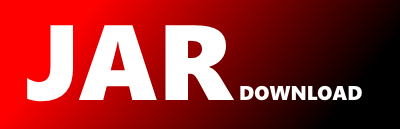
cn.vertxup.erp.domain.tables.pojos.EDept Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.erp.domain.tables.pojos;
import cn.vertxup.erp.domain.tables.interfaces.IEDept;
import io.github.jklingsporn.vertx.jooq.shared.internal.VertxPojo;
import java.time.LocalDateTime;
import static io.github.jklingsporn.vertx.jooq.shared.internal.VertxPojo.*;
/**
* This class is generated by jOOQ.
*/
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class EDept implements VertxPojo, IEDept {
private static final long serialVersionUID = 1L;
private String key;
private String name;
private String code;
private String managerId;
private String managerName;
private String companyId;
private String deptId;
private String comment;
private String metadata;
private Boolean active;
private String sigma;
private String language;
private LocalDateTime createdAt;
private String createdBy;
private LocalDateTime updatedAt;
private String updatedBy;
public EDept() {}
public EDept(IEDept value) {
this.key = value.getKey();
this.name = value.getName();
this.code = value.getCode();
this.managerId = value.getManagerId();
this.managerName = value.getManagerName();
this.companyId = value.getCompanyId();
this.deptId = value.getDeptId();
this.comment = value.getComment();
this.metadata = value.getMetadata();
this.active = value.getActive();
this.sigma = value.getSigma();
this.language = value.getLanguage();
this.createdAt = value.getCreatedAt();
this.createdBy = value.getCreatedBy();
this.updatedAt = value.getUpdatedAt();
this.updatedBy = value.getUpdatedBy();
}
public EDept(
String key,
String name,
String code,
String managerId,
String managerName,
String companyId,
String deptId,
String comment,
String metadata,
Boolean active,
String sigma,
String language,
LocalDateTime createdAt,
String createdBy,
LocalDateTime updatedAt,
String updatedBy
) {
this.key = key;
this.name = name;
this.code = code;
this.managerId = managerId;
this.managerName = managerName;
this.companyId = companyId;
this.deptId = deptId;
this.comment = comment;
this.metadata = metadata;
this.active = active;
this.sigma = sigma;
this.language = language;
this.createdAt = createdAt;
this.createdBy = createdBy;
this.updatedAt = updatedAt;
this.updatedBy = updatedBy;
}
public EDept(io.vertx.core.json.JsonObject json) {
this();
fromJson(json);
}
/**
* Getter for DB_ETERNAL.E_DEPT.KEY
. 「key」- 部门主键
*/
@Override
public String getKey() {
return this.key;
}
/**
* Setter for DB_ETERNAL.E_DEPT.KEY
. 「key」- 部门主键
*/
@Override
public EDept setKey(String key) {
this.key = key;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.NAME
. 「name」- 部门名称
*/
@Override
public String getName() {
return this.name;
}
/**
* Setter for DB_ETERNAL.E_DEPT.NAME
. 「name」- 部门名称
*/
@Override
public EDept setName(String name) {
this.name = name;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.CODE
. 「code」- 部门编号
*/
@Override
public String getCode() {
return this.code;
}
/**
* Setter for DB_ETERNAL.E_DEPT.CODE
. 「code」- 部门编号
*/
@Override
public EDept setCode(String code) {
this.code = code;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.MANAGER_ID
. 「managerId」- 部门经理
*/
@Override
public String getManagerId() {
return this.managerId;
}
/**
* Setter for DB_ETERNAL.E_DEPT.MANAGER_ID
. 「managerId」- 部门经理
*/
@Override
public EDept setManagerId(String managerId) {
this.managerId = managerId;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.MANAGER_NAME
. 「managerName」-
* 部门名称
*/
@Override
public String getManagerName() {
return this.managerName;
}
/**
* Setter for DB_ETERNAL.E_DEPT.MANAGER_NAME
. 「managerName」-
* 部门名称
*/
@Override
public EDept setManagerName(String managerName) {
this.managerName = managerName;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.COMPANY_ID
. 「companyId」- 所属公司
*/
@Override
public String getCompanyId() {
return this.companyId;
}
/**
* Setter for DB_ETERNAL.E_DEPT.COMPANY_ID
. 「companyId」- 所属公司
*/
@Override
public EDept setCompanyId(String companyId) {
this.companyId = companyId;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.DEPT_ID
. 「deptId」- 父部门
*/
@Override
public String getDeptId() {
return this.deptId;
}
/**
* Setter for DB_ETERNAL.E_DEPT.DEPT_ID
. 「deptId」- 父部门
*/
@Override
public EDept setDeptId(String deptId) {
this.deptId = deptId;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.COMMENT
. 「comment」- 部门备注
*/
@Override
public String getComment() {
return this.comment;
}
/**
* Setter for DB_ETERNAL.E_DEPT.COMMENT
. 「comment」- 部门备注
*/
@Override
public EDept setComment(String comment) {
this.comment = comment;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.METADATA
. 「metadata」- 附加配置
*/
@Override
public String getMetadata() {
return this.metadata;
}
/**
* Setter for DB_ETERNAL.E_DEPT.METADATA
. 「metadata」- 附加配置
*/
@Override
public EDept setMetadata(String metadata) {
this.metadata = metadata;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.ACTIVE
. 「active」- 是否启用
*/
@Override
public Boolean getActive() {
return this.active;
}
/**
* Setter for DB_ETERNAL.E_DEPT.ACTIVE
. 「active」- 是否启用
*/
@Override
public EDept setActive(Boolean active) {
this.active = active;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.SIGMA
. 「sigma」- 统一标识
*/
@Override
public String getSigma() {
return this.sigma;
}
/**
* Setter for DB_ETERNAL.E_DEPT.SIGMA
. 「sigma」- 统一标识
*/
@Override
public EDept setSigma(String sigma) {
this.sigma = sigma;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.LANGUAGE
. 「language」- 使用的语言
*/
@Override
public String getLanguage() {
return this.language;
}
/**
* Setter for DB_ETERNAL.E_DEPT.LANGUAGE
. 「language」- 使用的语言
*/
@Override
public EDept setLanguage(String language) {
this.language = language;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.CREATED_AT
. 「createdAt」- 创建时间
*/
@Override
public LocalDateTime getCreatedAt() {
return this.createdAt;
}
/**
* Setter for DB_ETERNAL.E_DEPT.CREATED_AT
. 「createdAt」- 创建时间
*/
@Override
public EDept setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.CREATED_BY
. 「createdBy」- 创建人
*/
@Override
public String getCreatedBy() {
return this.createdBy;
}
/**
* Setter for DB_ETERNAL.E_DEPT.CREATED_BY
. 「createdBy」- 创建人
*/
@Override
public EDept setCreatedBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.UPDATED_AT
. 「updatedAt」- 更新时间
*/
@Override
public LocalDateTime getUpdatedAt() {
return this.updatedAt;
}
/**
* Setter for DB_ETERNAL.E_DEPT.UPDATED_AT
. 「updatedAt」- 更新时间
*/
@Override
public EDept setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Getter for DB_ETERNAL.E_DEPT.UPDATED_BY
. 「updatedBy」- 更新人
*/
@Override
public String getUpdatedBy() {
return this.updatedBy;
}
/**
* Setter for DB_ETERNAL.E_DEPT.UPDATED_BY
. 「updatedBy」- 更新人
*/
@Override
public EDept setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("EDept (");
sb.append(key);
sb.append(", ").append(name);
sb.append(", ").append(code);
sb.append(", ").append(managerId);
sb.append(", ").append(managerName);
sb.append(", ").append(companyId);
sb.append(", ").append(deptId);
sb.append(", ").append(comment);
sb.append(", ").append(metadata);
sb.append(", ").append(active);
sb.append(", ").append(sigma);
sb.append(", ").append(language);
sb.append(", ").append(createdAt);
sb.append(", ").append(createdBy);
sb.append(", ").append(updatedAt);
sb.append(", ").append(updatedBy);
sb.append(")");
return sb.toString();
}
// -------------------------------------------------------------------------
// FROM and INTO
// -------------------------------------------------------------------------
@Override
public void from(IEDept from) {
setKey(from.getKey());
setName(from.getName());
setCode(from.getCode());
setManagerId(from.getManagerId());
setManagerName(from.getManagerName());
setCompanyId(from.getCompanyId());
setDeptId(from.getDeptId());
setComment(from.getComment());
setMetadata(from.getMetadata());
setActive(from.getActive());
setSigma(from.getSigma());
setLanguage(from.getLanguage());
setCreatedAt(from.getCreatedAt());
setCreatedBy(from.getCreatedBy());
setUpdatedAt(from.getUpdatedAt());
setUpdatedBy(from.getUpdatedBy());
}
@Override
public E into(E into) {
into.from(this);
return into;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy