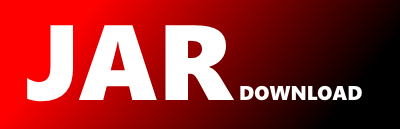
co.cask.wrangler.utils.StructuredRecordConverter Maven / Gradle / Ivy
/*
* Copyright © 2017 Cask Data, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package co.cask.wrangler.utils;
import co.cask.cdap.api.data.format.StructuredRecord;
import co.cask.cdap.api.data.format.UnexpectedFormatException;
import co.cask.cdap.api.data.schema.Schema;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import java.io.IOException;
import java.lang.reflect.Array;
import java.util.List;
import java.util.Map;
/**
* Converts an {@link StructuredRecord} to subset of the input {@link StructuredRecord} based
* on the {@link Schema} specified.
*/
public final class StructuredRecordConverter {
public static StructuredRecord transform(StructuredRecord record, Schema schema) throws IOException {
StructuredRecord.Builder builder = StructuredRecord.builder(schema);
for (Schema.Field field : record.getSchema().getFields()) {
String name = field.getName();
// If the field name is not in the output, then skip it and if it's not nullable, then
// it would be error out -- in which case, the user has to fix the schema to proceed.
if (schema.getField(name) != null) {
builder.set(name, convertField(record.get(name), field.getSchema()));
}
}
return builder.build();
}
private static Object convertUnion(Object value, List schemas) {
boolean isNullable = false;
for (Schema possibleSchema : schemas) {
if (possibleSchema.getType() == Schema.Type.NULL) {
isNullable = true;
if (value == null) {
return value;
}
} else {
try {
return convertField(value, possibleSchema);
} catch (Exception e) {
// if we couldn't convert, move to the next possibility
}
}
}
if (isNullable) {
return null;
}
throw new UnexpectedFormatException("Unable to determine the union type.");
}
private static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy