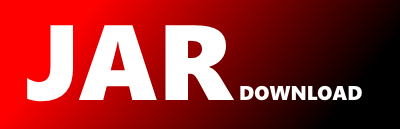
io.vertx.up.uca.jooq.JqReader Maven / Gradle / Ivy
package io.vertx.up.uca.jooq;
import io.vertx.core.Future;
import io.vertx.core.json.JsonObject;
import io.vertx.up.uca.jooq.util.JqFlow;
import java.util.List;
/**
* Jooq Splitted Reader
* SELECT
* COUNT
* - Search, Check, Find
*/
@SuppressWarnings("all")
class JqReader {
private transient ActionSearch search;
private transient ActionFetch fetch;
private transient ActionCheck check;
private JqReader(final JqAnalyzer analyzer) {
/*
* New Structure for more details
*/
this.search = new ActionSearch(analyzer);
this.fetch = new ActionFetch(analyzer);
this.check = new ActionCheck(analyzer);
}
static JqReader create(final JqAnalyzer analyzer) {
return new JqReader(analyzer);
}
// ============ Search Processing =============
Future searchAsync(final JsonObject params, final JqFlow workflow) {
return this.search.searchAsync(params, workflow);
}
JsonObject search(final JsonObject params, final JqFlow workflow) {
return this.search.search(params, workflow);
}
// ============ Fetch Processing ================
Future> fetchAllAsync() {
return this.fetch.fetchAllAsync();
}
List fetchAll() {
return this.fetch.fetchAll();
}
Future> fetchAsync(final String field, final Object value) {
return this.fetch.fetchAsync(field, value);
}
List fetch(final String field, final Object value) {
return this.fetch.fetch(field, value);
}
Future> fetchAsync(final JsonObject criteria) {
return this.fetch.fetchAsync(criteria);
}
List fetch(final JsonObject criteria) {
return this.fetch.fetch(criteria);
}
Future fetchByIdAsync(final ID id) {
return this.fetch.fetchByIdAsync(id);
}
T fetchById(final ID id) {
return this.fetch.fetchById(id);
}
// ============ Fetch One Operation =============
/* Async fetch one operation: SELECT */
Future fetchOneAsync(final String field, final Object value) {
return this.fetch.fetchOneAsync(field, value);
}
T fetchOne(final String field, final Object value) {
return this.fetch.fetchOne(field, value);
}
Future fetchOneAsync(final JsonObject criteria) {
return this.fetch.fetchOneAsync(criteria);
}
T fetchOne(final JsonObject criteria) {
return this.fetch.fetchOne(criteria);
}
// ============ Exist Operation =============
Future existByIdAsync(final Object id) {
return this.check.existByIdAsync(id);
}
Boolean existById(final Object id) {
return this.check.existById(id);
}
Future existAsync(final JsonObject criteria) {
return this.check.existAsync(criteria);
}
Boolean exist(final JsonObject criteria) {
return this.check.exist(criteria);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy