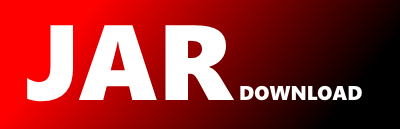
io.vertx.up.uca.invoker.InvokerUtil Maven / Gradle / Ivy
package io.vertx.up.uca.invoker;
import io.vertx.core.json.JsonObject;
import io.vertx.ext.web.Session;
import io.vertx.up.commune.Envelop;
import io.vertx.up.eon.Values;
import io.vertx.up.fn.Fn;
import io.vertx.up.log.Annal;
import io.vertx.up.runtime.ZeroSerializer;
import io.vertx.up.uca.serialization.TypedArgument;
import io.vertx.up.util.Ut;
import io.vertx.zero.exception.AsyncSignatureException;
import io.vertx.zero.exception.WorkerArgumentException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Objects;
import java.util.function.Supplier;
/**
* Tool for invoker do shared works.
*/
@SuppressWarnings("all")
public class InvokerUtil {
private static final Annal LOGGER = Annal.get(InvokerUtil.class);
public static T invoke(final Object proxy, final Method method, final Object... args) {
/*
* Be sure to trust args first calling and then normalized calling
* by `Ut.invoke`, because `Ut.invoke` will parse many parameters here, it means that
* it will analyze the metadata information in runtime, I think it's not needed in
* zero framework now. the method could be invoked with args directly.
*/
try {
return (T) method.invoke(proxy, args);
} catch (final InvocationTargetException | IllegalAccessException ex) {
return Ut.invoke(proxy, method.getName(), args);
}
}
/**
* Whether this method is void
*
* @param method checked method
*
* @return checked result
*/
static boolean isVoid(final Method method) {
final Class> returnType = method.getReturnType();
return void.class == returnType || Void.class == returnType;
}
/**
* TypedArgument verification
* Public for replacing duplicated code
*
* @param method checked method.
* @param target checked class
*/
public static void verifyArgs(final Method method,
final Class> target) {
// 1. Ensure method length
final Class>[] params = method.getParameterTypes();
final Annal logger = Annal.get(target);
// 2. The parameters
Fn.outUp(Values.ZERO == params.length,
logger, WorkerArgumentException.class,
target, method);
}
static void verify(
final boolean condition,
final Class> returnType,
final Class> paramType,
final Class> target) {
final Annal logger = Annal.get(target);
Fn.outUp(condition, logger,
AsyncSignatureException.class, target,
returnType.getName(), paramType.getName());
}
private static Object getValue(final Class> type,
final Envelop envelop,
final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy