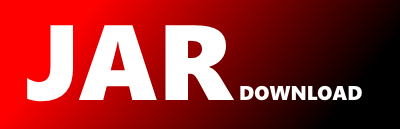
io.vertx.up.runtime.soul.UriStore Maven / Gradle / Ivy
package io.vertx.up.runtime.soul;
import io.vertx.up.annotations.Address;
import io.vertx.up.atom.agent.Event;
import io.vertx.up.atom.worker.Receipt;
import io.vertx.up.runtime.ZeroAnno;
import io.vertx.up.util.Ut;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.stream.Collectors;
/**
* @author Lang
*/
class UriStore {
private static final ConcurrentMap URIS = new ConcurrentHashMap<>();
private static UriStore INSTANCE;
static {
/*
* Initialize data here.
*/
final Set receipts = ZeroAnno.getReceipts();
final ConcurrentMap receiptMap = Ut.elementMap(new ArrayList<>(receipts), Receipt::getAddress);
final Set events = ZeroAnno.getEvents();
/*
* UriMeta building
*/
for (final Event event : events) {
/*
* Uri Meta
*/
final UriMeta uriMeta = new UriMeta();
uriMeta.setMethod(event.getMethod());
uriMeta.setUri(event.getPath());
uriMeta.setDynamic(Boolean.FALSE);
/*
* name / comment
*/
uriMeta.setComment(event.getPath());
uriMeta.setName(event.getPath());
/*
* Get Address
*/
final Method method = event.getAction();
final Annotation annotation = method.getDeclaredAnnotation(Address.class);
final String address = Ut.invoke(annotation, "value");
if (Objects.nonNull(address) && receiptMap.containsKey(address)) {
/*
* Worker Build
*/
final Receipt receipt = receiptMap.get(address);
uriMeta.setAddress(address);
uriMeta.setWorkerMethod(receipt.getMethod());
}
/*
* Cache Key calculation
*/
final String cacheKey = uriMeta.getCacheKey();
if (Ut.notNil(cacheKey)) {
URIS.put(cacheKey, uriMeta);
}
}
}
private UriStore() {
}
/*
* Store URIs when container boot up
* Lazy initialized and extract from Event/Receipt
*/
static UriStore create() {
if (Objects.isNull(INSTANCE)) {
INSTANCE = new UriStore();
}
return INSTANCE;
}
/*
* Add UriMeta into storage
*/
UriStore add(final UriMeta meta) {
final String cacheKey = meta.getCacheKey();
if (Ut.notNil(cacheKey)) {
URIS.put(cacheKey, meta);
}
return this;
}
/*
* Get all Uris, sort by `uri` here
*/
List getAll() {
final List uris = new ArrayList<>(URIS.values());
uris.sort(Comparator.comparing(UriMeta::getUri));
return uris;
}
/*
* Search UriMeta by `keyword`
*/
List search(final String keyword) {
if (Ut.isNil(keyword)) {
return new ArrayList<>();
} else {
final List sortedList = this.getAll();
return sortedList.stream().filter(item -> {
if (item.getUri().contains(keyword)) {
return true;
}
if (Ut.isNil(item.getName())) {
return false;
} else {
return item.getName().contains(keyword);
}
}).collect(Collectors.toList());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy