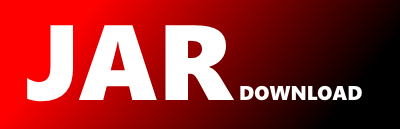
cn.vertxup.graphic.domain.tables.pojos.GNode Maven / Gradle / Ivy
/*
* This file is generated by jOOQ.
*/
package cn.vertxup.graphic.domain.tables.pojos;
import cn.vertxup.graphic.domain.tables.interfaces.IGNode;
import java.math.BigDecimal;
import java.time.LocalDateTime;
import javax.annotation.Generated;
/**
* This class is generated by jOOQ.
*/
@Generated(
value = {
"http://www.jooq.org",
"jOOQ version:3.10.8"
},
comments = "This class is generated by jOOQ"
)
@SuppressWarnings({ "all", "unchecked", "rawtypes" })
public class GNode implements IGNode {
private static final long serialVersionUID = 1252759814;
private String key;
private String name;
private BigDecimal x;
private BigDecimal y;
private String ui;
private String graphicId;
private String recordData;
private String recordKey;
private String recordComponent;
private String recordClass;
private String sigma;
private String language;
private Boolean active;
private String metadata;
private LocalDateTime createdAt;
private String createdBy;
private LocalDateTime updatedAt;
private String updatedBy;
public GNode() {}
public GNode(GNode value) {
this.key = value.key;
this.name = value.name;
this.x = value.x;
this.y = value.y;
this.ui = value.ui;
this.graphicId = value.graphicId;
this.recordData = value.recordData;
this.recordKey = value.recordKey;
this.recordComponent = value.recordComponent;
this.recordClass = value.recordClass;
this.sigma = value.sigma;
this.language = value.language;
this.active = value.active;
this.metadata = value.metadata;
this.createdAt = value.createdAt;
this.createdBy = value.createdBy;
this.updatedAt = value.updatedAt;
this.updatedBy = value.updatedBy;
}
public GNode(
String key,
String name,
BigDecimal x,
BigDecimal y,
String ui,
String graphicId,
String recordData,
String recordKey,
String recordComponent,
String recordClass,
String sigma,
String language,
Boolean active,
String metadata,
LocalDateTime createdAt,
String createdBy,
LocalDateTime updatedAt,
String updatedBy
) {
this.key = key;
this.name = name;
this.x = x;
this.y = y;
this.ui = ui;
this.graphicId = graphicId;
this.recordData = recordData;
this.recordKey = recordKey;
this.recordComponent = recordComponent;
this.recordClass = recordClass;
this.sigma = sigma;
this.language = language;
this.active = active;
this.metadata = metadata;
this.createdAt = createdAt;
this.createdBy = createdBy;
this.updatedAt = updatedAt;
this.updatedBy = updatedBy;
}
@Override
public String getKey() {
return this.key;
}
@Override
public GNode setKey(String key) {
this.key = key;
return this;
}
@Override
public String getName() {
return this.name;
}
@Override
public GNode setName(String name) {
this.name = name;
return this;
}
@Override
public BigDecimal getX() {
return this.x;
}
@Override
public GNode setX(BigDecimal x) {
this.x = x;
return this;
}
@Override
public BigDecimal getY() {
return this.y;
}
@Override
public GNode setY(BigDecimal y) {
this.y = y;
return this;
}
@Override
public String getUi() {
return this.ui;
}
@Override
public GNode setUi(String ui) {
this.ui = ui;
return this;
}
@Override
public String getGraphicId() {
return this.graphicId;
}
@Override
public GNode setGraphicId(String graphicId) {
this.graphicId = graphicId;
return this;
}
@Override
public String getRecordData() {
return this.recordData;
}
@Override
public GNode setRecordData(String recordData) {
this.recordData = recordData;
return this;
}
@Override
public String getRecordKey() {
return this.recordKey;
}
@Override
public GNode setRecordKey(String recordKey) {
this.recordKey = recordKey;
return this;
}
@Override
public String getRecordComponent() {
return this.recordComponent;
}
@Override
public GNode setRecordComponent(String recordComponent) {
this.recordComponent = recordComponent;
return this;
}
@Override
public String getRecordClass() {
return this.recordClass;
}
@Override
public GNode setRecordClass(String recordClass) {
this.recordClass = recordClass;
return this;
}
@Override
public String getSigma() {
return this.sigma;
}
@Override
public GNode setSigma(String sigma) {
this.sigma = sigma;
return this;
}
@Override
public String getLanguage() {
return this.language;
}
@Override
public GNode setLanguage(String language) {
this.language = language;
return this;
}
@Override
public Boolean getActive() {
return this.active;
}
@Override
public GNode setActive(Boolean active) {
this.active = active;
return this;
}
@Override
public String getMetadata() {
return this.metadata;
}
@Override
public GNode setMetadata(String metadata) {
this.metadata = metadata;
return this;
}
@Override
public LocalDateTime getCreatedAt() {
return this.createdAt;
}
@Override
public GNode setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
@Override
public String getCreatedBy() {
return this.createdBy;
}
@Override
public GNode setCreatedBy(String createdBy) {
this.createdBy = createdBy;
return this;
}
@Override
public LocalDateTime getUpdatedAt() {
return this.updatedAt;
}
@Override
public GNode setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
@Override
public String getUpdatedBy() {
return this.updatedBy;
}
@Override
public GNode setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("GNode (");
sb.append(key);
sb.append(", ").append(name);
sb.append(", ").append(x);
sb.append(", ").append(y);
sb.append(", ").append(ui);
sb.append(", ").append(graphicId);
sb.append(", ").append(recordData);
sb.append(", ").append(recordKey);
sb.append(", ").append(recordComponent);
sb.append(", ").append(recordClass);
sb.append(", ").append(sigma);
sb.append(", ").append(language);
sb.append(", ").append(active);
sb.append(", ").append(metadata);
sb.append(", ").append(createdAt);
sb.append(", ").append(createdBy);
sb.append(", ").append(updatedAt);
sb.append(", ").append(updatedBy);
sb.append(")");
return sb.toString();
}
// -------------------------------------------------------------------------
// FROM and INTO
// -------------------------------------------------------------------------
/**
* {@inheritDoc}
*/
@Override
public void from(IGNode from) {
setKey(from.getKey());
setName(from.getName());
setX(from.getX());
setY(from.getY());
setUi(from.getUi());
setGraphicId(from.getGraphicId());
setRecordData(from.getRecordData());
setRecordKey(from.getRecordKey());
setRecordComponent(from.getRecordComponent());
setRecordClass(from.getRecordClass());
setSigma(from.getSigma());
setLanguage(from.getLanguage());
setActive(from.getActive());
setMetadata(from.getMetadata());
setCreatedAt(from.getCreatedAt());
setCreatedBy(from.getCreatedBy());
setUpdatedAt(from.getUpdatedAt());
setUpdatedBy(from.getUpdatedBy());
}
/**
* {@inheritDoc}
*/
@Override
public E into(E into) {
into.from(this);
return into;
}
public GNode(io.vertx.core.json.JsonObject json) {
fromJson(json);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy