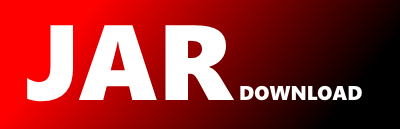
cn.woodwhales.common.webhook.executor.BaseWebhookExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of woodwhales-common Show documentation
Show all versions of woodwhales-common Show documentation
https://github.com/woodwhales
package cn.woodwhales.common.webhook.executor;
import cn.woodwhales.common.webhook.enums.WebhookProductEnum;
import cn.woodwhales.common.webhook.model.param.ExecuteParam;
import cn.woodwhales.common.webhook.model.request.BaseWebhookRequestBody;
import cn.woodwhales.common.webhook.model.response.ExecuteResponse;
import com.google.gson.Gson;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.lang.reflect.ParameterizedType;
import static java.util.Objects.nonNull;
/**
* 请求执行器
* @author woodwhales on 2021-07-16 21:24
*/
@Slf4j
public abstract class BaseWebhookExecutor {
/**
* 请求之前的处理方法
* @param executeParam executeParam
*/
protected void beforeHandler(ExecuteParam executeParam) {
return;
}
/**
* 请求之后的处理方法
* @param executeParam executeParam
* @param executeResponse executeResponse
*/
protected void afterHandler(ExecuteParam executeParam, ExecuteResponse executeResponse) {
return;
}
/**
* 校验解析后的响应结果
* @param executeResponse executeResponse
* @return 是都校验通过
*/
protected abstract boolean checkResponseObjectHandler(ExecuteResponse executeResponse);
/**
* 解析响应结果
* @param executeResponse executeResponse
* @return 解析响应结果
*/
protected Response parseResponseHandler(ExecuteResponse executeResponse) {
ParameterizedType genericSuperclass = (ParameterizedType)this.getClass()
.getGenericSuperclass();
Class clazz = (Class)genericSuperclass.getActualTypeArguments()[1];
return new Gson().fromJson(executeResponse.originResponseContent, clazz);
}
/**
* 校验响应结果失败之后的处理
* @param executeParam executeParam
* @param executeResponse executeResponse
*/
protected void checkFailHandler(ExecuteParam executeParam, ExecuteResponse executeResponse) {
log.error("{}发送消息失败, requestContent = {}, originResponseContent = {}",
webhookProductEnum().chineseName,
executeParam.content,
executeResponse.originResponseContent);
}
/**
* 校验响应结果成功之后的处理
* @param executeParam executeParam
* @param executeResponse executeResponse
*/
protected void checkSuccessHandler(ExecuteParam executeParam, ExecuteResponse executeResponse) {
log.info("{}发送消息成功, requestContent = {}, originResponseContent = {}",
webhookProductEnum().chineseName,
executeParam.content,
executeResponse.originResponseContent);
}
/**
* webhook 产品信息
* @return WebhookProductEnum
*/
protected abstract WebhookProductEnum webhookProductEnum();
/**
* 执行发送消息
* @param url 请求地址
* @param content 请求报文
*/
protected void execute(String url, String content) {
this.execute(ExecuteParam.newInstance(url, content));
}
/**
* 执行发送消息
* @param executeParam executeParam
*/
protected void execute(ExecuteParam executeParam) {
// 请求之前处理
this.beforeHandler(executeParam);
// 执行请求
ExecuteResponse executeResponse = executeRequest(executeParam);
// 解析请求
executeResponse.parsedResponseObject = this.parseResponseHandler(executeResponse);
// 校验请求
executeResponse.checkResult = this.checkResponseObjectHandler(executeResponse);
if(!executeResponse.checkResult) {
this.checkFailHandler(executeParam, executeResponse);
} else {
this.checkSuccessHandler(executeParam, executeResponse);
}
// 请求之后处理
this.afterHandler(executeParam, executeResponse);
}
private ExecuteResponse executeRequest(ExecuteParam executeParam) {
ExecuteResponse executeResponse = null;
try (CloseableHttpClient httpClient = HttpClients.createDefault()){
HttpPost post = new HttpPost(executeParam.url);
post.setHeader("Accept","aplication/json");
post.setHeader("Content-Type", "application/json;charset=UTF-8");
StringEntity se = new StringEntity(executeParam.content, "UTF-8");
se.setContentEncoding("UTF-8");
se.setContentType("application/json");
post.setEntity(se);
CloseableHttpResponse response = httpClient.execute(post);
int statusCode = response.getStatusLine().getStatusCode();
String originResponseContent = EntityUtils.toString(response.getEntity());
executeResponse = new ExecuteResponse<>(statusCode, originResponseContent);
} catch (Exception e) {
log.error("{}发送消息失败, 异常原因:", e.getMessage(), e);
}
return executeResponse;
}
/**
* 执行请求
* @param url 请求地址
* @param requestBody 请求报文
*/
public void execute(String url, RequestBody requestBody) {
if(nonNull(requestBody)) {
this.execute(url, requestBody.toJsonSting());
} else {
log.warn("dingTalkRequestBody is NULL");
}
}
protected Response getParsedResponse(ExecuteResponse executeResponse) {
return executeResponse.parsedResponseObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy