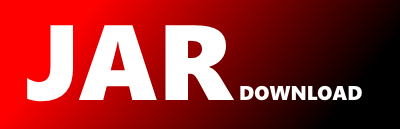
co.elastic.clients.elasticsearch.transform.ElasticsearchTransformAsyncClient Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.transform;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.EndpointWithResponseMapperAttr;
import co.elastic.clients.util.ObjectBuilder;
import java.lang.reflect.Type;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the transform namespace.
*/
public class ElasticsearchTransformAsyncClient
extends
ApiClient {
public ElasticsearchTransformAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchTransformAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchTransformAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchTransformAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: transform.delete_transform
/**
* Deletes a transform.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteTransform(DeleteTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Deletes a transform.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteTransform(
Function> fn) {
return deleteTransform(fn.apply(new DeleteTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.get_transform
/**
* Retrieves configuration information for transforms.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTransform(GetTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves configuration information for transforms.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getTransform(
Function> fn) {
return getTransform(fn.apply(new GetTransformRequest.Builder()).build());
}
/**
* Retrieves configuration information for transforms.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTransform() {
return this.transport.performRequestAsync(new GetTransformRequest.Builder().build(),
GetTransformRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: transform.get_transform_stats
/**
* Retrieves usage information for transforms.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTransformStats(GetTransformStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTransformStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves usage information for transforms.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTransformStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getTransformStats(
Function> fn) {
return getTransformStats(fn.apply(new GetTransformStatsRequest.Builder()).build());
}
// ----- Endpoint: transform.preview_transform
/**
* Previews a transform.
*
* It returns a maximum of 100 results. The calculations are based on all the
* current data in the source index. It also generates a list of mappings and
* settings for the destination index. These values are determined based on the
* field types of the source index and the transform aggregations.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture> previewTransform(
PreviewTransformRequest request, Class tTransformClass) {
@SuppressWarnings("unchecked")
JsonEndpoint, ErrorResponse> endpoint = (JsonEndpoint, ErrorResponse>) PreviewTransformRequest._ENDPOINT;
endpoint = new EndpointWithResponseMapperAttr<>(endpoint,
"co.elastic.clients:Deserializer:transform.preview_transform.Response.TTransform",
getDeserializer(tTransformClass));
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Previews a transform.
*
* It returns a maximum of 100 results. The calculations are based on all the
* current data in the source index. It also generates a list of mappings and
* settings for the destination index. These values are determined based on the
* field types of the source index and the transform aggregations.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture> previewTransform(
Function> fn,
Class tTransformClass) {
return previewTransform(fn.apply(new PreviewTransformRequest.Builder()).build(), tTransformClass);
}
/**
* Previews a transform.
*
* It returns a maximum of 100 results. The calculations are based on all the
* current data in the source index. It also generates a list of mappings and
* settings for the destination index. These values are determined based on the
* field types of the source index and the transform aggregations.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture> previewTransform(
PreviewTransformRequest request, Type tTransformType) {
@SuppressWarnings("unchecked")
JsonEndpoint, ErrorResponse> endpoint = (JsonEndpoint, ErrorResponse>) PreviewTransformRequest._ENDPOINT;
endpoint = new EndpointWithResponseMapperAttr<>(endpoint,
"co.elastic.clients:Deserializer:transform.preview_transform.Response.TTransform",
getDeserializer(tTransformType));
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Previews a transform.
*
* It returns a maximum of 100 results. The calculations are based on all the
* current data in the source index. It also generates a list of mappings and
* settings for the destination index. These values are determined based on the
* field types of the source index and the transform aggregations.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture> previewTransform(
Function> fn, Type tTransformType) {
return previewTransform(fn.apply(new PreviewTransformRequest.Builder()).build(), tTransformType);
}
// ----- Endpoint: transform.put_transform
/**
* Creates a transform.
*
* A transform copies data from source indices, transforms it, and persists it
* into an entity-centric destination index. You can also think of the
* destination index as a two-dimensional tabular data structure (known as a
* data frame). The ID for each document in the data frame is generated from a
* hash of the entity, so there is a unique row per entity.
*
* You must choose either the latest or pivot method for your transform; you
* cannot use both in a single transform. If you choose to use the pivot method
* for your transform, the entities are defined by the set of
* group_by
fields in the pivot object. If you choose to use the
* latest method, the entities are defined by the unique_key
field
* values in the latest object.
*
* You must have create_index
, index
, and
* read
privileges on the destination index and read
* and view_index_metadata
privileges on the source indices. When
* Elasticsearch security features are enabled, the transform remembers which
* roles the user that created it had at the time of creation and uses those
* same roles. If those roles do not have the required privileges on the source
* and destination indices, the transform fails when it attempts unauthorized
* operations.
*
* NOTE: You must use Kibana or this API to create a transform. Do not add a
* transform directly into any .transform-internal*
indices using
* the Elasticsearch index API. If Elasticsearch security features are enabled,
* do not give users any privileges on .transform-internal*
* indices. If you used transforms prior to 7.5, also do not give users any
* privileges on .data-frame-internal*
indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putTransform(PutTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates a transform.
*
* A transform copies data from source indices, transforms it, and persists it
* into an entity-centric destination index. You can also think of the
* destination index as a two-dimensional tabular data structure (known as a
* data frame). The ID for each document in the data frame is generated from a
* hash of the entity, so there is a unique row per entity.
*
* You must choose either the latest or pivot method for your transform; you
* cannot use both in a single transform. If you choose to use the pivot method
* for your transform, the entities are defined by the set of
* group_by
fields in the pivot object. If you choose to use the
* latest method, the entities are defined by the unique_key
field
* values in the latest object.
*
* You must have create_index
, index
, and
* read
privileges on the destination index and read
* and view_index_metadata
privileges on the source indices. When
* Elasticsearch security features are enabled, the transform remembers which
* roles the user that created it had at the time of creation and uses those
* same roles. If those roles do not have the required privileges on the source
* and destination indices, the transform fails when it attempts unauthorized
* operations.
*
* NOTE: You must use Kibana or this API to create a transform. Do not add a
* transform directly into any .transform-internal*
indices using
* the Elasticsearch index API. If Elasticsearch security features are enabled,
* do not give users any privileges on .transform-internal*
* indices. If you used transforms prior to 7.5, also do not give users any
* privileges on .data-frame-internal*
indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putTransform(
Function> fn) {
return putTransform(fn.apply(new PutTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.reset_transform
/**
* Resets a transform. Before you can reset it, you must stop it; alternatively,
* use the force
query parameter. If the destination index was
* created by the transform, it is deleted.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture resetTransform(ResetTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ResetTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Resets a transform. Before you can reset it, you must stop it; alternatively,
* use the force
query parameter. If the destination index was
* created by the transform, it is deleted.
*
* @param fn
* a function that initializes a builder to create the
* {@link ResetTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture resetTransform(
Function> fn) {
return resetTransform(fn.apply(new ResetTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.schedule_now_transform
/**
* Schedules now a transform.
*
* If you _schedule_now a transform, it will process the new data instantly,
* without waiting for the configured frequency interval. After _schedule_now
* API is called, the transform will be processed again at now + frequency
* unless _schedule_now API is called again in the meantime.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture scheduleNowTransform(ScheduleNowTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ScheduleNowTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Schedules now a transform.
*
* If you _schedule_now a transform, it will process the new data instantly,
* without waiting for the configured frequency interval. After _schedule_now
* API is called, the transform will be processed again at now + frequency
* unless _schedule_now API is called again in the meantime.
*
* @param fn
* a function that initializes a builder to create the
* {@link ScheduleNowTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture scheduleNowTransform(
Function> fn) {
return scheduleNowTransform(fn.apply(new ScheduleNowTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.start_transform
/**
* Starts a transform.
*
* When you start a transform, it creates the destination index if it does not
* already exist. The number_of_shards
is set to 1
and
* the auto_expand_replicas
is set to 0-1
. If it is a
* pivot transform, it deduces the mapping definitions for the destination index
* from the source indices and the transform aggregations. If fields in the
* destination index are derived from scripts (as in the case of
* scripted_metric
or bucket_script
aggregations), the
* transform uses dynamic mappings unless an index template exists. If it is a
* latest transform, it does not deduce mapping definitions; it uses dynamic
* mappings. To use explicit mappings, create the destination index before you
* start the transform. Alternatively, you can create an index template, though
* it does not affect the deduced mappings in a pivot transform.
*
* When the transform starts, a series of validations occur to ensure its
* success. If you deferred validation when you created the transform, they
* occur when you start the transform—with the exception of privilege checks.
* When Elasticsearch security features are enabled, the transform remembers
* which roles the user that created it had at the time of creation and uses
* those same roles. If those roles do not have the required privileges on the
* source and destination indices, the transform fails when it attempts
* unauthorized operations.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture startTransform(StartTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Starts a transform.
*
* When you start a transform, it creates the destination index if it does not
* already exist. The number_of_shards
is set to 1
and
* the auto_expand_replicas
is set to 0-1
. If it is a
* pivot transform, it deduces the mapping definitions for the destination index
* from the source indices and the transform aggregations. If fields in the
* destination index are derived from scripts (as in the case of
* scripted_metric
or bucket_script
aggregations), the
* transform uses dynamic mappings unless an index template exists. If it is a
* latest transform, it does not deduce mapping definitions; it uses dynamic
* mappings. To use explicit mappings, create the destination index before you
* start the transform. Alternatively, you can create an index template, though
* it does not affect the deduced mappings in a pivot transform.
*
* When the transform starts, a series of validations occur to ensure its
* success. If you deferred validation when you created the transform, they
* occur when you start the transform—with the exception of privilege checks.
* When Elasticsearch security features are enabled, the transform remembers
* which roles the user that created it had at the time of creation and uses
* those same roles. If those roles do not have the required privileges on the
* source and destination indices, the transform fails when it attempts
* unauthorized operations.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture startTransform(
Function> fn) {
return startTransform(fn.apply(new StartTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.stop_transform
/**
* Stops one or more transforms.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture stopTransform(StopTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Stops one or more transforms.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture stopTransform(
Function> fn) {
return stopTransform(fn.apply(new StopTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.update_transform
/**
* Updates certain properties of a transform.
*
* All updated properties except description
do not take effect
* until after the transform starts the next checkpoint, thus there is data
* consistency in each checkpoint. To use this API, you must have
* read
and view_index_metadata
privileges for the
* source indices. You must also have index
and read
* privileges for the destination index. When Elasticsearch security features
* are enabled, the transform remembers which roles the user who updated it had
* at the time of update and runs with those privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateTransform(UpdateTransformRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateTransformRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Updates certain properties of a transform.
*
* All updated properties except description
do not take effect
* until after the transform starts the next checkpoint, thus there is data
* consistency in each checkpoint. To use this API, you must have
* read
and view_index_metadata
privileges for the
* source indices. You must also have index
and read
* privileges for the destination index. When Elasticsearch security features
* are enabled, the transform remembers which roles the user who updated it had
* at the time of update and runs with those privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateTransformRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateTransform(
Function> fn) {
return updateTransform(fn.apply(new UpdateTransformRequest.Builder()).build());
}
// ----- Endpoint: transform.upgrade_transforms
/**
* Upgrades all transforms. This API identifies transforms that have a legacy
* configuration format and upgrades them to the latest version. It also cleans
* up the internal data structures that store the transform state and
* checkpoints. The upgrade does not affect the source and destination indices.
* The upgrade also does not affect the roles that transforms use when
* Elasticsearch security features are enabled; the role used to read source
* data and write to the destination index remains unchanged.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture upgradeTransforms(UpgradeTransformsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpgradeTransformsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Upgrades all transforms. This API identifies transforms that have a legacy
* configuration format and upgrades them to the latest version. It also cleans
* up the internal data structures that store the transform state and
* checkpoints. The upgrade does not affect the source and destination indices.
* The upgrade also does not affect the roles that transforms use when
* Elasticsearch security features are enabled; the role used to read source
* data and write to the destination index remains unchanged.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpgradeTransformsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture upgradeTransforms(
Function> fn) {
return upgradeTransforms(fn.apply(new UpgradeTransformsRequest.Builder()).build());
}
/**
* Upgrades all transforms. This API identifies transforms that have a legacy
* configuration format and upgrades them to the latest version. It also cleans
* up the internal data structures that store the transform state and
* checkpoints. The upgrade does not affect the source and destination indices.
* The upgrade also does not affect the roles that transforms use when
* Elasticsearch security features are enabled; the role used to read source
* data and write to the destination index remains unchanged.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture upgradeTransforms() {
return this.transport.performRequestAsync(new UpgradeTransformsRequest.Builder().build(),
UpgradeTransformsRequest._ENDPOINT, this.transportOptions);
}
}