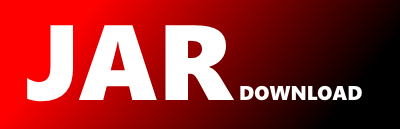
cn.vertxup.graphic.domain.tables.pojos.GGraphic Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.graphic.domain.tables.pojos;
import cn.vertxup.graphic.domain.tables.interfaces.IGGraphic;
import java.time.LocalDateTime;
import javax.annotation.Generated;
/**
* This class is generated by jOOQ.
*/
@Generated(
value = {
"http://www.jooq.org",
"jOOQ version:3.10.8"
},
comments = "This class is generated by jOOQ"
)
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class GGraphic implements IGGraphic {
private static final long serialVersionUID = 1855315510;
private String key;
private String name;
private String code;
private String mode;
private String type;
private String comments;
private String ownerId;
private String ui;
private String graphicId;
private Boolean master;
private String modelId;
private String modelKey;
private String modelCategory;
private String sigma;
private String language;
private Boolean active;
private String metadata;
private LocalDateTime createdAt;
private String createdBy;
private LocalDateTime updatedAt;
private String updatedBy;
public GGraphic() {}
public GGraphic(GGraphic value) {
this.key = value.key;
this.name = value.name;
this.code = value.code;
this.mode = value.mode;
this.type = value.type;
this.comments = value.comments;
this.ownerId = value.ownerId;
this.ui = value.ui;
this.graphicId = value.graphicId;
this.master = value.master;
this.modelId = value.modelId;
this.modelKey = value.modelKey;
this.modelCategory = value.modelCategory;
this.sigma = value.sigma;
this.language = value.language;
this.active = value.active;
this.metadata = value.metadata;
this.createdAt = value.createdAt;
this.createdBy = value.createdBy;
this.updatedAt = value.updatedAt;
this.updatedBy = value.updatedBy;
}
public GGraphic(
String key,
String name,
String code,
String mode,
String type,
String comments,
String ownerId,
String ui,
String graphicId,
Boolean master,
String modelId,
String modelKey,
String modelCategory,
String sigma,
String language,
Boolean active,
String metadata,
LocalDateTime createdAt,
String createdBy,
LocalDateTime updatedAt,
String updatedBy
) {
this.key = key;
this.name = name;
this.code = code;
this.mode = mode;
this.type = type;
this.comments = comments;
this.ownerId = ownerId;
this.ui = ui;
this.graphicId = graphicId;
this.master = master;
this.modelId = modelId;
this.modelKey = modelKey;
this.modelCategory = modelCategory;
this.sigma = sigma;
this.language = language;
this.active = active;
this.metadata = metadata;
this.createdAt = createdAt;
this.createdBy = createdBy;
this.updatedAt = updatedAt;
this.updatedBy = updatedBy;
}
@Override
public String getKey() {
return this.key;
}
@Override
public GGraphic setKey(String key) {
this.key = key;
return this;
}
@Override
public String getName() {
return this.name;
}
@Override
public GGraphic setName(String name) {
this.name = name;
return this;
}
@Override
public String getCode() {
return this.code;
}
@Override
public GGraphic setCode(String code) {
this.code = code;
return this;
}
@Override
public String getMode() {
return this.mode;
}
@Override
public GGraphic setMode(String mode) {
this.mode = mode;
return this;
}
@Override
public String getType() {
return this.type;
}
@Override
public GGraphic setType(String type) {
this.type = type;
return this;
}
@Override
public String getComments() {
return this.comments;
}
@Override
public GGraphic setComments(String comments) {
this.comments = comments;
return this;
}
@Override
public String getOwnerId() {
return this.ownerId;
}
@Override
public GGraphic setOwnerId(String ownerId) {
this.ownerId = ownerId;
return this;
}
@Override
public String getUi() {
return this.ui;
}
@Override
public GGraphic setUi(String ui) {
this.ui = ui;
return this;
}
@Override
public String getGraphicId() {
return this.graphicId;
}
@Override
public GGraphic setGraphicId(String graphicId) {
this.graphicId = graphicId;
return this;
}
@Override
public Boolean getMaster() {
return this.master;
}
@Override
public GGraphic setMaster(Boolean master) {
this.master = master;
return this;
}
@Override
public String getModelId() {
return this.modelId;
}
@Override
public GGraphic setModelId(String modelId) {
this.modelId = modelId;
return this;
}
@Override
public String getModelKey() {
return this.modelKey;
}
@Override
public GGraphic setModelKey(String modelKey) {
this.modelKey = modelKey;
return this;
}
@Override
public String getModelCategory() {
return this.modelCategory;
}
@Override
public GGraphic setModelCategory(String modelCategory) {
this.modelCategory = modelCategory;
return this;
}
@Override
public String getSigma() {
return this.sigma;
}
@Override
public GGraphic setSigma(String sigma) {
this.sigma = sigma;
return this;
}
@Override
public String getLanguage() {
return this.language;
}
@Override
public GGraphic setLanguage(String language) {
this.language = language;
return this;
}
@Override
public Boolean getActive() {
return this.active;
}
@Override
public GGraphic setActive(Boolean active) {
this.active = active;
return this;
}
@Override
public String getMetadata() {
return this.metadata;
}
@Override
public GGraphic setMetadata(String metadata) {
this.metadata = metadata;
return this;
}
@Override
public LocalDateTime getCreatedAt() {
return this.createdAt;
}
@Override
public GGraphic setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
@Override
public String getCreatedBy() {
return this.createdBy;
}
@Override
public GGraphic setCreatedBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
@Override
public LocalDateTime getUpdatedAt() {
return this.updatedAt;
}
@Override
public GGraphic setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
@Override
public String getUpdatedBy() {
return this.updatedBy;
}
@Override
public GGraphic setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("GGraphic (");
sb.append(key);
sb.append(", ").append(name);
sb.append(", ").append(code);
sb.append(", ").append(mode);
sb.append(", ").append(type);
sb.append(", ").append(comments);
sb.append(", ").append(ownerId);
sb.append(", ").append(ui);
sb.append(", ").append(graphicId);
sb.append(", ").append(master);
sb.append(", ").append(modelId);
sb.append(", ").append(modelKey);
sb.append(", ").append(modelCategory);
sb.append(", ").append(sigma);
sb.append(", ").append(language);
sb.append(", ").append(active);
sb.append(", ").append(metadata);
sb.append(", ").append(createdAt);
sb.append(", ").append(createdBy);
sb.append(", ").append(updatedAt);
sb.append(", ").append(updatedBy);
sb.append(")");
return sb.toString();
}
// -------------------------------------------------------------------------
// FROM and INTO
// -------------------------------------------------------------------------
/**
* {@inheritDoc}
*/
@Override
public void from(IGGraphic from) {
setKey(from.getKey());
setName(from.getName());
setCode(from.getCode());
setMode(from.getMode());
setType(from.getType());
setComments(from.getComments());
setOwnerId(from.getOwnerId());
setUi(from.getUi());
setGraphicId(from.getGraphicId());
setMaster(from.getMaster());
setModelId(from.getModelId());
setModelKey(from.getModelKey());
setModelCategory(from.getModelCategory());
setSigma(from.getSigma());
setLanguage(from.getLanguage());
setActive(from.getActive());
setMetadata(from.getMetadata());
setCreatedAt(from.getCreatedAt());
setCreatedBy(from.getCreatedBy());
setUpdatedAt(from.getUpdatedAt());
setUpdatedBy(from.getUpdatedBy());
}
/**
* {@inheritDoc}
*/
@Override
public E into(E into) {
into.from(this);
return into;
}
public GGraphic(io.vertx.core.json.JsonObject json) {
fromJson(json);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy