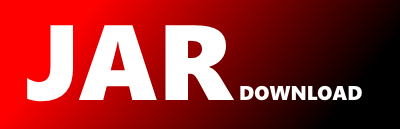
co.elastic.clients.elasticsearch.security.ElasticsearchSecurityAsyncClient Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.security;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.BooleanResponse;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the security namespace.
*/
public class ElasticsearchSecurityAsyncClient
extends
ApiClient {
public ElasticsearchSecurityAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchSecurityAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchSecurityAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchSecurityAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: security.activate_user_profile
/**
* Creates or updates a user profile on behalf of another user.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture activateUserProfile(ActivateUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ActivateUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates or updates a user profile on behalf of another user.
*
* @param fn
* a function that initializes a builder to create the
* {@link ActivateUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture activateUserProfile(
Function> fn) {
return activateUserProfile(fn.apply(new ActivateUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.authenticate
/**
* Enables you to submit a request with a basic auth header to authenticate a
* user and retrieve information about the authenticated user. A successful call
* returns a JSON structure that shows user information such as their username,
* the roles that are assigned to the user, any assigned metadata, and
* information about the realms that authenticated and authorized the user. If
* the user cannot be authenticated, this API returns a 401 status code.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture authenticate() {
return this.transport.performRequestAsync(AuthenticateRequest._INSTANCE, AuthenticateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.bulk_delete_role
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk delete roles API
* cannot delete roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture bulkDeleteRole(BulkDeleteRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) BulkDeleteRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk delete roles API
* cannot delete roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link BulkDeleteRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture bulkDeleteRole(
Function> fn) {
return bulkDeleteRole(fn.apply(new BulkDeleteRoleRequest.Builder()).build());
}
// ----- Endpoint: security.bulk_put_role
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk create or update roles
* API cannot update roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture bulkPutRole(BulkPutRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) BulkPutRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk create or update roles
* API cannot update roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link BulkPutRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture bulkPutRole(
Function> fn) {
return bulkPutRole(fn.apply(new BulkPutRoleRequest.Builder()).build());
}
// ----- Endpoint: security.change_password
/**
* Changes the passwords of users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture changePassword(ChangePasswordRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ChangePasswordRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Changes the passwords of users in the native realm and built-in users.
*
* @param fn
* a function that initializes a builder to create the
* {@link ChangePasswordRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture changePassword(
Function> fn) {
return changePassword(fn.apply(new ChangePasswordRequest.Builder()).build());
}
/**
* Changes the passwords of users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture changePassword() {
return this.transport.performRequestAsync(new ChangePasswordRequest.Builder().build(),
ChangePasswordRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.clear_api_key_cache
/**
* Evicts a subset of all entries from the API key cache. The cache is also
* automatically cleared on state changes of the security index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearApiKeyCache(ClearApiKeyCacheRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearApiKeyCacheRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evicts a subset of all entries from the API key cache. The cache is also
* automatically cleared on state changes of the security index.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearApiKeyCacheRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearApiKeyCache(
Function> fn) {
return clearApiKeyCache(fn.apply(new ClearApiKeyCacheRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_privileges
/**
* Evicts application privileges from the native application privileges cache.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedPrivileges(
ClearCachedPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evicts application privileges from the native application privileges cache.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedPrivileges(
Function> fn) {
return clearCachedPrivileges(fn.apply(new ClearCachedPrivilegesRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_realms
/**
* Evicts users from the user cache. Can completely clear the cache or evict
* specific users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedRealms(ClearCachedRealmsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedRealmsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evicts users from the user cache. Can completely clear the cache or evict
* specific users.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedRealmsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedRealms(
Function> fn) {
return clearCachedRealms(fn.apply(new ClearCachedRealmsRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_roles
/**
* Evicts roles from the native role cache.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedRoles(ClearCachedRolesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedRolesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evicts roles from the native role cache.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedRolesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedRoles(
Function> fn) {
return clearCachedRoles(fn.apply(new ClearCachedRolesRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_service_tokens
/**
* Evicts tokens from the service account token caches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedServiceTokens(
ClearCachedServiceTokensRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedServiceTokensRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evicts tokens from the service account token caches.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedServiceTokensRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedServiceTokens(
Function> fn) {
return clearCachedServiceTokens(fn.apply(new ClearCachedServiceTokensRequest.Builder()).build());
}
// ----- Endpoint: security.create_api_key
/**
* Creates an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createApiKey(CreateApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture createApiKey(
Function> fn) {
return createApiKey(fn.apply(new CreateApiKeyRequest.Builder()).build());
}
/**
* Creates an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createApiKey() {
return this.transport.performRequestAsync(new CreateApiKeyRequest.Builder().build(),
CreateApiKeyRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.create_service_token
/**
* Creates a service accounts token for access without requiring basic
* authentication.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createServiceToken(CreateServiceTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateServiceTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates a service accounts token for access without requiring basic
* authentication.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateServiceTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture createServiceToken(
Function> fn) {
return createServiceToken(fn.apply(new CreateServiceTokenRequest.Builder()).build());
}
// ----- Endpoint: security.delete_privileges
/**
* Removes application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deletePrivileges(DeletePrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeletePrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Removes application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeletePrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deletePrivileges(
Function> fn) {
return deletePrivileges(fn.apply(new DeletePrivilegesRequest.Builder()).build());
}
// ----- Endpoint: security.delete_role
/**
* Removes roles in the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteRole(DeleteRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Removes roles in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteRole(
Function> fn) {
return deleteRole(fn.apply(new DeleteRoleRequest.Builder()).build());
}
// ----- Endpoint: security.delete_role_mapping
/**
* Removes role mappings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteRoleMapping(DeleteRoleMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRoleMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Removes role mappings.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteRoleMapping(
Function> fn) {
return deleteRoleMapping(fn.apply(new DeleteRoleMappingRequest.Builder()).build());
}
// ----- Endpoint: security.delete_service_token
/**
* Deletes a service account token.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteServiceToken(DeleteServiceTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteServiceTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Deletes a service account token.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteServiceTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteServiceToken(
Function> fn) {
return deleteServiceToken(fn.apply(new DeleteServiceTokenRequest.Builder()).build());
}
// ----- Endpoint: security.delete_user
/**
* Deletes users from the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteUser(DeleteUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Deletes users from the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteUser(
Function> fn) {
return deleteUser(fn.apply(new DeleteUserRequest.Builder()).build());
}
// ----- Endpoint: security.disable_user
/**
* Disables users in the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture disableUser(DisableUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DisableUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Disables users in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DisableUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture disableUser(
Function> fn) {
return disableUser(fn.apply(new DisableUserRequest.Builder()).build());
}
// ----- Endpoint: security.disable_user_profile
/**
* Disables a user profile so it's not visible in user profile searches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture disableUserProfile(DisableUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DisableUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Disables a user profile so it's not visible in user profile searches.
*
* @param fn
* a function that initializes a builder to create the
* {@link DisableUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture disableUserProfile(
Function> fn) {
return disableUserProfile(fn.apply(new DisableUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.enable_user
/**
* Enables users in the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enableUser(EnableUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EnableUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Enables users in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link EnableUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture enableUser(
Function> fn) {
return enableUser(fn.apply(new EnableUserRequest.Builder()).build());
}
// ----- Endpoint: security.enable_user_profile
/**
* Enables a user profile so it's visible in user profile searches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enableUserProfile(EnableUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EnableUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Enables a user profile so it's visible in user profile searches.
*
* @param fn
* a function that initializes a builder to create the
* {@link EnableUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture enableUserProfile(
Function> fn) {
return enableUserProfile(fn.apply(new EnableUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.enroll_kibana
/**
* Enables a Kibana instance to configure itself for communication with a
* secured Elasticsearch cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enrollKibana() {
return this.transport.performRequestAsync(EnrollKibanaRequest._INSTANCE, EnrollKibanaRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.enroll_node
/**
* Allows a new node to join an existing cluster with security features enabled.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enrollNode() {
return this.transport.performRequestAsync(EnrollNodeRequest._INSTANCE, EnrollNodeRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_api_key
/**
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getApiKey(GetApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getApiKey(
Function> fn) {
return getApiKey(fn.apply(new GetApiKeyRequest.Builder()).build());
}
/**
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getApiKey() {
return this.transport.performRequestAsync(new GetApiKeyRequest.Builder().build(), GetApiKeyRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_builtin_privileges
/**
* Retrieves the list of cluster privileges and index privileges that are
* available in this version of Elasticsearch.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getBuiltinPrivileges() {
return this.transport.performRequestAsync(GetBuiltinPrivilegesRequest._INSTANCE,
GetBuiltinPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_privileges
/**
* Retrieves application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getPrivileges(GetPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getPrivileges(
Function> fn) {
return getPrivileges(fn.apply(new GetPrivilegesRequest.Builder()).build());
}
/**
* Retrieves application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getPrivileges() {
return this.transport.performRequestAsync(new GetPrivilegesRequest.Builder().build(),
GetPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_role
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The get roles API cannot
* retrieve roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRole(GetRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The get roles API cannot
* retrieve roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getRole(
Function> fn) {
return getRole(fn.apply(new GetRoleRequest.Builder()).build());
}
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The get roles API cannot
* retrieve roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRole() {
return this.transport.performRequestAsync(new GetRoleRequest.Builder().build(), GetRoleRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_role_mapping
/**
* Retrieves role mappings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRoleMapping(GetRoleMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRoleMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves role mappings.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getRoleMapping(
Function> fn) {
return getRoleMapping(fn.apply(new GetRoleMappingRequest.Builder()).build());
}
/**
* Retrieves role mappings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRoleMapping() {
return this.transport.performRequestAsync(new GetRoleMappingRequest.Builder().build(),
GetRoleMappingRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_service_accounts
/**
* This API returns a list of service accounts that match the provided path
* parameter(s).
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getServiceAccounts(GetServiceAccountsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetServiceAccountsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* This API returns a list of service accounts that match the provided path
* parameter(s).
*
* @param fn
* a function that initializes a builder to create the
* {@link GetServiceAccountsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getServiceAccounts(
Function> fn) {
return getServiceAccounts(fn.apply(new GetServiceAccountsRequest.Builder()).build());
}
/**
* This API returns a list of service accounts that match the provided path
* parameter(s).
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getServiceAccounts() {
return this.transport.performRequestAsync(new GetServiceAccountsRequest.Builder().build(),
GetServiceAccountsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_service_credentials
/**
* Retrieves information of all service credentials for a service account.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getServiceCredentials(
GetServiceCredentialsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetServiceCredentialsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves information of all service credentials for a service account.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetServiceCredentialsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getServiceCredentials(
Function> fn) {
return getServiceCredentials(fn.apply(new GetServiceCredentialsRequest.Builder()).build());
}
// ----- Endpoint: security.get_token
/**
* Creates a bearer token for access without requiring basic authentication.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getToken(GetTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates a bearer token for access without requiring basic authentication.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getToken(
Function> fn) {
return getToken(fn.apply(new GetTokenRequest.Builder()).build());
}
/**
* Creates a bearer token for access without requiring basic authentication.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getToken() {
return this.transport.performRequestAsync(new GetTokenRequest.Builder().build(), GetTokenRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_user
/**
* Retrieves information about users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUser(GetUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves information about users in the native realm and built-in users.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getUser(
Function> fn) {
return getUser(fn.apply(new GetUserRequest.Builder()).build());
}
/**
* Retrieves information about users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUser() {
return this.transport.performRequestAsync(new GetUserRequest.Builder().build(), GetUserRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_user_privileges
/**
* Retrieves security privileges for the logged in user.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUserPrivileges(GetUserPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves security privileges for the logged in user.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getUserPrivileges(
Function> fn) {
return getUserPrivileges(fn.apply(new GetUserPrivilegesRequest.Builder()).build());
}
/**
* Retrieves security privileges for the logged in user.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUserPrivileges() {
return this.transport.performRequestAsync(new GetUserPrivilegesRequest.Builder().build(),
GetUserPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_user_profile
/**
* Retrieves a user's profile using the unique profile ID.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUserProfile(GetUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves a user's profile using the unique profile ID.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getUserProfile(
Function> fn) {
return getUserProfile(fn.apply(new GetUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.grant_api_key
/**
* Creates an API key on behalf of another user. This API is similar to Create
* API keys, however it creates the API key for a user that is different than
* the user that runs the API. The caller must have authentication credentials
* (either an access token, or a username and password) for the user on whose
* behalf the API key will be created. It is not possible to use this API to
* create an API key without that user’s credentials. The user, for whom the
* authentication credentials is provided, can optionally "run as"
* (impersonate) another user. In this case, the API key will be created on
* behalf of the impersonated user.
*
* This API is intended be used by applications that need to create and manage
* API keys for end users, but cannot guarantee that those users have permission
* to create API keys on their own behalf.
*
* A successful grant API key API call returns a JSON structure that contains
* the API key, its unique id, and its name. If applicable, it also returns
* expiration information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture grantApiKey(GrantApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GrantApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates an API key on behalf of another user. This API is similar to Create
* API keys, however it creates the API key for a user that is different than
* the user that runs the API. The caller must have authentication credentials
* (either an access token, or a username and password) for the user on whose
* behalf the API key will be created. It is not possible to use this API to
* create an API key without that user’s credentials. The user, for whom the
* authentication credentials is provided, can optionally "run as"
* (impersonate) another user. In this case, the API key will be created on
* behalf of the impersonated user.
*
* This API is intended be used by applications that need to create and manage
* API keys for end users, but cannot guarantee that those users have permission
* to create API keys on their own behalf.
*
* A successful grant API key API call returns a JSON structure that contains
* the API key, its unique id, and its name. If applicable, it also returns
* expiration information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* @param fn
* a function that initializes a builder to create the
* {@link GrantApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture grantApiKey(
Function> fn) {
return grantApiKey(fn.apply(new GrantApiKeyRequest.Builder()).build());
}
// ----- Endpoint: security.has_privileges
/**
* Determines whether the specified user has a specified list of privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture hasPrivileges(HasPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HasPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Determines whether the specified user has a specified list of privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link HasPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture hasPrivileges(
Function> fn) {
return hasPrivileges(fn.apply(new HasPrivilegesRequest.Builder()).build());
}
/**
* Determines whether the specified user has a specified list of privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture hasPrivileges() {
return this.transport.performRequestAsync(new HasPrivilegesRequest.Builder().build(),
HasPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.has_privileges_user_profile
/**
* Determines whether the users associated with the specified profile IDs have
* all the requested privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture hasPrivilegesUserProfile(
HasPrivilegesUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HasPrivilegesUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Determines whether the users associated with the specified profile IDs have
* all the requested privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link HasPrivilegesUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture hasPrivilegesUserProfile(
Function> fn) {
return hasPrivilegesUserProfile(fn.apply(new HasPrivilegesUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.invalidate_api_key
/**
* Invalidates one or more API keys. The manage_api_key
privilege
* allows deleting any API keys. The manage_own_api_key
only allows
* deleting API keys that are owned by the user. In addition, with the
* manage_own_api_key
privilege, an invalidation request must be
* issued in one of the three formats:
*
* - Set the parameter
owner=true
.
* - Or, set both
username
and realm_name
to match
* the user’s identity.
* - Or, if the request is issued by an API key, i.e. an API key invalidates
* itself, specify its ID in the
ids
field.
*
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture invalidateApiKey(InvalidateApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InvalidateApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Invalidates one or more API keys. The manage_api_key
privilege
* allows deleting any API keys. The manage_own_api_key
only allows
* deleting API keys that are owned by the user. In addition, with the
* manage_own_api_key
privilege, an invalidation request must be
* issued in one of the three formats:
*
* - Set the parameter
owner=true
.
* - Or, set both
username
and realm_name
to match
* the user’s identity.
* - Or, if the request is issued by an API key, i.e. an API key invalidates
* itself, specify its ID in the
ids
field.
*
*
* @param fn
* a function that initializes a builder to create the
* {@link InvalidateApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture invalidateApiKey(
Function> fn) {
return invalidateApiKey(fn.apply(new InvalidateApiKeyRequest.Builder()).build());
}
/**
* Invalidates one or more API keys. The manage_api_key
privilege
* allows deleting any API keys. The manage_own_api_key
only allows
* deleting API keys that are owned by the user. In addition, with the
* manage_own_api_key
privilege, an invalidation request must be
* issued in one of the three formats:
*
* - Set the parameter
owner=true
.
* - Or, set both
username
and realm_name
to match
* the user’s identity.
* - Or, if the request is issued by an API key, i.e. an API key invalidates
* itself, specify its ID in the
ids
field.
*
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture invalidateApiKey() {
return this.transport.performRequestAsync(new InvalidateApiKeyRequest.Builder().build(),
InvalidateApiKeyRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.invalidate_token
/**
* Invalidates one or more access tokens or refresh tokens.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture invalidateToken(InvalidateTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InvalidateTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Invalidates one or more access tokens or refresh tokens.
*
* @param fn
* a function that initializes a builder to create the
* {@link InvalidateTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture invalidateToken(
Function> fn) {
return invalidateToken(fn.apply(new InvalidateTokenRequest.Builder()).build());
}
/**
* Invalidates one or more access tokens or refresh tokens.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture invalidateToken() {
return this.transport.performRequestAsync(new InvalidateTokenRequest.Builder().build(),
InvalidateTokenRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.put_privileges
/**
* Adds or updates application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putPrivileges(PutPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Adds or updates application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putPrivileges(
Function> fn) {
return putPrivileges(fn.apply(new PutPrivilegesRequest.Builder()).build());
}
/**
* Adds or updates application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putPrivileges() {
return this.transport.performRequestAsync(new PutPrivilegesRequest.Builder().build(),
PutPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.put_role
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The create or update roles API
* cannot update roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putRole(PutRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The create or update roles API
* cannot update roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putRole(
Function> fn) {
return putRole(fn.apply(new PutRoleRequest.Builder()).build());
}
// ----- Endpoint: security.put_role_mapping
/**
* Creates and updates role mappings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putRoleMapping(PutRoleMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutRoleMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates and updates role mappings.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putRoleMapping(
Function> fn) {
return putRoleMapping(fn.apply(new PutRoleMappingRequest.Builder()).build());
}
// ----- Endpoint: security.put_user
/**
* Adds and updates users in the native realm. These users are commonly referred
* to as native users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putUser(PutUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Adds and updates users in the native realm. These users are commonly referred
* to as native users.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putUser(
Function> fn) {
return putUser(fn.apply(new PutUserRequest.Builder()).build());
}
// ----- Endpoint: security.query_api_keys
/**
* Retrieves information for API keys in a paginated manner. You can optionally
* filter the results with a query.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryApiKeys(QueryApiKeysRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryApiKeysRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves information for API keys in a paginated manner. You can optionally
* filter the results with a query.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryApiKeysRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture queryApiKeys(
Function> fn) {
return queryApiKeys(fn.apply(new QueryApiKeysRequest.Builder()).build());
}
/**
* Retrieves information for API keys in a paginated manner. You can optionally
* filter the results with a query.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryApiKeys() {
return this.transport.performRequestAsync(new QueryApiKeysRequest.Builder().build(),
QueryApiKeysRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.query_role
/**
* Retrieves roles in a paginated manner. You can optionally filter the results
* with a query.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryRole(QueryRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves roles in a paginated manner. You can optionally filter the results
* with a query.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture queryRole(
Function> fn) {
return queryRole(fn.apply(new QueryRoleRequest.Builder()).build());
}
/**
* Retrieves roles in a paginated manner. You can optionally filter the results
* with a query.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryRole() {
return this.transport.performRequestAsync(new QueryRoleRequest.Builder().build(), QueryRoleRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.query_user
/**
* Retrieves information for Users in a paginated manner. You can optionally
* filter the results with a query.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryUser(QueryUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves information for Users in a paginated manner. You can optionally
* filter the results with a query.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture queryUser(
Function> fn) {
return queryUser(fn.apply(new QueryUserRequest.Builder()).build());
}
/**
* Retrieves information for Users in a paginated manner. You can optionally
* filter the results with a query.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryUser() {
return this.transport.performRequestAsync(new QueryUserRequest.Builder().build(), QueryUserRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.saml_authenticate
/**
* Submits a SAML Response message to Elasticsearch for consumption.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture samlAuthenticate(SamlAuthenticateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlAuthenticateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Submits a SAML Response message to Elasticsearch for consumption.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlAuthenticateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture samlAuthenticate(
Function> fn) {
return samlAuthenticate(fn.apply(new SamlAuthenticateRequest.Builder()).build());
}
// ----- Endpoint: security.saml_complete_logout
/**
* Verifies the logout response sent from the SAML IdP.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture samlCompleteLogout(SamlCompleteLogoutRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) SamlCompleteLogoutRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Verifies the logout response sent from the SAML IdP.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlCompleteLogoutRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture samlCompleteLogout(
Function> fn) {
return samlCompleteLogout(fn.apply(new SamlCompleteLogoutRequest.Builder()).build());
}
// ----- Endpoint: security.saml_invalidate
/**
* Submits a SAML LogoutRequest message to Elasticsearch for consumption.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture samlInvalidate(SamlInvalidateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlInvalidateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Submits a SAML LogoutRequest message to Elasticsearch for consumption.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlInvalidateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture samlInvalidate(
Function> fn) {
return samlInvalidate(fn.apply(new SamlInvalidateRequest.Builder()).build());
}
// ----- Endpoint: security.saml_logout
/**
* Submits a request to invalidate an access token and refresh token.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture samlLogout(SamlLogoutRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint