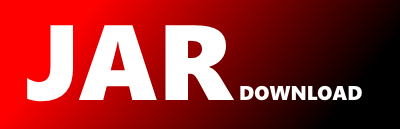
io.vertx.up.unity.Async Maven / Gradle / Ivy
package io.vertx.up.unity;
import io.vertx.core.Future;
import io.vertx.core.Promise;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import io.vertx.up.atom.Refer;
import io.vertx.up.eon.KName;
import io.vertx.up.eon.KValue;
import io.vertx.up.eon.Values;
import io.vertx.up.fn.Fn;
import io.vertx.up.log.Annal;
import io.vertx.up.util.Ut;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.function.Function;
import java.util.function.Supplier;
class Async {
private static final Annal LOGGER = Annal.get(Async.class);
static Future fromAsync(final CompletionStage state) {
final Promise promise = Promise.promise();
state.whenComplete((result, error) -> {
if (Objects.isNull(error)) {
promise.complete(result);
} else {
promise.fail(error);
}
});
return promise.future();
}
static Future future(final T input, final Set>> set) {
final List> futures = new ArrayList<>();
set.stream().map(consumer -> consumer.apply(input)).forEach(futures::add);
Ux.thenCombineT(futures).compose(nil -> {
LOGGER.info("「Job Plugin」 There are `{0}` jobs that are finished successfully!", String.valueOf(set.size()));
return Ux.future(nil);
});
return Ux.future(input);
}
@SuppressWarnings("all")
static Future future(final T input, final List>> queues) {
if (0 == queues.size()) {
/*
* None queue here
*/
return To.future(input);
} else {
Future first = queues.get(Values.IDX).apply(input);
if (Objects.isNull(first)) {
LOGGER.error("The index = 0 future returned null, plugins will be terminal");
return To.future(input);
} else {
if (1 == queues.size()) {
/*
* Get first future
*/
return first;
} else {
/*
* future[0]
* .compose(future[1])
* .compose(future[2])
* .compose(...)
*/
final Refer response = new Refer();
response.add(input);
for (int idx = 1; idx < queues.size(); idx++) {
final int current = idx;
first = first.compose(json -> {
final Future future = queues.get(current).apply(json);
if (Objects.isNull(future)) {
/*
* When null found, skip current
*/
return To.future(json);
} else {
return future
/*
* Replace the result with successed item here
* If success
* -- replace previous response with next
* If failure
* -- returned current json and replace previous response with current
*
* The step stopped
*/
.compose(response::future)
.otherwise(Ux.otherwise(() -> response.add(json).get()));
}
}).otherwise(Ux.otherwise(() -> response.get()));
}
return first;
}
}
}
}
@SuppressWarnings("all")
static Future toJsonFuture(
final String pojo,
final CompletableFuture completableFuture
) {
final Promise future = Promise.promise();
Fn.safeSemi(null == completableFuture, null,
() -> future.complete(new JsonObject()),
() -> completableFuture.thenAcceptAsync((item) -> Fn.safeSemi(
null == item, null,
() -> future.complete(new JsonObject()),
() -> future.complete(To.toJObject(item, pojo))
)).exceptionally((ex) -> {
LOGGER.jvm(ex);
future.fail(ex);
return null;
}));
return future.future();
}
@SuppressWarnings("all")
static Future toArrayFuture(
final String pojo,
final CompletableFuture> completableFuture
) {
final Promise future = Promise.promise();
Fn.safeSemi(null == completableFuture, null,
() -> future.complete(new JsonArray()),
() -> completableFuture.thenAcceptAsync((item) -> Fn.safeSemi(
null == item, null,
() -> future.complete(new JsonArray()),
() -> future.complete(To.toJArray(item, pojo))
)).exceptionally((ex) -> {
LOGGER.jvm(ex);
future.fail(ex);
return null;
}));
return future.future();
}
@SuppressWarnings("all")
static Future toUpsertFuture(final T entity, final String pojo,
final Supplier> supplier,
final Function updateFun) {
return Fn.match(
Fn.fork(() -> Future.succeededFuture(To.toJObject(entity, pojo))
.compose(item -> null == updateFun ?
Future.succeededFuture(item) :
Future.succeededFuture(updateFun.apply(item))
)
),
Fn.branch(null == entity, supplier));
}
static Function> toErrorFuture(final Supplier input) {
return ex -> {
if (Objects.nonNull(ex)) {
ex.printStackTrace();
}
return Future.succeededFuture(input.get());
};
}
static JsonObject bool(final String key, final boolean checked) {
final KValue.Bool response = checked ?
KValue.Bool.SUCCESS : KValue.Bool.FAILURE;
return new JsonObject().put(key, response.name());
}
static Boolean bool(final JsonObject checkedJson) {
final String result = checkedJson.getString(KName.RESULT);
final KValue.Bool resultValue = Ut.toEnum(() -> result, KValue.Bool.class, KValue.Bool.FAILURE);
return KValue.Bool.SUCCESS == resultValue;
}
static JsonObject array(final JsonArray array) {
return new JsonObject().put(KName.DATA, array);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy