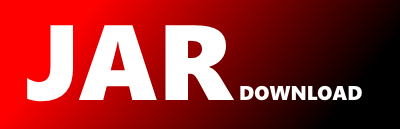
co.decodable.sdk.pipeline.internal.DelegatingSourceReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of decodable-pipeline-sdk Show documentation
Show all versions of decodable-pipeline-sdk Show documentation
A software development kit for implementing Apache Flink jobs and running them on Decodable
/*
* SPDX-License-Identifier: Apache-2.0
*
* Copyright Decodable, Inc.
*
* Licensed under the Apache Software License version 2.0, available at http://www.apache.org/licenses/LICENSE-2.0
*/
package co.decodable.sdk.pipeline.internal;
import co.decodable.sdk.pipeline.DecodableSourceSplit;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.stream.Collectors;
import org.apache.flink.api.connector.source.ReaderOutput;
import org.apache.flink.api.connector.source.SourceReader;
import org.apache.flink.connector.kafka.source.split.KafkaPartitionSplit;
import org.apache.flink.core.io.InputStatus;
public class DelegatingSourceReader implements SourceReader {
private final SourceReader delegate;
public DelegatingSourceReader(SourceReader delegate) {
this.delegate = delegate;
}
@Override
public void close() throws Exception {
delegate.close();
}
@Override
public void start() {
delegate.start();
}
@Override
public InputStatus pollNext(ReaderOutput output) throws Exception {
return delegate.pollNext(output);
}
@Override
public List snapshotState(long checkpointId) {
return delegate.snapshotState(checkpointId).stream()
.map(DecodableSourceSplitImpl::new)
.collect(Collectors.toList());
}
@Override
public CompletableFuture isAvailable() {
return delegate.isAvailable();
}
@Override
public void addSplits(List splits) {
List delegateSplits =
splits.stream()
.map(s -> ((DecodableSourceSplitImpl) s).getDelegate())
.collect(Collectors.toList());
delegate.addSplits(delegateSplits);
}
@Override
public void notifyNoMoreSplits() {
delegate.notifyNoMoreSplits();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy