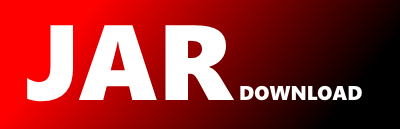
matcher.driver.MatcherExploitStringTester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of regex-static-analysis Show documentation
Show all versions of regex-static-analysis Show documentation
A tool to perform static analysis on regexes to determine whether they are vulnerable to ReDoS.
package matcher.driver;
import matcher.*;
import regexcompiler.*;
import analysis.AnalysisSettings.NFAConstruction;
public class MatcherExploitStringTester {
public static void main(String args[]) {
if (args.length < 4 || args.length % 2 != 0) {
System.out.println("usage: ... ");
System.exit(0);
}
String patternStr = args[0];
int numPumpSeparators = (args.length - 2) / 2;
int numPumps = (args.length - 2) / 2;
String prefix = makeVerbatim(args[1]);
String[] pumpSeparators = new String[numPumpSeparators];
String[] pumps = new String[numPumps];
for (int i = 1; i < args.length - 1; i += 2) {
pumpSeparators[(i - 1) / 2] = makeVerbatim(args[i]);
pumps[(i - 1) / 2] = makeVerbatim(args[i + 1]);
}
for (int i = 0; i < numPumps; i++) {
System.out.println("ps_" + i + ": " + pumps[i] + " p_" + i + ": " + pumpSeparators[i]);
}
String suffix = makeVerbatim(args[args.length - 1]);
int counter = 1;
StringBuilder sb = new StringBuilder("Trying to exploit " + patternStr + " with ");
for (int i = 1; i < args.length - 1; i += 2) {
sb.append(args[i] + args[i + 1] + "..." + args[i + 1]);
}
sb.append(suffix);
System.out.println(sb.toString());
StringBuilder[] pumpers = new StringBuilder[numPumps];
for (int i = 0; i < numPumps; i++) {
pumpers[i] = new StringBuilder(pumps[i]);
}
while (true) {
StringBuilder exploitStringBuilder = new StringBuilder();
for (int i = 0; i < numPumps; i++) {
exploitStringBuilder.append(pumpSeparators[i]);
exploitStringBuilder.append(pumpers[i].toString());
pumpers[i].append(pumps[i]);
}
exploitStringBuilder.append(suffix);
String exploitString = exploitStringBuilder.toString();
//System.out.println(exploitString);
MyPattern pattern = MyPattern.compile(patternStr, NFAConstruction.JAVA);
MyMatcher matcher = pattern.matcher(exploitString);
long startTime = System.currentTimeMillis();
boolean matches = matcher.matches();
long endTime = System.currentTimeMillis();
System.out.println("Iteration: " + counter + "| String length: " + exploitString.length() + "| Match time: " + (endTime - startTime));
counter++;
}
}
private static String makeVerbatim(String s) {
String toReturn = s;
java.util.regex.Pattern hexCharsPattern = java.util.regex.Pattern.compile("\\\\x([0-9a-fA-F]{2})");
java.util.regex.Matcher m = hexCharsPattern.matcher(s);
while (m.find()) {
toReturn = toReturn.replaceAll(java.util.regex.Matcher.quoteReplacement(m.group(0)), java.util.regex.Matcher.quoteReplacement("" + (char) Integer.parseInt(m.group(1), 16)));
}
return toReturn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy