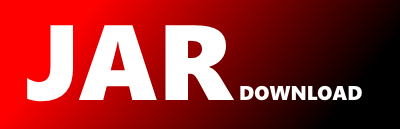
cn.vertxup.erp.domain.tables.pojos.EContract Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.erp.domain.tables.pojos;
import cn.vertxup.erp.domain.tables.interfaces.IEContract;
import io.github.jklingsporn.vertx.jooq.shared.internal.VertxPojo;
import java.math.BigDecimal;
import java.time.LocalDateTime;
import static io.github.jklingsporn.vertx.jooq.shared.internal.VertxPojo.*;
/**
* This class is generated by jOOQ.
*/
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class EContract implements VertxPojo, IEContract {
private static final long serialVersionUID = 1L;
private String key;
private String name;
private String code;
private String title;
private String fileKey;
private BigDecimal amount;
private String companyId;
private String customerId;
private LocalDateTime expiredAt;
private LocalDateTime signedAt;
private LocalDateTime runAt;
private LocalDateTime runUpAt;
private String aName;
private String aPhone;
private String aAddress;
private String bName;
private String bPhone;
private String bAddress;
private String type;
private String metadata;
private Boolean active;
private String sigma;
private String language;
private LocalDateTime createdAt;
private String createdBy;
private LocalDateTime updatedAt;
private String updatedBy;
public EContract() {}
public EContract(IEContract value) {
this.key = value.getKey();
this.name = value.getName();
this.code = value.getCode();
this.title = value.getTitle();
this.fileKey = value.getFileKey();
this.amount = value.getAmount();
this.companyId = value.getCompanyId();
this.customerId = value.getCustomerId();
this.expiredAt = value.getExpiredAt();
this.signedAt = value.getSignedAt();
this.runAt = value.getRunAt();
this.runUpAt = value.getRunUpAt();
this.aName = value.getAName();
this.aPhone = value.getAPhone();
this.aAddress = value.getAAddress();
this.bName = value.getBName();
this.bPhone = value.getBPhone();
this.bAddress = value.getBAddress();
this.type = value.getType();
this.metadata = value.getMetadata();
this.active = value.getActive();
this.sigma = value.getSigma();
this.language = value.getLanguage();
this.createdAt = value.getCreatedAt();
this.createdBy = value.getCreatedBy();
this.updatedAt = value.getUpdatedAt();
this.updatedBy = value.getUpdatedBy();
}
public EContract(
String key,
String name,
String code,
String title,
String fileKey,
BigDecimal amount,
String companyId,
String customerId,
LocalDateTime expiredAt,
LocalDateTime signedAt,
LocalDateTime runAt,
LocalDateTime runUpAt,
String aName,
String aPhone,
String aAddress,
String bName,
String bPhone,
String bAddress,
String type,
String metadata,
Boolean active,
String sigma,
String language,
LocalDateTime createdAt,
String createdBy,
LocalDateTime updatedAt,
String updatedBy
) {
this.key = key;
this.name = name;
this.code = code;
this.title = title;
this.fileKey = fileKey;
this.amount = amount;
this.companyId = companyId;
this.customerId = customerId;
this.expiredAt = expiredAt;
this.signedAt = signedAt;
this.runAt = runAt;
this.runUpAt = runUpAt;
this.aName = aName;
this.aPhone = aPhone;
this.aAddress = aAddress;
this.bName = bName;
this.bPhone = bPhone;
this.bAddress = bAddress;
this.type = type;
this.metadata = metadata;
this.active = active;
this.sigma = sigma;
this.language = language;
this.createdAt = createdAt;
this.createdBy = createdBy;
this.updatedAt = updatedAt;
this.updatedBy = updatedBy;
}
public EContract(io.vertx.core.json.JsonObject json) {
this();
fromJson(json);
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.KEY
. 「key」- 合同主键
*/
@Override
public String getKey() {
return this.key;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.KEY
. 「key」- 合同主键
*/
@Override
public EContract setKey(String key) {
this.key = key;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.NAME
. 「name」- 合同名称
*/
@Override
public String getName() {
return this.name;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.NAME
. 「name」- 合同名称
*/
@Override
public EContract setName(String name) {
this.name = name;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.CODE
. 「code」- 合同编号
*/
@Override
public String getCode() {
return this.code;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.CODE
. 「code」- 合同编号
*/
@Override
public EContract setCode(String code) {
this.code = code;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.TITLE
. 「title」- 合同标题
*/
@Override
public String getTitle() {
return this.title;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.TITLE
. 「title」- 合同标题
*/
@Override
public EContract setTitle(String title) {
this.title = title;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.FILE_KEY
. 「fileKey」-
* 合同附件Key
*/
@Override
public String getFileKey() {
return this.fileKey;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.FILE_KEY
. 「fileKey」-
* 合同附件Key
*/
@Override
public EContract setFileKey(String fileKey) {
this.fileKey = fileKey;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.AMOUNT
. 「amount」- 合同金额
*/
@Override
public BigDecimal getAmount() {
return this.amount;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.AMOUNT
. 「amount」- 合同金额
*/
@Override
public EContract setAmount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.COMPANY_ID
. 「companyId」-
* 合同甲方
*/
@Override
public String getCompanyId() {
return this.companyId;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.COMPANY_ID
. 「companyId」-
* 合同甲方
*/
@Override
public EContract setCompanyId(String companyId) {
this.companyId = companyId;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.CUSTOMER_ID
. 「customerId」-
* 合同乙方
*/
@Override
public String getCustomerId() {
return this.customerId;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.CUSTOMER_ID
. 「customerId」-
* 合同乙方
*/
@Override
public EContract setCustomerId(String customerId) {
this.customerId = customerId;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.EXPIRED_AT
. 「expiredAt」-
* 过期时间
*/
@Override
public LocalDateTime getExpiredAt() {
return this.expiredAt;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.EXPIRED_AT
. 「expiredAt」-
* 过期时间
*/
@Override
public EContract setExpiredAt(LocalDateTime expiredAt) {
this.expiredAt = expiredAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.SIGNED_AT
. 「signedAt」- 签订时间
*/
@Override
public LocalDateTime getSignedAt() {
return this.signedAt;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.SIGNED_AT
. 「signedAt」- 签订时间
*/
@Override
public EContract setSignedAt(LocalDateTime signedAt) {
this.signedAt = signedAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.RUN_AT
. 「runAt」- 生效时间
*/
@Override
public LocalDateTime getRunAt() {
return this.runAt;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.RUN_AT
. 「runAt」- 生效时间
*/
@Override
public EContract setRunAt(LocalDateTime runAt) {
this.runAt = runAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.RUN_UP_AT
. 「runUpAt」-
* 挂账到期时间
*/
@Override
public LocalDateTime getRunUpAt() {
return this.runUpAt;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.RUN_UP_AT
. 「runUpAt」-
* 挂账到期时间
*/
@Override
public EContract setRunUpAt(LocalDateTime runUpAt) {
this.runUpAt = runUpAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.A_NAME
. 「aName」- 甲方签订人
*/
@Override
public String getAName() {
return this.aName;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.A_NAME
. 「aName」- 甲方签订人
*/
@Override
public EContract setAName(String aName) {
this.aName = aName;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.A_PHONE
. 「aPhone」- 甲方签订人姓名
*/
@Override
public String getAPhone() {
return this.aPhone;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.A_PHONE
. 「aPhone」- 甲方签订人姓名
*/
@Override
public EContract setAPhone(String aPhone) {
this.aPhone = aPhone;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.A_ADDRESS
. 「aAddress」-
* 甲方联系地址
*/
@Override
public String getAAddress() {
return this.aAddress;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.A_ADDRESS
. 「aAddress」-
* 甲方联系地址
*/
@Override
public EContract setAAddress(String aAddress) {
this.aAddress = aAddress;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.B_NAME
. 「bName」- 乙方签订人
*/
@Override
public String getBName() {
return this.bName;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.B_NAME
. 「bName」- 乙方签订人
*/
@Override
public EContract setBName(String bName) {
this.bName = bName;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.B_PHONE
. 「bPhone」- 乙方签订人姓名
*/
@Override
public String getBPhone() {
return this.bPhone;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.B_PHONE
. 「bPhone」- 乙方签订人姓名
*/
@Override
public EContract setBPhone(String bPhone) {
this.bPhone = bPhone;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.B_ADDRESS
. 「bAddress」-
* 乙方联系地址
*/
@Override
public String getBAddress() {
return this.bAddress;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.B_ADDRESS
. 「bAddress」-
* 乙方联系地址
*/
@Override
public EContract setBAddress(String bAddress) {
this.bAddress = bAddress;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.TYPE
. 「type」- 合同分类
*/
@Override
public String getType() {
return this.type;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.TYPE
. 「type」- 合同分类
*/
@Override
public EContract setType(String type) {
this.type = type;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.METADATA
. 「metadata」- 附加配置
*/
@Override
public String getMetadata() {
return this.metadata;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.METADATA
. 「metadata」- 附加配置
*/
@Override
public EContract setMetadata(String metadata) {
this.metadata = metadata;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.ACTIVE
. 「active」- 是否启用
*/
@Override
public Boolean getActive() {
return this.active;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.ACTIVE
. 「active」- 是否启用
*/
@Override
public EContract setActive(Boolean active) {
this.active = active;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.SIGMA
. 「sigma」-
* 统一标识(公司所属应用)
*/
@Override
public String getSigma() {
return this.sigma;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.SIGMA
. 「sigma」-
* 统一标识(公司所属应用)
*/
@Override
public EContract setSigma(String sigma) {
this.sigma = sigma;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.LANGUAGE
. 「language」- 使用的语言
*/
@Override
public String getLanguage() {
return this.language;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.LANGUAGE
. 「language」- 使用的语言
*/
@Override
public EContract setLanguage(String language) {
this.language = language;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.CREATED_AT
. 「createdAt」-
* 创建时间
*/
@Override
public LocalDateTime getCreatedAt() {
return this.createdAt;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.CREATED_AT
. 「createdAt」-
* 创建时间
*/
@Override
public EContract setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.CREATED_BY
. 「createdBy」-
* 创建人
*/
@Override
public String getCreatedBy() {
return this.createdBy;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.CREATED_BY
. 「createdBy」-
* 创建人
*/
@Override
public EContract setCreatedBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.UPDATED_AT
. 「updatedAt」-
* 更新时间
*/
@Override
public LocalDateTime getUpdatedAt() {
return this.updatedAt;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.UPDATED_AT
. 「updatedAt」-
* 更新时间
*/
@Override
public EContract setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CONTRACT.UPDATED_BY
. 「updatedBy」-
* 更新人
*/
@Override
public String getUpdatedBy() {
return this.updatedBy;
}
/**
* Setter for DB_ETERNAL.E_CONTRACT.UPDATED_BY
. 「updatedBy」-
* 更新人
*/
@Override
public EContract setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("EContract (");
sb.append(key);
sb.append(", ").append(name);
sb.append(", ").append(code);
sb.append(", ").append(title);
sb.append(", ").append(fileKey);
sb.append(", ").append(amount);
sb.append(", ").append(companyId);
sb.append(", ").append(customerId);
sb.append(", ").append(expiredAt);
sb.append(", ").append(signedAt);
sb.append(", ").append(runAt);
sb.append(", ").append(runUpAt);
sb.append(", ").append(aName);
sb.append(", ").append(aPhone);
sb.append(", ").append(aAddress);
sb.append(", ").append(bName);
sb.append(", ").append(bPhone);
sb.append(", ").append(bAddress);
sb.append(", ").append(type);
sb.append(", ").append(metadata);
sb.append(", ").append(active);
sb.append(", ").append(sigma);
sb.append(", ").append(language);
sb.append(", ").append(createdAt);
sb.append(", ").append(createdBy);
sb.append(", ").append(updatedAt);
sb.append(", ").append(updatedBy);
sb.append(")");
return sb.toString();
}
// -------------------------------------------------------------------------
// FROM and INTO
// -------------------------------------------------------------------------
@Override
public void from(IEContract from) {
setKey(from.getKey());
setName(from.getName());
setCode(from.getCode());
setTitle(from.getTitle());
setFileKey(from.getFileKey());
setAmount(from.getAmount());
setCompanyId(from.getCompanyId());
setCustomerId(from.getCustomerId());
setExpiredAt(from.getExpiredAt());
setSignedAt(from.getSignedAt());
setRunAt(from.getRunAt());
setRunUpAt(from.getRunUpAt());
setAName(from.getAName());
setAPhone(from.getAPhone());
setAAddress(from.getAAddress());
setBName(from.getBName());
setBPhone(from.getBPhone());
setBAddress(from.getBAddress());
setType(from.getType());
setMetadata(from.getMetadata());
setActive(from.getActive());
setSigma(from.getSigma());
setLanguage(from.getLanguage());
setCreatedAt(from.getCreatedAt());
setCreatedBy(from.getCreatedBy());
setUpdatedAt(from.getUpdatedAt());
setUpdatedBy(from.getUpdatedBy());
}
@Override
public E into(E into) {
into.from(this);
return into;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy