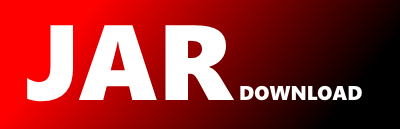
cn.woodwhales.common.business.DataTool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of woodwhales-common Show documentation
Show all versions of woodwhales-common Show documentation
https://github.com/woodwhales
package cn.woodwhales.common.business;
import com.google.common.base.Preconditions;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.collections4.MapUtils;
import java.util.*;
import java.util.function.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static java.util.Collections.emptyList;
import static java.util.Collections.emptyMap;
import static java.util.function.Function.identity;
import static org.apache.commons.collections4.CollectionUtils.isEmpty;
/**
* 业务处理工具类
* @author woodwhales
* 2020-11-17 13:44
*/
public class DataTool {
/**
* list 转 set 集合
* @param source 数据源集合
* @param mapper 按照源数据的 mapper 规则生成 set 元素
* @param 数据源集合中元素的类型
* @param set 集合中元素的类型
* @return set 集合
*/
public static Set toSet(List source,
Function super S, ? extends T> mapper) {
if(isEmpty(source)) {
return Collections.emptySet();
}
return source.stream().map(mapper).collect(Collectors.toSet());
}
/**
* list 转 map 集合
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param valueMapper map 集合中的 value 获取规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @param map 集合中的 value 类型
* @return map 集合
*/
public static Map toMap(List source,
Function super S, ? extends K> keyMapper,
Function super S, ? extends T> valueMapper) {
if(isEmpty(source)) {
return Collections.emptyMap();
}
return source.stream().collect(Collectors.toMap(keyMapper, valueMapper));
}
/**
* list 转 map 集合
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param valueMapper map 集合中的 value 获取规则
* @param mergeFunction 存在相同 key 时取 value 的规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @param map 集合中的 value 类型
* @return map 集合
*/
public static Map toMap(Collection source,
Function super S, ? extends K> keyMapper,
Function super S, ? extends T> valueMapper,
BinaryOperator mergeFunction) {
if(isEmpty(source)) {
return Collections.emptyMap();
}
return source.stream().collect(Collectors.toMap(keyMapper, valueMapper, mergeFunction));
}
/**
* list 转 map 集合
* map 的 value 为集合元素本身
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @return map 集合
*/
public static Map toMap(List source,
Function super S, ? extends K> keyMapper) {
return toMap(source, keyMapper, identity());
}
/**
* list 转 map 集合
* map 的 value 为集合元素本身
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param mergeFunction 存在相同 key 时取 value 的规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @return map 集合
*/
public static Map toMap(List source,
Function super S, ? extends K> keyMapper,
BinaryOperator mergeFunction) {
return toMap(source, keyMapper, identity(), mergeFunction);
}
/**
* 将原始的 list 按照 mapper 规则转成新的 list
* 再按照新的 list 按照 keyMapper 为生成 key 规则转成 map 集合
* @param source 数据源集合
* @param mapper list 转 map 的规则
* @param keyMapper map 集合中的 key 获取规则
* @param 数据源集合中元素的类型
* @param 目标数据的类型
* @param map 集合中的 key 类型
* @return list
*/
public static Map toMapFromList(List source,
Function super S, ? extends T> mapper,
Function super T, ? extends K> keyMapper) {
if (isEmpty(source)) {
return Collections.emptyMap();
}
return toMap(source.stream().map(mapper).collect(Collectors.toList()), keyMapper);
}
/**
* list 转 map 集合
* map 的 value 为集合元素本身
* 如果出现 key 重复,则取前一个元素
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @return map 集合
*/
public static Map toMapForSaveOld(List source,
Function super S, ? extends K> keyMapper) {
return toMap(source, keyMapper, identity(), (o1, o2) -> o1);
}
/**
* list 转 map 集合
* 如果出现 key 重复,则取前一个元素
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param valueMapper map 集合中的 value 获取规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @param map 集合中的 value 类型
* @return map 集合
*/
public static Map toMapForSaveOld(List source,
Function super S, ? extends K> keyMapper,
Function super S, ? extends T> valueMapper) {
return toMap(source, keyMapper, valueMapper, (o1, o2) -> o1);
}
/**
* list 转 map 集合
* map 的 value 为集合元素本身
* 如果出现 key 重复,则取前一个元素
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @return map 集合
*/
public static Map toMapForSaveNew(Collection source,
Function super S, ? extends K> keyMapper) {
return toMap(source, keyMapper, identity(), (o1, o2) -> o2);
}
/**
* list 转 map 集合
* 如果出现 key 重复,则取前一个元素
* @param source 数据源集合
* @param keyMapper map 集合中的 key 获取规则
* @param valueMapper map 集合中的 value 获取规则
* @param map 集合中的 key 类型
* @param 数据源集合中元素的类型
* @param map 集合中的 value 类型
* @return map 集合
*/
public static Map toMapForSaveNew(List source,
Function super S, ? extends K> keyMapper,
Function super S, ? extends T> valueMapper) {
return toMap(source, keyMapper, valueMapper, (o1, o2) -> o2);
}
/**
* 将原始的 list 按照 mapper 规则转成新的 list
* @param source 源数据集合
* @param mapper 生成新的 list 接口规则
* @param 源数据类型
* @param 目标数据类型
* @return list
*/
public static List toList(List source,
Function super S, ? extends T> mapper) {
return toList(source, mapper, false);
}
/**
* 将原始的 array 按照 mapper 规则转成新的 list
* @param array 源数据数组
* @param mapper 生成新的 list 接口规则
* @param 源数据类型
* @param 目标数据类型
* @return list
*/
public static List toList(S[] array,
Function super S, ? extends T> mapper) {
return toList(Arrays.asList(array), mapper);
}
/**
* 将原始的 list 按照 mapper 规则转成新的 list
* @param source 源数据集合
* @param mapper 生成新的 list 接口规则
* @param distinct 是否去重
* @param 源数据类型
* @param 目标数据类型
* @return list
*/
public static List toList(List source,
Function super S, ? extends T> mapper,
boolean distinct) {
if (isEmpty(source)) {
return Collections.emptyList();
}
if(distinct) {
return source.stream().map(mapper).distinct().collect(Collectors.toList());
}
return source.stream().map(mapper).collect(Collectors.toList());
}
/**
* list 遍历并匹配 map 中的元素,根据 discard 决定元素未匹配到 map 是否丢弃
* @param source 源数据集合
* @param map mpa 集合
* @param keyFunction 生成 key 的接口
* @param mapContainKeyFunction 生成 list 的接口
* @param discard 决定元素未匹配到 map 是否丢弃:true 丢弃,false 不丢弃
* @param 源数据集合元素类型
* @param 目标数据集合元素类型
* @param map 集合的 key 类型
* @param map 集合的 value 类型
* @return list
*/
private static List toListWithMap(List source, Map map,
Function keyFunction,
BiFunction mapContainKeyFunction,
boolean discard,
Function function) {
Preconditions.checkNotNull(keyFunction, "keyFunction不允许为空");
Preconditions.checkNotNull(mapContainKeyFunction, "mapContainKeyFunction不允许为空");
if(!discard) {
Preconditions.checkNotNull(function, "list元素不存在map集合中的处理接口function不允许为空");
}
if (isEmpty(source)) {
return Collections.emptyList();
}
if(MapUtils.isEmpty(map)) {
map = emptyMap();
}
List result = new ArrayList<>();
for (S s : source) {
K key = keyFunction.apply(s);
if(map.containsKey(key)) {
V v = map.get(key);
T t = mapContainKeyFunction.apply(s, v);
result.add(t);
} else {
if(!discard) {
T t = function.apply(s);
result.add(t);
}
}
}
return result;
}
/**
* list 遍历并匹配 map 中的元素,没有匹配到则丢弃
* @param source 源数据集合
* @param map mpa 集合
* @param keyFunction 生成 key 的接口
* @param mapContainKeyFunction 生成 list 的接口
* @param 源数据集合元素类型
* @param 目标数据集合元素类型
* @param map 集合的 key 类型
* @param map 集合的 value 类型
* @return list
*/
public static List toListWithMap(List source, Map map,
Function keyFunction,
BiFunction mapContainKeyFunction) {
return toListWithMap(source, map, keyFunction, mapContainKeyFunction, true, null);
}
/**
* list 遍历并匹配 map 中的元素,没有匹配到不丢弃
* @param source 源数据集合
* @param map mpa 集合
* @param keyFunction 生成 key 的接口
* @param mapContainKeyFunction 生成 list 的接口
* @param function 生成 list 的接口
* @param 源数据集合元素类型
* @param 目标数据集合元素类型
* @param map 集合的 key 类型
* @param map 集合的 value 类型
* @return list
*/
public static List toListWithMap(List source, Map map,
Function keyFunction,
BiFunction mapContainKeyFunction,
Function function) {
Preconditions.checkNotNull(function, "function不允许为空");
return toListWithMap(source, map, keyFunction, mapContainKeyFunction, false, function);
}
/**
* 将原始的 list 按照 filter 过滤之后,按照 mapper 规则转成新的 list
* @param source 源数据集合
* @param filter 源数据集合过滤规则
* @param mapper 生成新的 list 接口规则
* @param distinct 是否去重 distinct 为 true,表示过滤之后生成list之前进行去重操作
* @param 源数据类型
* @param 目标数据类型
* @return list
*/
public static List toList(List source,
Predicate filter,
Function super S, ? extends T> mapper,
boolean distinct) {
if (isEmpty(source)) {
return Collections.emptyList();
}
Stream extends T> stream = source.stream()
.filter(filter::test)
.map(mapper);
if(distinct) {
stream = stream.distinct();
}
return stream.collect(Collectors.toList());
}
/**
* 将原始的 list 按照 filter 过滤之后,按照 mapper 规则转成新的 list
*
* @param source 源数据数组
* @param filter 源数据集合过滤规则
* @param mapper 生成新的 list 接口规则
* @param distinct 是否去重 distinct 为 true,表示过滤之后生成list之前进行去重操作
* @param 源数据类型
* @param 目标数据类型
* @return list
*/
public static List toList(S[] source,
Predicate filter,
Function super S, ? extends T> mapper,
boolean distinct) {
if (Objects.isNull(source)) {
return Collections.emptyList();
}
Stream extends T> stream = Stream.of(source)
.filter(filter::test)
.map(mapper);
if(distinct) {
stream = stream.distinct();
}
return stream.collect(Collectors.toList());
}
/**
* 将原始的 list 按照 filter 过滤之后,按照 mapper 规则转成新的 list
* @param source 源数据集合
* @param filter 源数据集合过滤规则
* @param mapper 生成新的 list 接口规则
* @param 源数据类型
* @param 目标数据类型
* @return list
*/
public static List toList(List source,
Predicate filter,
Function super S, ? extends T> mapper) {
return toList(source, filter, mapper, false);
}
/**
*
* 从 map 中遍历生成 list
* @param map 源数据 map 集合
* @param function 生成 list 规则
* @param map 集合的 key 类型
* @param map 集合的数据类型
* @param 目标数据类型
* @return list
*/
public static List toListByMap(Map map,
BiFunction function) {
if(MapUtils.isEmpty(map)) {
return emptyList();
}
return map.entrySet().stream()
.map(entry -> function.apply(entry.getKey(), entry.getValue()))
.collect(Collectors.toList());
}
/**
* 从过滤的 map 中遍历生成 list
* @param map 源数据 map 集合
* @param filterMapMapper 过滤 map 的接口规则
* @param function 生成 list 规则
* @param map 集合的 key 类型
* @param map 集合的数据类型
* @param 目标数据类型
* @return list
*/
public static List toListByMap(Map map,
BiPredicate filterMapMapper,
BiFunction function) {
if(MapUtils.isEmpty(map)) {
return emptyList();
}
return map.entrySet().stream()
.filter(entry -> filterMapMapper.test(entry.getKey(), entry.getValue()))
.map(entry -> function.apply(entry.getKey(), entry.getValue()))
.collect(Collectors.toList());
}
/**
* 将 list 集合分组
* @param source 数据源集合
* @param classifier 分组规则
* @param map 集合中的 key 类型
* @param map 集合中的 value 类型
* @return list
*/
public static Map> groupingBy(List source,
Function super S, ? extends K> classifier) {
if(isEmpty(source)) {
return Collections.emptyMap();
}
return source.stream().collect(Collectors.groupingBy(classifier));
}
/**
* 将过滤的 list 集合分组
* @param source 数据源集合
* @param filter 过滤源数据集合的接口规则
* @param classifier 分组规则
* @param map 集合中的 key 类型
* @param map 集合中的 value 类型
* @return list
*/
public static Map> groupingBy(List source,
Predicate filter,
Function super S, ? extends K> classifier) {
if(isEmpty(source)) {
return Collections.emptyMap();
}
return source.stream()
.filter(filter::test)
.collect(Collectors.groupingBy(classifier));
}
/**
* 对 list 集合进行排序
* @param source 数据源集合
* @param comparator 排序器
* @param 集合的数据类型
* @return list
*/
public static List sort(List source, Comparator comparator) {
return sort(source, comparator, true);
}
/**
* 对 list 集合进行排序
* @param source 数据源集合
* @param comparator 排序器
* @param reverse 是否逆序
* @param 集合的数据类型
* @return list
*/
public static List sort(List source,
Comparator comparator,
boolean reverse) {
if(isEmpty(source)) {
return source;
}
Stream stream = source.stream();
Stream sorted;
if(reverse) {
sorted = stream.sorted(comparator.reversed());
} else {
sorted = stream.sorted(comparator);
}
return sorted.collect(Collectors.toList());
}
/**
* 对集合数据进行去重器, 默认保留最后出现的重复元素
* 非线程安全
* @param source 数据源集合
* @param isValidFunction 数据是否有效
* @param getDeduplicatedKeyFunction 获取去重的数据值
* @param 去重属性的类型
* @param 数据源集合中元素的类型
* @return DeduplicateResult
*/
public static DeduplicateResult deduplicate(List source,
Function isValidFunction,
Function getDeduplicatedKeyFunction) {
return deduplicate(source, isValidFunction, getDeduplicatedKeyFunction, false);
}
/**
* 对集合数据进行去重器, 默认保留最后出现的重复元素
* 非线程安全
* @param source 数据源集合
* @param getDeduplicatedKeyFunction 获取去重的数据值
* @param 去重属性的类型
* @param 数据源集合中元素的类型
* @return DeduplicateResult
*/
public static DeduplicateResult deduplicate(List source,
Function getDeduplicatedKeyFunction) {
return deduplicate(source, null, getDeduplicatedKeyFunction, false);
}
/**
* 对集合数据进行去重器
* 非线程安全
* @param source 数据源集合
* @param getDeduplicatedKeyFunction 获取去重的数据值
* @param remainFirst 是否保留第一次出现的重复值
* @param 去重属性的类型
* @param 数据源集合中元素的类型
* @return DeduplicateResult
*/
public static DeduplicateResult deduplicate(List source,
Function getDeduplicatedKeyFunction,
boolean remainFirst) {
return deduplicate(source, null, getDeduplicatedKeyFunction, remainFirst);
}
/**
* 对集合数据进行去重器
* 非线程安全
* @param source 数据源集合
* @param isValidFunction 数据是否有效
* @param getDeduplicatedKeyFunction 获取去重的数据值
* @param remainFirst 是否保留第一次出现的重复值
* @param 去重属性的类型
* @param 数据源集合中元素的类型
* @return DeduplicateResult
*/
public static DeduplicateResult deduplicate(List source,
Function isValidFunction,
Function getDeduplicatedKeyFunction,
boolean remainFirst) {
if(isEmpty(source)) {
return new DeduplicateResult(source, emptyList(), emptyList(), emptyList(), emptyList());
}
Map container = new LinkedHashMap<>();
// 无效的数据集合
List invalidList = new LinkedList<>();
// 重复的数据集合
List repetitiveList = new LinkedList<>();
for (T data : source) {
if (Objects.nonNull(isValidFunction) && !Objects.equals(true, isValidFunction.apply(data))) {
invalidList.add(data);
} else {
K deduplicatedKey = getDeduplicatedKeyFunction.apply(data);
if (container.containsKey(deduplicatedKey)) {
T putData;
if(remainFirst) {
putData = data;
} else {
putData = container.put(deduplicatedKey, data);
}
if(Objects.nonNull(putData)) {
repetitiveList.add(putData);
}
} else {
container.put(deduplicatedKey, data);
}
}
}
// 已去重的数据集合
List deduplicatedList = new ArrayList<>(container.values());
List deduplicatedKeyList = new ArrayList<>(container.keySet());
return new DeduplicateResult(source, invalidList, deduplicatedList, deduplicatedKeyList, repetitiveList);
}
/**
* 枚举转 map 集合
* @param sourceEnumClass 数据源枚举 Class类
* @param keyMapper map 集合中的 key 获取规则
* @param map 集合中的 key 类型
* @param map 集合中的 value 类型
* @return map 集合
*/
public static > Map enumMap(Class sourceEnumClass,
Function keyMapper) {
EnumSet enumSet = EnumSet.allOf(sourceEnumClass);
return enumSet.stream().collect(Collectors.toMap(keyMapper, Function.identity()));
}
/**
* 枚举转 map 集合
* map 集合中的 key 为 Enum 的 name() 方法返回值
* @param sourceEnumClass 数据源枚举 Class类
* @param map 集合中的 value 类型
* @return map 集合
*/
public static > Map enumMap(Class sourceEnumClass) {
EnumSet enumSet = EnumSet.allOf(sourceEnumClass);
return enumSet.stream().collect(Collectors.toMap(Enum::name, Function.identity()));
}
/**
* 枚举转 list 集合
* 按照 function 将枚举转成 list 集合
* @param sourceEnumClass 数据源枚举 Class类
* @param function 生成接口
* @param 枚举类型
* @param 结果集类型
* @return list
*/
public static , R> List enumList(Class sourceEnumClass,
Function function) {
EnumSet enumSet = EnumSet.allOf(sourceEnumClass);
return enumSet.stream().map(function).collect(Collectors.toList());
}
/**
* 判断 key 是否存在于枚举转 map 的集合中
* @param key 要判断的 key
* @param sourceEnumClass 数据源枚举 Class类
* @param keyMapper map 集合中的 key 获取规则
* @param map 集合中的 key 类型
* @param map 集合中的 value 类型
* @return map
*/
public static > boolean enumContainsKey(K key, Class sourceEnumClass,
Function keyMapper) {
if(Objects.isNull(key)) {
return false;
}
Map extends K, T> map = enumMap(sourceEnumClass, keyMapper);
return map.containsKey(key);
}
/**
* 根据 key 获取对应的枚举实例
* @param key 要获取枚举实例的 key
* @param sourceEnumClass 数据源枚举 Class类
* @param keyMapper map 集合中的 key 获取规则
* @param map 集合中的 key 类型
* @param map 集合中的 value 类型
* @return 枚举实例
*/
public static > T enumGetValue(K key, Class sourceEnumClass,
Function keyMapper) {
if(Objects.isNull(key)) {
return null;
}
Map map = enumMap(sourceEnumClass, keyMapper);
return map.get(key);
}
/**
* 将原始的 list 按照 filter 过滤
* @param source 数据原始集合
* @param filter 过滤规则
* @param 数据类型
* @return list
*/
public static List filter(List source,
Predicate super T> filter) {
if (isEmpty(source)) {
return Collections.emptyList();
}
return source.stream()
.filter(filter)
.collect(Collectors.toList());
}
/**
* 将原始的 list 按照 filter 过滤
* @param source 数据原始数组
* @param filter 过滤规则
* @param 数据类型
* @return list
*/
public static List filter(T[] source,
Predicate super T> filter) {
if (Objects.isNull(source)) {
return Collections.emptyList();
}
return Stream.of(source)
.filter(filter)
.collect(Collectors.toList());
}
/**
* 根据 searchData 按照 searchFunction 规则从 sourceList 集合中搜索数据
* @param searchData 要搜索的源数据
* @param searchFunction 搜索的key
* @param sourceList 被搜索的数据源
* @param keyFunction 被搜索的数据源索引生成规则
* @param 要搜索的源数据类型
* @param 搜索的 key 类型
* @param 被搜索的数据源类型
* @return 被搜索的数据源
*/
public static T getDataFromList(M searchData,
Function searchFunction,
List sourceList,
Function keyFunction) {
Preconditions.checkNotNull(searchFunction, "function不允许为空");
if(isEmpty(sourceList)) {
return null;
}
return toMapForSaveNew(sourceList, keyFunction).get(searchFunction.apply(searchData));
}
/**
* 对原始 map 进行数据操作
* @param map 源 map 集合
* @param handlerMapper 集合元素处理接口规则
* @param map 集合的 key 数据类型
* @param map 集合的 value 数据类型
* @return 返回原始 map 集合
*/
public static Map handleMap(Map map,
BiConsumer handlerMapper) {
if(MapUtils.isEmpty(map)) {
return emptyMap();
}
for (Map.Entry entry : map.entrySet()) {
K key = entry.getKey();
T value = entry.getValue();
handlerMapper.accept(key, value);
}
return map;
}
/**
* 去除 list 集合中的 null 元素进行
* @param oldList 原始数据集合
* @param 原始数据类型
* @return list
*/
public static List removeNull(List extends T> oldList) {
oldList.removeAll(Collections.singleton(null));
return (List) oldList;
}
/**
* 从 map 中获取 value 集合并根据 function 转成 R 集合
* @param map 原始数据源集合
* @param function 映射接口
* @param key 类型
* @param value 类型
* @param 目标数据类型
* @return list
*/
public static