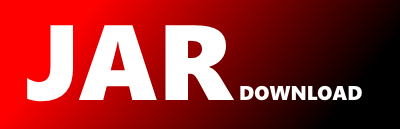
io.vertx.tp.plugin.job.JobClient Maven / Gradle / Ivy
package io.vertx.tp.plugin.job;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.core.AsyncResult;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.Vertx;
import io.vertx.core.json.JsonObject;
import io.vertx.up.atom.worker.Mission;
import io.vertx.up.eon.Constants;
import io.vertx.up.eon.Strings;
import java.util.List;
import java.util.Set;
@SuppressWarnings("all")
public interface JobClient {
/*
* Create local session store bind data
*/
static JobClient createShared(final Vertx vertx, final JsonObject config) {
return new JobClientImpl(vertx, config);
}
static JobClient createShared(final Vertx vertx) {
return new JobClientImpl(vertx, new JsonObject());
}
static void bind(final Long timerId, final String code) {
JobPool.bind(timerId, code);
}
static String code(final String name) {
return Constants.DEFAULT_JOB_NAMESPACE + Strings.DASH + name;
}
// ========================== UxJob mount
/* Start new job */
@Fluent
JobClient startAsync(String code, Handler> handler);
/* Start */
Future startAsync(String code);
/* Stop running job */
@Fluent
JobClient stopAsync(String code, Handler> handler);
/* Stop */
Future stopAsync(String code);
/* Resume a failure job */
@Fluent
JobClient resumeAsync(String code, Handler> handler);
/* Resume */
Future resumeAsync(String timerId);
// ========================== UxJob crud
/* Fetch Single **/
@Fluent
JobClient fetchAsync(String code, Handler> handler);
/* Fetch List */
@Fluent
JobClient fetchAsync(Set code, Handler>> handler);
/* Fetch */
Future fetchAsync(String code);
/* Fetch List */
Future> fetchAsync(Set codes);
Mission fetch(String code);
List fetch(Set codes);
@Fluent
JobClient saveAsync(Set missions, Handler>> handler);
Future> saveAsync(Set missions);
/* Save Mission */
@Fluent
JobClient saveAsync(Mission mission, Handler> handler);
/* Save */
Future saveAsync(Mission mission);
Set save(Set missions);
Mission save(Mission mission);
/* Remove */
@Fluent
JobClient removeAsync(String code, Handler> handler);
/* Delete */
Future removeAsync(String code);
Mission remove(String code);
JsonObject status(String namespace);
Future statusAsync(String namespace);
@Fluent
JobClient statusAsync(String namespace, Handler> handler);
/*
* Because the JobStore started before JobClient initialized,
* In this kind of situation, the class `Pre` could control
* JobPool here
*/
static final class Pre {
public static void save(final Set missions) {
missions.forEach(JobPool::save);
}
public static void save(final Mission mission) {
JobPool.save(mission);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy