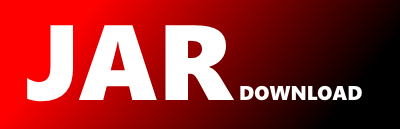
cn.vertxup.erp.domain.tables.pojos.ECustomer Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.erp.domain.tables.pojos;
import cn.vertxup.erp.domain.tables.interfaces.IECustomer;
import io.github.jklingsporn.vertx.jooq.shared.internal.VertxPojo;
import java.time.LocalDateTime;
import static io.github.jklingsporn.vertx.jooq.shared.internal.VertxPojo.*;
/**
* This class is generated by jOOQ.
*/
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class ECustomer implements VertxPojo, IECustomer {
private static final long serialVersionUID = 1L;
private String key;
private String comment;
private String name;
private String title;
private String code;
private String taxCode;
private String taxTitle;
private String contactName;
private String contactPhone;
private String contactEmail;
private String contactOnline;
private String email;
private String fax;
private String homepage;
private String logo;
private String phone;
private String address;
private Boolean runUp;
private String type;
private String metadata;
private Boolean active;
private String sigma;
private String language;
private LocalDateTime createdAt;
private String createdBy;
private LocalDateTime updatedAt;
private String updatedBy;
public ECustomer() {}
public ECustomer(IECustomer value) {
this.key = value.getKey();
this.comment = value.getComment();
this.name = value.getName();
this.title = value.getTitle();
this.code = value.getCode();
this.taxCode = value.getTaxCode();
this.taxTitle = value.getTaxTitle();
this.contactName = value.getContactName();
this.contactPhone = value.getContactPhone();
this.contactEmail = value.getContactEmail();
this.contactOnline = value.getContactOnline();
this.email = value.getEmail();
this.fax = value.getFax();
this.homepage = value.getHomepage();
this.logo = value.getLogo();
this.phone = value.getPhone();
this.address = value.getAddress();
this.runUp = value.getRunUp();
this.type = value.getType();
this.metadata = value.getMetadata();
this.active = value.getActive();
this.sigma = value.getSigma();
this.language = value.getLanguage();
this.createdAt = value.getCreatedAt();
this.createdBy = value.getCreatedBy();
this.updatedAt = value.getUpdatedAt();
this.updatedBy = value.getUpdatedBy();
}
public ECustomer(
String key,
String comment,
String name,
String title,
String code,
String taxCode,
String taxTitle,
String contactName,
String contactPhone,
String contactEmail,
String contactOnline,
String email,
String fax,
String homepage,
String logo,
String phone,
String address,
Boolean runUp,
String type,
String metadata,
Boolean active,
String sigma,
String language,
LocalDateTime createdAt,
String createdBy,
LocalDateTime updatedAt,
String updatedBy
) {
this.key = key;
this.comment = comment;
this.name = name;
this.title = title;
this.code = code;
this.taxCode = taxCode;
this.taxTitle = taxTitle;
this.contactName = contactName;
this.contactPhone = contactPhone;
this.contactEmail = contactEmail;
this.contactOnline = contactOnline;
this.email = email;
this.fax = fax;
this.homepage = homepage;
this.logo = logo;
this.phone = phone;
this.address = address;
this.runUp = runUp;
this.type = type;
this.metadata = metadata;
this.active = active;
this.sigma = sigma;
this.language = language;
this.createdAt = createdAt;
this.createdBy = createdBy;
this.updatedAt = updatedAt;
this.updatedBy = updatedBy;
}
public ECustomer(io.vertx.core.json.JsonObject json) {
this();
fromJson(json);
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.KEY
. 「key」- 客户ID
*/
@Override
public String getKey() {
return this.key;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.KEY
. 「key」- 客户ID
*/
@Override
public ECustomer setKey(String key) {
this.key = key;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.COMMENT
. 「comment」- 客户备注
*/
@Override
public String getComment() {
return this.comment;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.COMMENT
. 「comment」- 客户备注
*/
@Override
public ECustomer setComment(String comment) {
this.comment = comment;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.NAME
. 「name」- 客户名称
*/
@Override
public String getName() {
return this.name;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.NAME
. 「name」- 客户名称
*/
@Override
public ECustomer setName(String name) {
this.name = name;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.TITLE
. 「title」- 客户显示标题
*/
@Override
public String getTitle() {
return this.title;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.TITLE
. 「title」- 客户显示标题
*/
@Override
public ECustomer setTitle(String title) {
this.title = title;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CODE
. 「code」- 客户编号
*/
@Override
public String getCode() {
return this.code;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CODE
. 「code」- 客户编号
*/
@Override
public ECustomer setCode(String code) {
this.code = code;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.TAX_CODE
. 「taxCode」- 税号
*/
@Override
public String getTaxCode() {
return this.taxCode;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.TAX_CODE
. 「taxCode」- 税号
*/
@Override
public ECustomer setTaxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.TAX_TITLE
. 「taxTitle」- 开票抬头
*/
@Override
public String getTaxTitle() {
return this.taxTitle;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.TAX_TITLE
. 「taxTitle」- 开票抬头
*/
@Override
public ECustomer setTaxTitle(String taxTitle) {
this.taxTitle = taxTitle;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CONTACT_NAME
.
* 「contactName」- 联系人姓名
*/
@Override
public String getContactName() {
return this.contactName;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CONTACT_NAME
.
* 「contactName」- 联系人姓名
*/
@Override
public ECustomer setContactName(String contactName) {
this.contactName = contactName;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CONTACT_PHONE
.
* 「contactPhone」- 联系人电话
*/
@Override
public String getContactPhone() {
return this.contactPhone;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CONTACT_PHONE
.
* 「contactPhone」- 联系人电话
*/
@Override
public ECustomer setContactPhone(String contactPhone) {
this.contactPhone = contactPhone;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CONTACT_EMAIL
.
* 「contactEmail」- 联系人Email
*/
@Override
public String getContactEmail() {
return this.contactEmail;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CONTACT_EMAIL
.
* 「contactEmail」- 联系人Email
*/
@Override
public ECustomer setContactEmail(String contactEmail) {
this.contactEmail = contactEmail;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CONTACT_ONLINE
.
* 「contactOnline」- 在线联系方式
*/
@Override
public String getContactOnline() {
return this.contactOnline;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CONTACT_ONLINE
.
* 「contactOnline」- 在线联系方式
*/
@Override
public ECustomer setContactOnline(String contactOnline) {
this.contactOnline = contactOnline;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.EMAIL
. 「email」- 企业邮箱
*/
@Override
public String getEmail() {
return this.email;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.EMAIL
. 「email」- 企业邮箱
*/
@Override
public ECustomer setEmail(String email) {
this.email = email;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.FAX
. 「fax」- 传真号
*/
@Override
public String getFax() {
return this.fax;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.FAX
. 「fax」- 传真号
*/
@Override
public ECustomer setFax(String fax) {
this.fax = fax;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.HOMEPAGE
. 「homepage」- 客户主页
*/
@Override
public String getHomepage() {
return this.homepage;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.HOMEPAGE
. 「homepage」- 客户主页
*/
@Override
public ECustomer setHomepage(String homepage) {
this.homepage = homepage;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.LOGO
. 「logo」- 附件对应的
* attachment Key
*/
@Override
public String getLogo() {
return this.logo;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.LOGO
. 「logo」- 附件对应的
* attachment Key
*/
@Override
public ECustomer setLogo(String logo) {
this.logo = logo;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.PHONE
. 「phone」- 客户座机
*/
@Override
public String getPhone() {
return this.phone;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.PHONE
. 「phone」- 客户座机
*/
@Override
public ECustomer setPhone(String phone) {
this.phone = phone;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.ADDRESS
. 「address」- 客户地址
*/
@Override
public String getAddress() {
return this.address;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.ADDRESS
. 「address」- 客户地址
*/
@Override
public ECustomer setAddress(String address) {
this.address = address;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.RUN_UP
. 「runUp」- 挂账属性
*/
@Override
public Boolean getRunUp() {
return this.runUp;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.RUN_UP
. 「runUp」- 挂账属性
*/
@Override
public ECustomer setRunUp(Boolean runUp) {
this.runUp = runUp;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.TYPE
. 「type」-
* 客户分类(不同类型代表不同客户)
*/
@Override
public String getType() {
return this.type;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.TYPE
. 「type」-
* 客户分类(不同类型代表不同客户)
*/
@Override
public ECustomer setType(String type) {
this.type = type;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.METADATA
. 「metadata」- 附加配置
*/
@Override
public String getMetadata() {
return this.metadata;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.METADATA
. 「metadata」- 附加配置
*/
@Override
public ECustomer setMetadata(String metadata) {
this.metadata = metadata;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.ACTIVE
. 「active」- 是否启用
*/
@Override
public Boolean getActive() {
return this.active;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.ACTIVE
. 「active」- 是否启用
*/
@Override
public ECustomer setActive(Boolean active) {
this.active = active;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.SIGMA
. 「sigma」-
* 统一标识(客户所属应用)
*/
@Override
public String getSigma() {
return this.sigma;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.SIGMA
. 「sigma」-
* 统一标识(客户所属应用)
*/
@Override
public ECustomer setSigma(String sigma) {
this.sigma = sigma;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.LANGUAGE
. 「language」- 使用的语言
*/
@Override
public String getLanguage() {
return this.language;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.LANGUAGE
. 「language」- 使用的语言
*/
@Override
public ECustomer setLanguage(String language) {
this.language = language;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CREATED_AT
. 「createdAt」-
* 创建时间
*/
@Override
public LocalDateTime getCreatedAt() {
return this.createdAt;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CREATED_AT
. 「createdAt」-
* 创建时间
*/
@Override
public ECustomer setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.CREATED_BY
. 「createdBy」-
* 创建人
*/
@Override
public String getCreatedBy() {
return this.createdBy;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.CREATED_BY
. 「createdBy」-
* 创建人
*/
@Override
public ECustomer setCreatedBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.UPDATED_AT
. 「updatedAt」-
* 更新时间
*/
@Override
public LocalDateTime getUpdatedAt() {
return this.updatedAt;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.UPDATED_AT
. 「updatedAt」-
* 更新时间
*/
@Override
public ECustomer setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_CUSTOMER.UPDATED_BY
. 「updatedBy」-
* 更新人
*/
@Override
public String getUpdatedBy() {
return this.updatedBy;
}
/**
* Setter for DB_ETERNAL.E_CUSTOMER.UPDATED_BY
. 「updatedBy」-
* 更新人
*/
@Override
public ECustomer setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("ECustomer (");
sb.append(key);
sb.append(", ").append(comment);
sb.append(", ").append(name);
sb.append(", ").append(title);
sb.append(", ").append(code);
sb.append(", ").append(taxCode);
sb.append(", ").append(taxTitle);
sb.append(", ").append(contactName);
sb.append(", ").append(contactPhone);
sb.append(", ").append(contactEmail);
sb.append(", ").append(contactOnline);
sb.append(", ").append(email);
sb.append(", ").append(fax);
sb.append(", ").append(homepage);
sb.append(", ").append(logo);
sb.append(", ").append(phone);
sb.append(", ").append(address);
sb.append(", ").append(runUp);
sb.append(", ").append(type);
sb.append(", ").append(metadata);
sb.append(", ").append(active);
sb.append(", ").append(sigma);
sb.append(", ").append(language);
sb.append(", ").append(createdAt);
sb.append(", ").append(createdBy);
sb.append(", ").append(updatedAt);
sb.append(", ").append(updatedBy);
sb.append(")");
return sb.toString();
}
// -------------------------------------------------------------------------
// FROM and INTO
// -------------------------------------------------------------------------
@Override
public void from(IECustomer from) {
setKey(from.getKey());
setComment(from.getComment());
setName(from.getName());
setTitle(from.getTitle());
setCode(from.getCode());
setTaxCode(from.getTaxCode());
setTaxTitle(from.getTaxTitle());
setContactName(from.getContactName());
setContactPhone(from.getContactPhone());
setContactEmail(from.getContactEmail());
setContactOnline(from.getContactOnline());
setEmail(from.getEmail());
setFax(from.getFax());
setHomepage(from.getHomepage());
setLogo(from.getLogo());
setPhone(from.getPhone());
setAddress(from.getAddress());
setRunUp(from.getRunUp());
setType(from.getType());
setMetadata(from.getMetadata());
setActive(from.getActive());
setSigma(from.getSigma());
setLanguage(from.getLanguage());
setCreatedAt(from.getCreatedAt());
setCreatedBy(from.getCreatedBy());
setUpdatedAt(from.getUpdatedAt());
setUpdatedBy(from.getUpdatedBy());
}
@Override
public E into(E into) {
into.from(this);
return into;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy