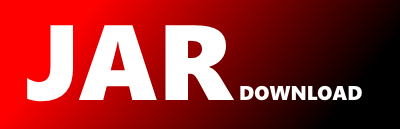
io.vertx.up.uca.jooq.ActionDelete Maven / Gradle / Ivy
package io.vertx.up.uca.jooq;
import io.vertx.core.Future;
import io.vertx.core.json.JsonObject;
import io.vertx.up.eon.Values;
import io.vertx.up.unity.Ux;
import io.vertx.up.util.Ut;
import org.jooq.Condition;
import org.jooq.DeleteConditionStep;
import org.jooq.Query;
import java.util.*;
/**
* @author Lang
*/
@SuppressWarnings("all")
class ActionDelete extends AbstractAction {
private transient final ActionFetch fetch;
ActionDelete(final JqAnalyzer analyzer) {
super(analyzer);
this.fetch = new ActionFetch(analyzer);
}
/* Future */
Future deleteAsync(final T entity) {
final Condition condition = this.analyzer.conditionUk(entity);
return ((Future) this.dao().deleteByCondition(condition)).compose(rows -> {
return Future.succeededFuture(entity);
});
}
/* T */
T delete(final T entity) {
final Condition condition = this.analyzer.conditionUk(entity);
final DeleteConditionStep deleteStep = this.context().deleteFrom(this.analyzer.table())
.where(condition);
final int rows = deleteStep.execute();
this.logging("[ Jq ] delete(T) executed rows: {0}", String.valueOf(rows));
return entity;
}
/* Future> */
Future> deleteAsync(final List entity) {
return this.dsl.executeBlocking(h -> h.complete(this.delete(entity)));
}
/* List */
List delete(final List entity) {
Objects.requireNonNull(entity);
if (entity.isEmpty()) {
return entity;
}
final List batchOps = new ArrayList<>();
entity.stream().map(item -> {
final Condition condition = this.analyzer.conditionUk(item);
return this.context().delete(this.analyzer.table()).where(condition);
}).forEach(batchOps::add);
final int rows[] = this.context().batch(batchOps).execute();
final long updated = Arrays.stream(rows).filter(value -> Values.ONE == value).count();
this.logging("[ Jq ] delete(List) executed rows: {0}/{1}",
String.valueOf(updated), String.valueOf(rows.length));
return entity;
}
/* By Id */
Boolean deleteById(final Collection ids) {
Objects.requireNonNull(ids);
if (ids.isEmpty()) {
return Boolean.TRUE;
}
final List batchOps = new ArrayList<>();
ids.stream().map(id -> {
final Condition condition = this.analyzer.conditionId(id);
return this.context().delete(this.analyzer.table()).where(condition);
}).forEach(batchOps::add);
final int rows[] = this.context().batch(batchOps).execute();
final long updated = Arrays.stream(rows).filter(value -> Values.ONE == value).count();
this.logging("[ Jq ] deleteById(Collection) executed rows: {0}/{1}",
String.valueOf(updated), String.valueOf(rows.length));
return Boolean.TRUE;
}
Future deleteByIdAsync(final Collection ids) {
Objects.requireNonNull(ids);
return ((Future) this.dao().deleteByIds(ids)).compose(rows -> {
this.logging("[ Jq ] deleteByIdAsync(Collection) executed rows: {0}/{1}",
String.valueOf(rows), String.valueOf(ids.size()));
return Future.succeededFuture(Boolean.TRUE);
});
}
Future deleteByAsync(final JsonObject criteria) {
if (Ut.isNil(criteria)) {
/*
* To avoid deleting all records
*/
this.logging("[ Jq ] deleteByAsync(JsonObject) Ignore because the condition is null: {0}",
criteria);
return Ux.future(Boolean.TRUE);
} else {
return fetch.fetchAsync(criteria).compose(list -> {
/*
* Extract primary key for eatch entities
*/
return this.deleteAsync(list).compose(nil -> Future.succeededFuture(Boolean.TRUE));
});
}
}
Boolean deleteBy(final JsonObject criteria) {
if (Ut.isNil(criteria)) {
/*
* To avoid deleting all records
*/
this.logging("[ Jq ] deleteBy(JsonObject) Ignore because the condition is null: {0}",
criteria);
return Boolean.TRUE;
} else {
final List entities = this.fetch.fetch(criteria);
this.delete(entities);
return Boolean.TRUE;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy