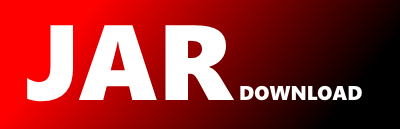
cn.wsyjlly.mavlink.common.v2.messages.LogErase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mavlink Show documentation
Show all versions of mavlink Show documentation
mavlink protocol description
The newest version!
package cn.wsyjlly.mavlink.common.v2.messages;
import cn.wsyjlly.mavlink.annotation.MavlinkMessage;
import cn.wsyjlly.mavlink.annotation.MavlinkMessageParam;
import cn.wsyjlly.mavlink.common.Message;
import cn.wsyjlly.mavlink.protocol.ByteArray;
import cn.wsyjlly.mavlink.protocol.util.ByteModel;
import java.util.Objects;
/**********************************
* Author YSW
* Description
* Date 2020.11.26 - 02:46
**********************************/
@MavlinkMessage(
id = 121,
messagePayloadLength = 2,
description = "Erase all logs"
)
public class LogErase implements Message {
@MavlinkMessageParam(mavlinkType = "uint8_t", position = 1, typeSize = 1, streamLength = 1, description = "System ID")
private short targetSystem;
@MavlinkMessageParam(mavlinkType = "uint8_t", position = 2, typeSize = 1, streamLength = 1, description = "Component ID")
private short targetComponent;
private final int messagePayloadLength = 2;
private byte[] messagePayload = new byte[messagePayloadLength];
public LogErase(short targetSystem, short targetComponent) {
this.targetSystem = targetSystem;
this.targetComponent = targetComponent;
}
public LogErase(byte[] messagePayload) {
if (messagePayload.length != messagePayloadLength){
throw new IllegalArgumentException("Byte array length is not equal to 2!");
}
messagePayload(messagePayload);
}
public LogErase(){ }
@Override
public void messagePayload(byte[] messagePayload) {
this.messagePayload = messagePayload;
ByteArray byteArray = new ByteArray(messagePayload);
targetSystem = byteArray.getUnsignedInt8(0);
targetComponent = byteArray.getUnsignedInt8(1);
}
@Override
public byte[] messagePayload() {
ByteArray byteArray = new ByteArray(messagePayload);
byteArray.putUnsignedInt8(targetSystem,0);
byteArray.putUnsignedInt8(targetComponent,1);
return messagePayload;
}
@Override
public String hexStringPayload() {
return ByteModel.bytes2HexString(messagePayload);
}
public final LogErase setTargetSystem(short targetSystem) {
this.targetSystem = targetSystem;
return this;
}
public final short getTargetSystem() {
return targetSystem;
}
public final LogErase setTargetComponent(short targetComponent) {
this.targetComponent = targetComponent;
return this;
}
public final short getTargetComponent() {
return targetComponent;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
} else if (o != null && this.getClass().equals(o.getClass())) {
LogErase other = (LogErase)o;
if (!Objects.deepEquals(this.targetSystem, other.targetSystem)) {
return false;
} else {
return Objects.deepEquals(this.targetComponent, other.targetComponent);
}
} else {
return false;
}
}
@Override
public int hashCode() {
int result = 0;
result = 31 * result + Objects.hashCode(this.targetSystem);
result = 31 * result + Objects.hashCode(this.targetComponent);
return result;
}
@Override
public String toString() {
return "LogErase{" +
"targetSystem=" + targetSystem +
", targetComponent=" + targetComponent +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy