsonObject search(final JsonObject params, final String pojo) {
final Inquiry inquiry = Query.getInquiry(params, pojo);
return search(inquiry, pojo);
}
public JsonObject search(final JsonObject params) {
return search(params, this.analyzer.getPojoFile());
}
public JsonObject search(final Inquiry inquiry, final String pojo) {
return this.analyzer.searchPagination(inquiry, pojo);
}
// -------------------- Fetch List -------------------
public Future> fetchAndAsync(final JsonObject filters) {
return this.reader.fetchAsync(JooqCond.transform(filters, Operator.AND, this.analyzer::getColumn));
}
public List fetchAnd(final JsonObject filters) {
return this.reader.fetch(JooqCond.transform(filters, Operator.AND, this.analyzer::getColumn));
}
public Future> fetchOrAsync(final JsonObject orFilters) {
return this.reader.fetchAsync(JooqCond.transform(orFilters, Operator.OR, this.analyzer::getColumn));
}
public List fetchOr(final JsonObject orFilters) {
return this.reader.fetch(JooqCond.transform(orFilters, Operator.OR, this.analyzer::getColumn));
}
// -------------------- Upsert ---------
public Future upsertAsync(final JsonObject filters, final T updated) {
return this.fetchOneAsync(filters).compose(item -> Fn.match(
// null != item, updated to existing item.
Fn.fork(() -> this.updateAsync(this.analyzer.copyEntity(item, updated))),
// null == item, insert data
Fn.branch(null == item, () -> this.insertAsync(updated))
));
}
public T upsert(final JsonObject filters, final T updated) {
final T entity = this.fetchOne(filters);
if (null == entity) {
return this.insert(updated);
} else {
return this.update(this.analyzer.copyEntity(entity, updated));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy