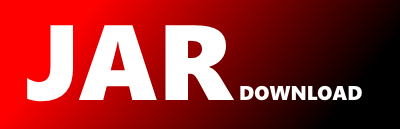
co.com.sofka.infraestructure.handle.CommandExecutor Maven / Gradle / Ivy
package co.com.sofka.infraestructure.handle;
import co.com.sofka.business.asyn.UseCaseExecutor;
import co.com.sofka.business.generic.UseCase;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Consumer;
import java.util.logging.Logger;
/**
* The type Command executor.
*/
public abstract class CommandExecutor implements CommandHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy