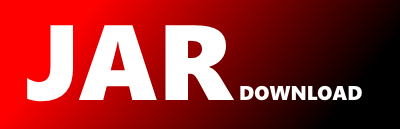
co.easimart.provider.Artwork Maven / Gradle / Ivy
package co.easimart.provider;
import android.content.ContentValues;
import android.content.Context;
import android.content.Intent;
import android.database.Cursor;
import android.net.Uri;
import android.os.Bundle;
import android.text.TextUtils;
import org.json.JSONException;
import org.json.JSONObject;
import java.net.URISyntaxException;
/**
* A serializable object representing a single artwork produced by a {@link ArtSource}. An
* artwork is simple an {@linkplain Artwork.Builder#imageUri(Uri) image} along with
* some metadata.
*
* To create an instance, use the {@link Artwork.Builder} class.
*/
public class Artwork {
public static final String FONT_TYPE_DEFAULT = "";
public static final String FONT_TYPE_ELEGANT = "elegant";
private static final String KEY_IMAGE_URI = "imageUri";
private static final String KEY_TITLE = "title";
private static final String KEY_BYLINE = "byline";
private static final String KEY_ATTRIBUTION = "attribution";
private static final String KEY_TOKEN = "token";
private static final String KEY_VIEW_INTENT = "viewIntent";
private static final String KEY_DETAILS_URI = "detailsUri";
private static final String KEY_META_FONT = "metaFont";
private Uri mImageUri;
private String mTitle;
private String mByline;
private String mAttribution;
private String mToken;
private Intent mViewIntent;
private String mMetaFont;
private Artwork() {
}
/**
* Returns the artwork's image URI, or null if it doesn't have one.
*
* @see Artwork.Builder#imageUri(Uri)
*/
public Uri getImageUri() {
return mImageUri;
}
/**
* Returns the artwork's user-visible title, or null if it doesn't have one.
*
* @see Artwork.Builder#title(String)
*/
public String getTitle() {
return mTitle;
}
/**
* Returns the artwork's user-visible byline (e.g. author and date), or null if it doesn't have
* one.
*
* @see Artwork.Builder#byline(String)
*/
public String getByline() {
return mByline;
}
/**
* Returns the artwork's user-visible attribution text, or null if it doesn't have any.
*
* @see Artwork.Builder#attribution(String)
*/
public String getAttribution() {
return mAttribution;
}
/**
* Returns the artwork's opaque application-specific identifier, or null if it doesn't have
* one.
*
* @see Artwork.Builder#token(String)
*/
public String getToken() {
return mToken;
}
/**
* Returns the activity {@link Intent} that will be started when the user clicks
* for more details about the artwork, or null if the artwork doesn't have one.
*
* @see Artwork.Builder#viewIntent(Intent)
*/
public Intent getViewIntent() {
return mViewIntent;
}
/**
* Returns the font type to use for showing metadata.
*
* @see Artwork.Builder#metaFont(String)
*/
public String getMetaFont() {
return mMetaFont;
}
/**
* Sets the artwork's image URI.
*
* @see Artwork.Builder#imageUri(Uri)
*/
public void setImageUri(Uri imageUri) {
mImageUri = imageUri;
}
/**
* Sets the artwork's user-visible title.
*
* @see Artwork.Builder#title(String)
*/
public void setTitle(String title) {
mTitle = title;
}
/**
* Sets the artwork's user-visible byline (e.g. author and date).
*
* @see Artwork.Builder#byline(String)
*/
public void setByline(String byline) {
mByline = byline;
}
/**
* Sets the artwork's user-visible attribution text.
*
* @see Artwork.Builder#attribution(String)
*/
public void setAttribution(String attribution) {
mAttribution = attribution;
}
/**
* Sets the artwork's opaque application-specific identifier.
*
* @see Artwork.Builder#token(String)
*/
public void setToken(String token) {
mToken = token;
}
/**
* Sets the activity {@link Intent} that will be started when the user clicks
* for more details about the artwork.
*
* @see Artwork.Builder#viewIntent(Intent)
*/
public void setViewIntent(Intent viewIntent) {
mViewIntent = viewIntent;
}
/**
* Sets the font type to use for showing metadata.
*
* @see Artwork.Builder#metaFont(String)
*/
public void setMetaFont(String metaFont) {
mMetaFont = metaFont;
}
/**
* A builder-style, fluent interface for creating {@link
* Artwork} objects. Example usage is below.
*
*
* Artwork artwork = new Artwork.Builder()
* .imageUri(Uri.parse("http://example.com/image.jpg"))
* .title("Example image")
* .byline("Unknown person, c. 1980")
* .attribution("Copyright (C) Unknown person, 1980")
* .viewIntent(new Intent(Intent.ACTION_VIEW,
* Uri.parse("http://example.com/imagedetails.html")))
* .build();
*
*
* The only required field is {@linkplain #imageUri(Uri) the image URI}, but you
* should really provide all the metadata, especially title, byline, and view intent.
*/
public static class Builder {
private Artwork mArtwork;
public Builder() {
mArtwork = new Artwork();
}
/**
* Sets the artwork's image URI, which must resolve to a JPEG or PNG image, ideally
* under 5MB. Supported URI schemes are:
*
*
* content://...
. Content URIs must be public (i.e. not require
* permissions). To build a file-based content provider, see the
* FileProvider
* class in the Android support library.
* http://...
or https://...
. These URLs must be
* publicly accessible (i.e. not require authentication of any kind).
*
*
* While Muzei will download and cache the artwork, these URIs should be as long-lived as
* possible, since in the event Muzei's cache is wiped out, it will attempt to fetch the
* image again. Also, given that the device may not be connected to the network at the time
* an artwork is {@linkplain ArtSource#publishArtwork(Artwork) published}, the time
* the URI may be fetched significantly after the artwork is published.
*/
public Builder imageUri(Uri imageUri) {
mArtwork.mImageUri = imageUri;
return this;
}
/**
* Sets the artwork's user-visible title.
*/
public Builder title(String title) {
mArtwork.mTitle = title;
return this;
}
/**
* Sets the artwork's user-visible byline (e.g. author and date).
*/
public Builder byline(String byline) {
mArtwork.mByline = byline;
return this;
}
/**
* Sets the artwork's user-visible attribution text.
*/
public Builder attribution(String attribution) {
mArtwork.mAttribution = attribution;
return this;
}
/**
* Sets the artwork's opaque application-specific identifier.
*/
public Builder token(String token) {
mArtwork.mToken = token;
return this;
}
/**
* Sets the activity {@link Intent} that will be
* {@linkplain Context#startActivity(Intent) started} when
* the user clicks for more details about the artwork.
*
* The activity that this intent resolves to must have android:exported
* set to true
.
*
*
Because artwork objects can be persisted across device reboots,
* {@linkplain android.app.PendingIntent pending intents}, which would alleviate the
* exported requirement, are not currently supported.
*/
public Builder viewIntent(Intent viewIntent) {
mArtwork.mViewIntent = viewIntent;
return this;
}
/**
* Sets the font type to use to show metadata for the artwork.
*
* @see #FONT_TYPE_DEFAULT
* @see #FONT_TYPE_ELEGANT
*/
public Builder metaFont(String metaFont) {
mArtwork.mMetaFont = metaFont;
return this;
}
/**
* Creates and returns the final Artwork object. Once this method is called, it is not valid
* to further use this {@link Artwork.Builder} object.
*/
public Artwork build() {
return mArtwork;
}
}
/**
* Serializes this artwork object to a {@link Bundle} representation.
*/
public Bundle toBundle() {
Bundle bundle = new Bundle();
bundle.putString(KEY_IMAGE_URI, (mImageUri != null) ? mImageUri.toString() : null);
bundle.putString(KEY_TITLE, mTitle);
bundle.putString(KEY_BYLINE, mByline);
bundle.putString(KEY_ATTRIBUTION, mAttribution);
bundle.putString(KEY_TOKEN, mToken);
bundle.putString(KEY_VIEW_INTENT, (mViewIntent != null)
? mViewIntent.toUri(Intent.URI_INTENT_SCHEME) : null);
bundle.putString(KEY_META_FONT, mMetaFont);
return bundle;
}
/**
* Deserializes an artwork object from a {@link Bundle}.
*/
public static Artwork fromBundle(Bundle bundle) {
Builder builder = new Builder()
.title(bundle.getString(KEY_TITLE))
.byline(bundle.getString(KEY_BYLINE))
.attribution(bundle.getString(KEY_ATTRIBUTION))
.token(bundle.getString(KEY_TOKEN))
.metaFont(bundle.getString(KEY_META_FONT));
String imageUri = bundle.getString(KEY_IMAGE_URI);
if (!TextUtils.isEmpty(imageUri)) {
builder.imageUri(Uri.parse(imageUri));
}
try {
String viewIntent = bundle.getString(KEY_VIEW_INTENT);
if (!TextUtils.isEmpty(viewIntent)) {
builder.viewIntent(Intent.parseUri(viewIntent, Intent.URI_INTENT_SCHEME));
}
} catch (URISyntaxException ignored) {
}
return builder.build();
}
/**
* Serializes this artwork object to a {@link JSONObject} representation.
*/
public JSONObject toJson() throws JSONException {
JSONObject jsonObject = new JSONObject();
jsonObject.put(KEY_IMAGE_URI, (mImageUri != null) ? mImageUri.toString() : null);
jsonObject.put(KEY_TITLE, mTitle);
jsonObject.put(KEY_BYLINE, mByline);
jsonObject.put(KEY_ATTRIBUTION, mAttribution);
jsonObject.put(KEY_TOKEN, mToken);
jsonObject.put(KEY_VIEW_INTENT, (mViewIntent != null)
? mViewIntent.toUri(Intent.URI_INTENT_SCHEME) : null);
jsonObject.put(KEY_META_FONT, mMetaFont);
return jsonObject;
}
/**
* Deserializes an artwork object from a {@link JSONObject}.
*/
public static Artwork fromJson(JSONObject jsonObject) throws JSONException {
Builder builder = new Builder()
.title(jsonObject.optString(KEY_TITLE))
.byline(jsonObject.optString(KEY_BYLINE))
.attribution(jsonObject.optString(KEY_ATTRIBUTION))
.token(jsonObject.optString(KEY_TOKEN))
.metaFont(jsonObject.optString(KEY_META_FONT));
String imageUri = jsonObject.optString(KEY_IMAGE_URI);
if (!TextUtils.isEmpty(imageUri)) {
builder.imageUri(Uri.parse(imageUri));
}
try {
String viewIntent = jsonObject.optString(KEY_VIEW_INTENT);
String detailsUri = jsonObject.optString(KEY_DETAILS_URI);
if (!TextUtils.isEmpty(viewIntent)) {
builder.viewIntent(Intent.parseUri(viewIntent, Intent.URI_INTENT_SCHEME));
} else if (!TextUtils.isEmpty(detailsUri)) {
builder.viewIntent(new Intent(Intent.ACTION_VIEW, Uri.parse(detailsUri)));
}
} catch (URISyntaxException ignored) {
}
return builder.build();
}
/**
* Serializes this artwork object to a {@link ContentValues} representation.
*/
public ContentValues toContentValues() {
ContentValues values = new ContentValues();
values.put(DbContract.Artwork.COLUMN_NAME_IMAGE_URI, (mImageUri != null)
? mImageUri.toString() : null);
values.put(DbContract.Artwork.COLUMN_NAME_TITLE, mTitle);
values.put(DbContract.Artwork.COLUMN_NAME_BYLINE, mByline);
values.put(DbContract.Artwork.COLUMN_NAME_ATTRIBUTION, mAttribution);
values.put(DbContract.Artwork.COLUMN_NAME_TOKEN, mToken);
values.put(DbContract.Artwork.COLUMN_NAME_VIEW_INTENT, (mViewIntent != null)
? mViewIntent.toUri(Intent.URI_INTENT_SCHEME) : null);
values.put(DbContract.Artwork.COLUMN_NAME_META_FONT, mMetaFont);
return values;
}
/**
* Deserializes an artwork object from a {@link Cursor}.
*/
public static Artwork fromCursor(Cursor cursor) {
Builder builder = new Builder();
int imageUriColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_IMAGE_URI);
if (imageUriColumnIndex != -1) {
builder.imageUri(Uri.parse(cursor.getString(imageUriColumnIndex)));
}
int titleColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_TITLE);
if (titleColumnIndex != -1) {
builder.title(cursor.getString(titleColumnIndex));
}
int bylineColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_BYLINE);
if (bylineColumnIndex != -1) {
builder.byline(cursor.getString(bylineColumnIndex));
}
int attributionColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_ATTRIBUTION);
if (attributionColumnIndex != -1) {
builder.attribution(cursor.getString(attributionColumnIndex));
}
int tokenColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_TOKEN);
if (tokenColumnIndex != -1) {
builder.token(cursor.getString(tokenColumnIndex));
}
int viewIntentColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_VIEW_INTENT);
if (viewIntentColumnIndex != -1) {
try {
String viewIntent = cursor.getString(viewIntentColumnIndex);
if (!TextUtils.isEmpty(viewIntent)) {
builder.viewIntent(Intent.parseUri(viewIntent, Intent.URI_INTENT_SCHEME));
}
} catch (URISyntaxException ignored) {
}
}
int metaFontColumnIndex = cursor.getColumnIndex(DbContract.Artwork.COLUMN_NAME_META_FONT);
if (metaFontColumnIndex != -1) {
builder.metaFont(cursor.getString(metaFontColumnIndex));
}
return builder.build();
}
}