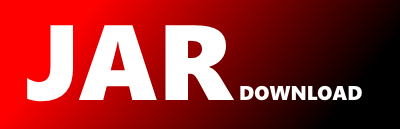
io.vertx.up.uca.jooq.JqWriter Maven / Gradle / Ivy
package io.vertx.up.uca.jooq;
import io.vertx.core.Future;
import io.vertx.core.json.JsonObject;
import java.util.Collection;
import java.util.List;
import java.util.function.BiPredicate;
/**
* Jooq Splitted Writter
* Create
* Update
* Delete
*/
@SuppressWarnings("all")
class JqWriter {
private transient ActionInsert insert;
private transient ActionUpdate update;
private transient ActionUpsert upsert;
private transient ActionDelete delete;
private JqWriter(final JqAnalyzer analyzer) {
/*
* New Structure for more details
*/
this.insert = new ActionInsert(analyzer);
this.update = new ActionUpdate(analyzer);
this.upsert = new ActionUpsert(analyzer);
this.delete = new ActionDelete(analyzer);
}
static JqWriter create(final JqAnalyzer analyzer) {
return new JqWriter(analyzer);
}
// ============ INSERT Operation =============
/* Async insert operation: INSERT */
Future insertAsync(final T entity) {
return this.insert.insertAsync(entity);
}
Future> insertAsync(final List entities) {
return this.insert.insertAsync(entities);
}
T insert(final T entity) {
return this.insert.insert(entity);
}
List insert(final List entities) {
return this.insert.insert(entities);
}
// ============ UPDATE Operation =============
/* Async insert operation: UPDATE */
Future updateAsync(final T entity) {
return this.update.updateAsync(entity);
}
/* Sync insert operation: UPDATE */
T update(final T entity) {
return this.update.update(entity);
}
T update(final ID id, final T updated) {
return this.update.update(id, updated);
}
Future updateAsync(final ID id, final T updated) {
return this.update.updateAsync(id, updated);
}
T update(final JsonObject criteria, final T updated) {
return this.update.update(criteria, updated);
}
Future updateAsync(final JsonObject criteria, final T updated) {
return this.update.updateAsync(criteria, updated);
}
Future> updateAsync(final List entities) {
return this.update.updateAsync(entities);
}
List update(final List entities) {
return this.update.update(entities);
}
// ============ DELETE Operation =============
Future deleteAsync(final T entity) {
return this.delete.deleteAsync(entity);
}
Future> deleteAsync(final List entity) {
return this.delete.deleteAsync(entity);
}
T delete(final T entity) {
return this.delete.delete(entity);
}
List delete(final List entity) {
return this.delete.delete(entity);
}
Future deleteByIdAsync(final Collection ids) {
return this.delete.deleteByIdAsync(ids);
}
Boolean deleteById(final Collection ids) {
return this.delete.deleteById(ids);
}
Boolean deleteBy(final JsonObject criteria) {
return this.delete.deleteBy(criteria);
}
Future deleteByAsync(final JsonObject criteria) {
return this.delete.deleteByAsync(criteria);
}
// ============ UPSERT Operation (Save) =============
public Future upsertAsync(final JsonObject criteria, final T updated) {
return this.upsert.upsertAsync(criteria, updated);
}
public Future upsertAsync(final ID id, final T updated) {
return this.upsert.upsertAsync(id, updated);
}
public T upsert(final JsonObject criteria, final T updated) {
return this.upsert.upsert(criteria, updated);
}
public T upsert(final ID id, final T updated) {
return this.upsert.upsert(id, updated);
}
List upsert(final JsonObject criteria, final List list, final BiPredicate finder) {
return this.upsert.upsert(criteria, list, finder);
}
Future> upsertAsync(final JsonObject criteria, final List list, final BiPredicate finder) {
return this.upsert.upsertAsync(criteria, list, finder);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy