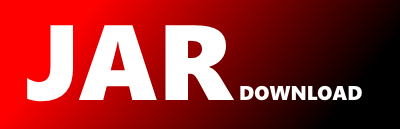
co.cask.common.config.ReflectionUtils Maven / Gradle / Ivy
/*
* Copyright © 2014 Cask Data, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package co.cask.common.config;
import java.lang.reflect.Constructor;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* General reflection utils.
*/
public class ReflectionUtils {
private static final Class>[] EMPTY_ARRAY = new Class[]{};
/**
* Cache of constructors for each class. Pins the classes so they
* can't be garbage collected until ReflectionUtils can be collected.
*/
private static final Map, Constructor>> CONSTRUCTOR_CACHE =
new ConcurrentHashMap, Constructor>>();
/**
* Check and set 'configuration' if necessary.
*
* @param theObject object for which to set configuration
* @param conf Configuration
*/
private static void setConf(Object theObject, Configuration conf) {
if (conf != null) {
if (theObject instanceof Configurable) {
((Configurable) theObject).setConf(conf);
}
}
}
/** Create an object for the given class and initialize it from conf.
*
* @param theClass class of which an object is created
* @param conf Configuration
* @return a new object
*/
@SuppressWarnings("unchecked")
public static T newInstance(Class theClass, Configuration conf) {
T result;
try {
Constructor meth = (Constructor) CONSTRUCTOR_CACHE.get(theClass);
if (meth == null) {
meth = theClass.getDeclaredConstructor(EMPTY_ARRAY);
meth.setAccessible(true);
CONSTRUCTOR_CACHE.put(theClass, meth);
}
result = meth.newInstance();
} catch (Exception e) {
throw new RuntimeException(e);
}
setConf(result, conf);
return result;
}
/**
* Return the correctly-typed {@link Class} of the given object.
*
* @param o object whose correctly-typed Class
is to be obtained
* @return the correctly typed Class
of the given object.
*/
@SuppressWarnings("unchecked")
public static Class getClass(T o) {
return (Class) o.getClass();
}
// methods to support testing
static void clearCache() {
CONSTRUCTOR_CACHE.clear();
}
static int getCacheSize() {
return CONSTRUCTOR_CACHE.size();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy