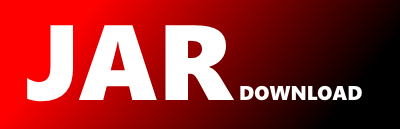
cn.woodwhales.common.business.tree.TreeNodeAttributeMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of woodwhales-common Show documentation
Show all versions of woodwhales-common Show documentation
https://github.com/woodwhales
package cn.woodwhales.common.business.tree;
import java.util.function.Function;
import static java.util.Objects.nonNull;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
/**
* @author woodwhales on 2020-12-11
*/
public class TreeNodeAttributeMapper {
/**
* 当前节点 id 的别名
*/
private String nodeId = "id";
/**
* 覆盖节点 id 对应数值的接口
*/
private Function overNodeIdFunction;
/**
* 当前节点名称变量的别名
*/
private String nodeName = "name";
/**
* 当前节点的父节点变量的别名
*/
private String parentId = "parentId";
/**
* 子节点变量的别名
*/
private String childrenName = "children";
/**
* 源数据变量的别名
*/
private String dataName = "data";
/**
* 当前节点的排序变量的别名
*/
private String sortName = "sort";
/**
* 扩展变量的别名
*/
private String extraName = "extra";
private TreeNodeAttributeMapper() {
}
public static TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder builder() {
return new TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder();
}
public static class TreeNodeAttributeMapperBuilder {
private String nodeId;
private Function overNodeIdFunction;
private String nodeName;
private String parentId;
private String childrenName;
private String dataName;
private String sortName;
private String extraName;
TreeNodeAttributeMapperBuilder() {}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder nodeId(String nodeId) {
this.nodeId = nodeId;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder overNodeId(Function overNodeIdFunction) {
this.overNodeIdFunction = overNodeIdFunction;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder overNodeId(String nodeId, Function overNodeIdFunction) {
this.nodeId = nodeId;
this.overNodeIdFunction = overNodeIdFunction;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder nodeName(String nodeName) {
this.nodeName = nodeName;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder parentId(String parentId) {
this.parentId = parentId;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder childrenName(String childrenName) {
this.childrenName = childrenName;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder dataName(String dataName) {
this.dataName = dataName;
return this;
}
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder sortName(String sortName) {
this.sortName = sortName;
return this;
}
/**
* 设置字段变量的别名
* 扩展字段的别名会覆盖掉已有的数据字段数据
* @param extraName 扩展字段变量的别名
* @return TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder
*/
public TreeNodeAttributeMapper.TreeNodeAttributeMapperBuilder extraName(String extraName) {
this.extraName = extraName;
return this;
}
public TreeNodeAttributeMapper build() {
return new TreeNodeAttributeMapper()
.setNodeId(this.nodeId)
.setOverNodeIdFunction(overNodeIdFunction)
.setNodeName(this.nodeName)
.setParentId(this.parentId)
.setChildrenName(this.childrenName)
.setDataName(this.dataName)
.setSortName(this.sortName)
.setExtraName(this.extraName);
}
}
public TreeNodeAttributeMapper setNodeId(String nodeId) {
if(isNotBlank(nodeId)) {
this.nodeId = nodeId;
}
return this;
}
public TreeNodeAttributeMapper setOverNodeIdFunction(Function overNodeIdFunction) {
if(nonNull(overNodeIdFunction)) {
this.overNodeIdFunction = overNodeIdFunction;
}
return this;
}
public TreeNodeAttributeMapper setNodeName(String nodeName) {
if(isNotBlank(nodeName)) {
this.nodeName = nodeName;
}
return this;
}
public TreeNodeAttributeMapper setParentId(String parentId) {
if(isNotBlank(parentId)) {
this.parentId = parentId;
}
return this;
}
public TreeNodeAttributeMapper setChildrenName(String childrenName) {
if(isNotBlank(childrenName)) {
this.childrenName = childrenName;
}
return this;
}
public TreeNodeAttributeMapper setDataName(String dataName) {
if(isNotBlank(dataName)) {
this.dataName = dataName;
}
return this;
}
public TreeNodeAttributeMapper setSortName(String sortName) {
if(isNotBlank(sortName)) {
this.sortName = sortName;
}
return this;
}
public TreeNodeAttributeMapper setExtraName(String extraName) {
if(isNotBlank(extraName)) {
this.extraName = extraName;
}
return this;
}
public String getNodeId() {
return nodeId;
}
public Function getOverNodeIdFunction() {
return overNodeIdFunction;
}
public String getNodeName() {
return nodeName;
}
public String getParentId() {
return parentId;
}
public String getChildrenName() {
return childrenName;
}
public String getDataName() {
return dataName;
}
public String getSortName() {
return sortName;
}
public String getExtraName() {
return extraName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy