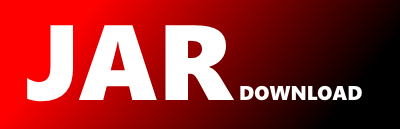
io.vertx.up.uca.jooq.AbstractAction Maven / Gradle / Ivy
package io.vertx.up.uca.jooq;
import io.github.jklingsporn.vertx.jooq.classic.VertxDAO;
import io.vertx.core.json.JsonObject;
import io.vertx.tp.plugin.jooq.JooqDsl;
import io.vertx.tp.plugin.jooq.condition.JooqCond;
import io.vertx.up.log.Annal;
import io.vertx.up.log.Debugger;
import io.vertx.up.util.Ut;
import org.jooq.*;
import org.jooq.impl.DSL;
import java.util.*;
/**
* @author Lang
* Two mode:
*
* 1) Dim1: Sync / Async
* 2) Dim2: Pojo / Bind-Pojo / Non-Pojo
* 3) Dim3: T, List, JsonObject, JsonArray
*
* This class is for basic operation abstraction such as:
*
* INSERT, UPDATE, DELETE, SELECT etc.
*
* The scope is default ( Package Only )
*/
@SuppressWarnings("all")
abstract class AbstractAction {
protected transient final JooqDsl dsl;
protected transient final JqAnalyzer analyzer;
protected AbstractAction(final JqAnalyzer analyzer) {
this.analyzer = analyzer;
this.dsl = analyzer.dsl();
}
protected VertxDAO dao() {
return this.dsl.dao();
}
protected DSLContext context() {
return this.dsl.context();
}
protected void logging(final String pattern, final Object... args) {
final Annal logger = Annal.get(getClass());
if (Debugger.onJooqCondition()) {
logger.info(pattern, args);
}
}
// -------------------------------- Input Method
/*
* Parameter processing here
* Here are two situations:
* 1): No collection -> List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy