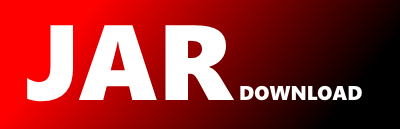
cn.vertxup.erp.domain.tables.EEmployee Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.erp.domain.tables;
import cn.vertxup.erp.domain.Db;
import cn.vertxup.erp.domain.Indexes;
import cn.vertxup.erp.domain.Keys;
import cn.vertxup.erp.domain.tables.records.EEmployeeRecord;
import org.jooq.*;
import org.jooq.impl.DSL;
import org.jooq.impl.SQLDataType;
import org.jooq.impl.TableImpl;
import java.time.LocalDateTime;
import java.util.Arrays;
import java.util.List;
/**
* This class is generated by jOOQ.
*/
@SuppressWarnings({"all", "unchecked", "rawtypes"})
public class EEmployee extends TableImpl {
/**
* The reference instance of DB_ETERNAL.E_EMPLOYEE
*/
public static final EEmployee E_EMPLOYEE = new EEmployee();
private static final long serialVersionUID = 1L;
/**
* The column DB_ETERNAL.E_EMPLOYEE.KEY
. 「key」- 员工主键
*/
public final TableField KEY = createField(DSL.name("KEY"), SQLDataType.VARCHAR(36).nullable(false), this, "「key」- 员工主键");
/**
* The column DB_ETERNAL.E_EMPLOYEE.COMPANY_ID
. 「companyId」-
* 所属公司
*/
public final TableField COMPANY_ID = createField(DSL.name("COMPANY_ID"), SQLDataType.VARCHAR(36), this, "「companyId」- 所属公司");
/**
* The column DB_ETERNAL.E_EMPLOYEE.DEPT_ID
. 「deptId」- 所属部门
*/
public final TableField DEPT_ID = createField(DSL.name("DEPT_ID"), SQLDataType.VARCHAR(36), this, "「deptId」- 所属部门");
/**
* The column DB_ETERNAL.E_EMPLOYEE.TEAM_ID
. 「teamId」- 所属组
*/
public final TableField TEAM_ID = createField(DSL.name("TEAM_ID"), SQLDataType.VARCHAR(36), this, "「teamId」- 所属组");
/**
* The column DB_ETERNAL.E_EMPLOYEE.IDENTITY_ID
. 「identityId」-
* 关联档案
*/
public final TableField IDENTITY_ID = createField(DSL.name("IDENTITY_ID"), SQLDataType.VARCHAR(36), this, "「identityId」- 关联档案");
/**
* The column DB_ETERNAL.E_EMPLOYEE.VICE_NAME
. 「viceName」- 员工姓名
*/
public final TableField VICE_NAME = createField(DSL.name("VICE_NAME"), SQLDataType.VARCHAR(255), this, "「viceName」- 员工姓名");
/**
* The column DB_ETERNAL.E_EMPLOYEE.VICE_EMAIL
. 「viceEmail」-
* 员工邮箱
*/
public final TableField VICE_EMAIL = createField(DSL.name("VICE_EMAIL"), SQLDataType.VARCHAR(255), this, "「viceEmail」- 员工邮箱");
/**
* The column DB_ETERNAL.E_EMPLOYEE.VICE_MOBILE
. 「viceMobile」-
* 员工手机
*/
public final TableField VICE_MOBILE = createField(DSL.name("VICE_MOBILE"), SQLDataType.VARCHAR(255), this, "「viceMobile」- 员工手机");
/**
* The column DB_ETERNAL.E_EMPLOYEE.WORK_NUMBER
. 「workNumber」-
* 工号
*/
public final TableField WORK_NUMBER = createField(DSL.name("WORK_NUMBER"), SQLDataType.VARCHAR(255), this, "「workNumber」- 工号");
/**
* The column DB_ETERNAL.E_EMPLOYEE.WORK_TITLE
. 「workTitle」- 头衔
*/
public final TableField WORK_TITLE = createField(DSL.name("WORK_TITLE"), SQLDataType.VARCHAR(255), this, "「workTitle」- 头衔");
/**
* The column DB_ETERNAL.E_EMPLOYEE.WORK_LOCATION
.
* 「workLocation」- 办公地点
*/
public final TableField WORK_LOCATION = createField(DSL.name("WORK_LOCATION"), SQLDataType.CLOB, this, "「workLocation」- 办公地点");
/**
* The column DB_ETERNAL.E_EMPLOYEE.WORK_PHONE
. 「workPhone」-
* 办公电话
*/
public final TableField WORK_PHONE = createField(DSL.name("WORK_PHONE"), SQLDataType.VARCHAR(20), this, "「workPhone」- 办公电话");
/**
* The column DB_ETERNAL.E_EMPLOYEE.WORK_EXTENSION
.
* 「workExtension」- 分机号
*/
public final TableField WORK_EXTENSION = createField(DSL.name("WORK_EXTENSION"), SQLDataType.VARCHAR(20), this, "「workExtension」- 分机号");
/**
* The column DB_ETERNAL.E_EMPLOYEE.TYPE
. 「type」- 员工分类
*/
public final TableField TYPE = createField(DSL.name("TYPE"), SQLDataType.VARCHAR(36), this, "「type」- 员工分类");
/**
* The column DB_ETERNAL.E_EMPLOYEE.METADATA
. 「metadata」- 附加配置
*/
public final TableField METADATA = createField(DSL.name("METADATA"), SQLDataType.CLOB, this, "「metadata」- 附加配置");
/**
* The column DB_ETERNAL.E_EMPLOYEE.ACTIVE
. 「active」- 是否启用
*/
public final TableField ACTIVE = createField(DSL.name("ACTIVE"), SQLDataType.BIT, this, "「active」- 是否启用");
/**
* The column DB_ETERNAL.E_EMPLOYEE.SIGMA
. 「sigma」-
* 统一标识(公司所属应用)
*/
public final TableField SIGMA = createField(DSL.name("SIGMA"), SQLDataType.VARCHAR(32), this, "「sigma」- 统一标识(公司所属应用)");
/**
* The column DB_ETERNAL.E_EMPLOYEE.LANGUAGE
. 「language」- 使用的语言
*/
public final TableField LANGUAGE = createField(DSL.name("LANGUAGE"), SQLDataType.VARCHAR(8), this, "「language」- 使用的语言");
/**
* The column DB_ETERNAL.E_EMPLOYEE.CREATED_AT
. 「createdAt」-
* 创建时间
*/
public final TableField CREATED_AT = createField(DSL.name("CREATED_AT"), SQLDataType.LOCALDATETIME(0), this, "「createdAt」- 创建时间");
/**
* The column DB_ETERNAL.E_EMPLOYEE.CREATED_BY
. 「createdBy」-
* 创建人
*/
public final TableField CREATED_BY = createField(DSL.name("CREATED_BY"), SQLDataType.VARCHAR(36), this, "「createdBy」- 创建人");
/**
* The column DB_ETERNAL.E_EMPLOYEE.UPDATED_AT
. 「updatedAt」-
* 更新时间
*/
public final TableField UPDATED_AT = createField(DSL.name("UPDATED_AT"), SQLDataType.LOCALDATETIME(0), this, "「updatedAt」- 更新时间");
/**
* The column DB_ETERNAL.E_EMPLOYEE.UPDATED_BY
. 「updatedBy」-
* 更新人
*/
public final TableField UPDATED_BY = createField(DSL.name("UPDATED_BY"), SQLDataType.VARCHAR(36), this, "「updatedBy」- 更新人");
private EEmployee(Name alias, Table aliased) {
this(alias, aliased, null);
}
private EEmployee(Name alias, Table aliased, Field>[] parameters) {
super(alias, null, aliased, parameters, DSL.comment(""), TableOptions.table());
}
/**
* Create an aliased DB_ETERNAL.E_EMPLOYEE
table reference
*/
public EEmployee(String alias) {
this(DSL.name(alias), E_EMPLOYEE);
}
/**
* Create an aliased DB_ETERNAL.E_EMPLOYEE
table reference
*/
public EEmployee(Name alias) {
this(alias, E_EMPLOYEE);
}
/**
* Create a DB_ETERNAL.E_EMPLOYEE
table reference
*/
public EEmployee() {
this(DSL.name("E_EMPLOYEE"), null);
}
public EEmployee(Table child, ForeignKey key) {
super(child, key, E_EMPLOYEE);
}
/**
* The class holding records for this type
*/
@Override
public Class getRecordType() {
return EEmployeeRecord.class;
}
@Override
public Schema getSchema() {
return aliased() ? null : Db.DB_ETERNAL;
}
@Override
public List getIndexes() {
return Arrays.asList(Indexes.E_EMPLOYEE_IDX_E_EMPLOYEE_SIGMA, Indexes.E_EMPLOYEE_IDX_E_EMPLOYEE_SIGMA_ACTIVE, Indexes.E_EMPLOYEE_IDX_E_EMPLOYEE_WORK_NUMBER);
}
@Override
public UniqueKey getPrimaryKey() {
return Keys.KEY_E_EMPLOYEE_PRIMARY;
}
@Override
public List> getUniqueKeys() {
return Arrays.asList(Keys.KEY_E_EMPLOYEE_WORK_NUMBER);
}
@Override
public EEmployee as(String alias) {
return new EEmployee(DSL.name(alias), this);
}
@Override
public EEmployee as(Name alias) {
return new EEmployee(alias, this);
}
/**
* Rename this table
*/
@Override
public EEmployee rename(String name) {
return new EEmployee(DSL.name(name), null);
}
/**
* Rename this table
*/
@Override
public EEmployee rename(Name name) {
return new EEmployee(name, null);
}
// -------------------------------------------------------------------------
// Row22 type methods
// -------------------------------------------------------------------------
@Override
public Row22 fieldsRow() {
return (Row22) super.fieldsRow();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy