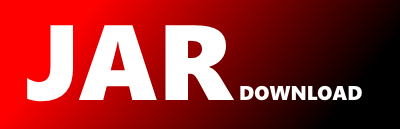
cn.zcltd.http.response.HttpResponseHandler Maven / Gradle / Ivy
package cn.zcltd.http.response;
import org.apache.http.Header;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpUriRequest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.URLEncoder;
import java.text.SimpleDateFormat;
import java.util.Date;
public abstract class HttpResponseHandler {
protected final Logger log = LoggerFactory.getLogger(this.getClass());
protected String charset = "UTF-8";
protected Boolean printLog = false;
protected Boolean printFile = false;
protected String reqFilePath = this.getClass().getResource("/").getPath();
public HttpResponseHandler() {
}
public HttpResponseHandler(String charset) {
this.charset = charset;
}
public String getCharset() {
return charset;
}
public void setCharset(String charset) {
this.charset = charset;
}
public Boolean getPrintLog() {
return printLog;
}
public void setPrintLog(Boolean printLog) {
this.printLog = printLog;
}
public Boolean getPrintFile() {
return printFile;
}
public void setPrintFile(Boolean printFile) {
this.printFile = printFile;
}
public String getReqFilePath() {
return reqFilePath;
}
public void setReqFilePath(String reqFilePath) {
reqFilePath = reqFilePath.replaceAll("\\\\", "/");
reqFilePath = reqFilePath.endsWith("/") ? reqFilePath : reqFilePath + "/";
this.reqFilePath = reqFilePath;
}
/**
* response处理程序
*
* @param request request
* @param response response
* @return 处理后结果
* @throws Exception 异常
*/
public abstract HttpResponseResult handler(HttpUriRequest request, HttpResponse response) throws Exception;
public void print(HttpUriRequest request, HttpResponse response, HttpResponseResult responseResult) {
try {
StringBuilder resultSb = new StringBuilder("\n");
resultSb.append(String.format("URL:%s\n", request.getURI()));
resultSb.append(String.format("Method:%s\n", request.getMethod()));
Header[] allHeaders = request.getAllHeaders();
resultSb.append(String.format("RequestHeaders:\n"));
for (Header allHeader : allHeaders) {
resultSb.append(String.format("\t%s:%s\n", allHeader.getName(), allHeader.getValue()));
}
resultSb.append(String.format("ResponseCode:%s\n", response.getStatusLine().getStatusCode()));
resultSb.append(String.format("ResponseHeaders:\n"));
for (Header allHeader : response.getAllHeaders()) {
resultSb.append(String.format("\t%s:%s\n", allHeader.getName(), allHeader.getValue()));
}
resultSb.append(String.format("ResponseEntity:\n"));
InputStream is = responseResult.getResponseBody();
byte[] b = new byte[1024];
int l;
while ((l = is.read(b)) != -1) {
resultSb.append(new String(b, 0, l, this.charset));
}
if (printLog) {
log.debug(resultSb.toString());
}
if (printFile) {
File dir = new File(reqFilePath);
if (!dir.exists() || !dir.isDirectory()) {
boolean isSuccess = dir.mkdirs();
if (!isSuccess) {
throw new RuntimeException("directory does not exist and create the failure," + dir.getCanonicalPath());
}
}
String fileName = new SimpleDateFormat("yyyyMMddHHmmssSSS").format(new Date()) + request.getMethod() + "-" + URLEncoder.encode(request.getURI().getPath(), "UTF-8") + ".req";
File file = new File(reqFilePath + fileName);
FileOutputStream outputStream = new FileOutputStream(file);
outputStream.write(resultSb.toString().getBytes());
outputStream.close();
}
} catch (Exception e) {
log.error(e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy