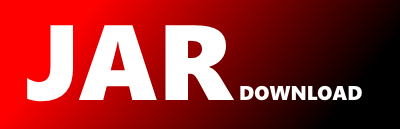
cn.vertxup.graphic.domain.tables.pojos.GEdge Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.graphic.domain.tables.pojos;
import cn.vertxup.graphic.domain.tables.interfaces.IGEdge;
import java.time.LocalDateTime;
import javax.annotation.Generated;
/**
* This class is generated by jOOQ.
*/
@Generated(
value = {
"http://www.jooq.org",
"jOOQ version:3.10.8"
},
comments = "This class is generated by jOOQ"
)
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class GEdge implements IGEdge {
private static final long serialVersionUID = 288176714;
private String key;
private String name;
private String type;
private String sourceKey;
private String targetKey;
private String graphicId;
private String ui;
private String recordKey;
private String recordData;
private String sigma;
private String language;
private Boolean active;
private String metadata;
private LocalDateTime createdAt;
private String createdBy;
private LocalDateTime updatedAt;
private String updatedBy;
public GEdge() {}
public GEdge(GEdge value) {
this.key = value.key;
this.name = value.name;
this.type = value.type;
this.sourceKey = value.sourceKey;
this.targetKey = value.targetKey;
this.graphicId = value.graphicId;
this.ui = value.ui;
this.recordKey = value.recordKey;
this.recordData = value.recordData;
this.sigma = value.sigma;
this.language = value.language;
this.active = value.active;
this.metadata = value.metadata;
this.createdAt = value.createdAt;
this.createdBy = value.createdBy;
this.updatedAt = value.updatedAt;
this.updatedBy = value.updatedBy;
}
public GEdge(
String key,
String name,
String type,
String sourceKey,
String targetKey,
String graphicId,
String ui,
String recordKey,
String recordData,
String sigma,
String language,
Boolean active,
String metadata,
LocalDateTime createdAt,
String createdBy,
LocalDateTime updatedAt,
String updatedBy
) {
this.key = key;
this.name = name;
this.type = type;
this.sourceKey = sourceKey;
this.targetKey = targetKey;
this.graphicId = graphicId;
this.ui = ui;
this.recordKey = recordKey;
this.recordData = recordData;
this.sigma = sigma;
this.language = language;
this.active = active;
this.metadata = metadata;
this.createdAt = createdAt;
this.createdBy = createdBy;
this.updatedAt = updatedAt;
this.updatedBy = updatedBy;
}
@Override
public String getKey() {
return this.key;
}
@Override
public GEdge setKey(String key) {
this.key = key;
return this;
}
@Override
public String getName() {
return this.name;
}
@Override
public GEdge setName(String name) {
this.name = name;
return this;
}
@Override
public String getType() {
return this.type;
}
@Override
public GEdge setType(String type) {
this.type = type;
return this;
}
@Override
public String getSourceKey() {
return this.sourceKey;
}
@Override
public GEdge setSourceKey(String sourceKey) {
this.sourceKey = sourceKey;
return this;
}
@Override
public String getTargetKey() {
return this.targetKey;
}
@Override
public GEdge setTargetKey(String targetKey) {
this.targetKey = targetKey;
return this;
}
@Override
public String getGraphicId() {
return this.graphicId;
}
@Override
public GEdge setGraphicId(String graphicId) {
this.graphicId = graphicId;
return this;
}
@Override
public String getUi() {
return this.ui;
}
@Override
public GEdge setUi(String ui) {
this.ui = ui;
return this;
}
@Override
public String getRecordKey() {
return this.recordKey;
}
@Override
public GEdge setRecordKey(String recordKey) {
this.recordKey = recordKey;
return this;
}
@Override
public String getRecordData() {
return this.recordData;
}
@Override
public GEdge setRecordData(String recordData) {
this.recordData = recordData;
return this;
}
@Override
public String getSigma() {
return this.sigma;
}
@Override
public GEdge setSigma(String sigma) {
this.sigma = sigma;
return this;
}
@Override
public String getLanguage() {
return this.language;
}
@Override
public GEdge setLanguage(String language) {
this.language = language;
return this;
}
@Override
public Boolean getActive() {
return this.active;
}
@Override
public GEdge setActive(Boolean active) {
this.active = active;
return this;
}
@Override
public String getMetadata() {
return this.metadata;
}
@Override
public GEdge setMetadata(String metadata) {
this.metadata = metadata;
return this;
}
@Override
public LocalDateTime getCreatedAt() {
return this.createdAt;
}
@Override
public GEdge setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
@Override
public String getCreatedBy() {
return this.createdBy;
}
@Override
public GEdge setCreatedBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
@Override
public LocalDateTime getUpdatedAt() {
return this.updatedAt;
}
@Override
public GEdge setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
@Override
public String getUpdatedBy() {
return this.updatedBy;
}
@Override
public GEdge setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("GEdge (");
sb.append(key);
sb.append(", ").append(name);
sb.append(", ").append(type);
sb.append(", ").append(sourceKey);
sb.append(", ").append(targetKey);
sb.append(", ").append(graphicId);
sb.append(", ").append(ui);
sb.append(", ").append(recordKey);
sb.append(", ").append(recordData);
sb.append(", ").append(sigma);
sb.append(", ").append(language);
sb.append(", ").append(active);
sb.append(", ").append(metadata);
sb.append(", ").append(createdAt);
sb.append(", ").append(createdBy);
sb.append(", ").append(updatedAt);
sb.append(", ").append(updatedBy);
sb.append(")");
return sb.toString();
}
// -------------------------------------------------------------------------
// FROM and INTO
// -------------------------------------------------------------------------
/**
* {@inheritDoc}
*/
@Override
public void from(IGEdge from) {
setKey(from.getKey());
setName(from.getName());
setType(from.getType());
setSourceKey(from.getSourceKey());
setTargetKey(from.getTargetKey());
setGraphicId(from.getGraphicId());
setUi(from.getUi());
setRecordKey(from.getRecordKey());
setRecordData(from.getRecordData());
setSigma(from.getSigma());
setLanguage(from.getLanguage());
setActive(from.getActive());
setMetadata(from.getMetadata());
setCreatedAt(from.getCreatedAt());
setCreatedBy(from.getCreatedBy());
setUpdatedAt(from.getUpdatedAt());
setUpdatedBy(from.getUpdatedBy());
}
/**
* {@inheritDoc}
*/
@Override
public E into(E into) {
into.from(this);
return into;
}
public GEdge(io.vertx.core.json.JsonObject json) {
fromJson(json);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy