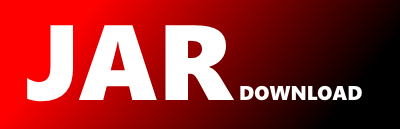
co.elastic.clients.elasticsearch.cat.ElasticsearchCatAsyncClient Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.cat;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the cat namespace.
*/
public class ElasticsearchCatAsyncClient extends ApiClient {
public ElasticsearchCatAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchCatAsyncClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchCatAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchCatAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: cat.aliases
/**
* Get aliases. Retrieves the cluster’s index aliases, including filter and
* routing information. The API does not return data stream aliases.
*
* CAT APIs are only intended for human consumption using the command line or
* the Kibana console. They are not intended for use by applications. For
* application consumption, use the aliases API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture aliases(AliasesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AliasesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get aliases. Retrieves the cluster’s index aliases, including filter and
* routing information. The API does not return data stream aliases.
*
* CAT APIs are only intended for human consumption using the command line or
* the Kibana console. They are not intended for use by applications. For
* application consumption, use the aliases API.
*
* @param fn
* a function that initializes a builder to create the
* {@link AliasesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture aliases(
Function> fn) {
return aliases(fn.apply(new AliasesRequest.Builder()).build());
}
/**
* Get aliases. Retrieves the cluster’s index aliases, including filter and
* routing information. The API does not return data stream aliases.
*
* CAT APIs are only intended for human consumption using the command line or
* the Kibana console. They are not intended for use by applications. For
* application consumption, use the aliases API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture aliases() {
return this.transport.performRequestAsync(new AliasesRequest.Builder().build(), AliasesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.allocation
/**
* Provides a snapshot of the number of shards allocated to each data node and
* their disk space. IMPORTANT: cat APIs are only intended for human consumption
* using the command line or Kibana console. They are not intended for use by
* applications.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture allocation(AllocationRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AllocationRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Provides a snapshot of the number of shards allocated to each data node and
* their disk space. IMPORTANT: cat APIs are only intended for human consumption
* using the command line or Kibana console. They are not intended for use by
* applications.
*
* @param fn
* a function that initializes a builder to create the
* {@link AllocationRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture allocation(
Function> fn) {
return allocation(fn.apply(new AllocationRequest.Builder()).build());
}
/**
* Provides a snapshot of the number of shards allocated to each data node and
* their disk space. IMPORTANT: cat APIs are only intended for human consumption
* using the command line or Kibana console. They are not intended for use by
* applications.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture allocation() {
return this.transport.performRequestAsync(new AllocationRequest.Builder().build(), AllocationRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.component_templates
/**
* Get component templates. Returns information about component templates in a
* cluster. Component templates are building blocks for constructing index
* templates that specify index mappings, settings, and aliases.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the get component template API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture componentTemplates(ComponentTemplatesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ComponentTemplatesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get component templates. Returns information about component templates in a
* cluster. Component templates are building blocks for constructing index
* templates that specify index mappings, settings, and aliases.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the get component template API.
*
* @param fn
* a function that initializes a builder to create the
* {@link ComponentTemplatesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture componentTemplates(
Function> fn) {
return componentTemplates(fn.apply(new ComponentTemplatesRequest.Builder()).build());
}
/**
* Get component templates. Returns information about component templates in a
* cluster. Component templates are building blocks for constructing index
* templates that specify index mappings, settings, and aliases.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the get component template API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture componentTemplates() {
return this.transport.performRequestAsync(new ComponentTemplatesRequest.Builder().build(),
ComponentTemplatesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.count
/**
* Get a document count. Provides quick access to a document count for a data
* stream, an index, or an entire cluster. The document count only includes live
* documents, not deleted documents which have not yet been removed by the merge
* process.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the count API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture count(CountRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CountRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get a document count. Provides quick access to a document count for a data
* stream, an index, or an entire cluster. The document count only includes live
* documents, not deleted documents which have not yet been removed by the merge
* process.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the count API.
*
* @param fn
* a function that initializes a builder to create the
* {@link CountRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture count(
Function> fn) {
return count(fn.apply(new CountRequest.Builder()).build());
}
/**
* Get a document count. Provides quick access to a document count for a data
* stream, an index, or an entire cluster. The document count only includes live
* documents, not deleted documents which have not yet been removed by the merge
* process.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the count API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture count() {
return this.transport.performRequestAsync(new CountRequest.Builder().build(), CountRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.fielddata
/**
* Returns the amount of heap memory currently used by the field data cache on
* every data node in the cluster. IMPORTANT: cat APIs are only intended for
* human consumption using the command line or Kibana console. They are not
* intended for use by applications. For application consumption, use the nodes
* stats API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture fielddata(FielddataRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FielddataRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns the amount of heap memory currently used by the field data cache on
* every data node in the cluster. IMPORTANT: cat APIs are only intended for
* human consumption using the command line or Kibana console. They are not
* intended for use by applications. For application consumption, use the nodes
* stats API.
*
* @param fn
* a function that initializes a builder to create the
* {@link FielddataRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture fielddata(
Function> fn) {
return fielddata(fn.apply(new FielddataRequest.Builder()).build());
}
/**
* Returns the amount of heap memory currently used by the field data cache on
* every data node in the cluster. IMPORTANT: cat APIs are only intended for
* human consumption using the command line or Kibana console. They are not
* intended for use by applications. For application consumption, use the nodes
* stats API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture fielddata() {
return this.transport.performRequestAsync(new FielddataRequest.Builder().build(), FielddataRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.health
/**
* Returns the health status of a cluster, similar to the cluster health API.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the cluster health API. This API is often used
* to check malfunctioning clusters. To help you track cluster health alongside
* log files and alerting systems, the API returns timestamps in two formats:
* HH:MM:SS
, which is human-readable but includes no date
* information; Unix epoch time
, which is machine-sortable and
* includes date information. The latter format is useful for cluster recoveries
* that take multiple days. You can use the cat health API to verify cluster
* health across multiple nodes. You also can use the API to track the recovery
* of a large cluster over a longer period of time.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture health(HealthRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HealthRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns the health status of a cluster, similar to the cluster health API.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the cluster health API. This API is often used
* to check malfunctioning clusters. To help you track cluster health alongside
* log files and alerting systems, the API returns timestamps in two formats:
* HH:MM:SS
, which is human-readable but includes no date
* information; Unix epoch time
, which is machine-sortable and
* includes date information. The latter format is useful for cluster recoveries
* that take multiple days. You can use the cat health API to verify cluster
* health across multiple nodes. You also can use the API to track the recovery
* of a large cluster over a longer period of time.
*
* @param fn
* a function that initializes a builder to create the
* {@link HealthRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture health(
Function> fn) {
return health(fn.apply(new HealthRequest.Builder()).build());
}
/**
* Returns the health status of a cluster, similar to the cluster health API.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the cluster health API. This API is often used
* to check malfunctioning clusters. To help you track cluster health alongside
* log files and alerting systems, the API returns timestamps in two formats:
* HH:MM:SS
, which is human-readable but includes no date
* information; Unix epoch time
, which is machine-sortable and
* includes date information. The latter format is useful for cluster recoveries
* that take multiple days. You can use the cat health API to verify cluster
* health across multiple nodes. You also can use the API to track the recovery
* of a large cluster over a longer period of time.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture health() {
return this.transport.performRequestAsync(new HealthRequest.Builder().build(), HealthRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.help
/**
* Get CAT help. Returns help for the CAT APIs.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture help() {
return this.transport.performRequestAsync(HelpRequest._INSTANCE, HelpRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.indices
/**
* Get index information. Returns high-level information about indices in a
* cluster, including backing indices for data streams.
*
* Use this request to get the following information for each index in a
* cluster:
*
* - shard count
* - document count
* - deleted document count
* - primary store size
* - total store size of all shards, including shard replicas
*
*
* These metrics are retrieved directly from Lucene, which Elasticsearch uses
* internally to power indexing and search. As a result, all document counts
* include hidden nested documents. To get an accurate count of Elasticsearch
* documents, use the cat count or count APIs.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use an index endpoint.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture indices(IndicesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) IndicesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get index information. Returns high-level information about indices in a
* cluster, including backing indices for data streams.
*
* Use this request to get the following information for each index in a
* cluster:
*
* - shard count
* - document count
* - deleted document count
* - primary store size
* - total store size of all shards, including shard replicas
*
*
* These metrics are retrieved directly from Lucene, which Elasticsearch uses
* internally to power indexing and search. As a result, all document counts
* include hidden nested documents. To get an accurate count of Elasticsearch
* documents, use the cat count or count APIs.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use an index endpoint.
*
* @param fn
* a function that initializes a builder to create the
* {@link IndicesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture indices(
Function> fn) {
return indices(fn.apply(new IndicesRequest.Builder()).build());
}
/**
* Get index information. Returns high-level information about indices in a
* cluster, including backing indices for data streams.
*
* Use this request to get the following information for each index in a
* cluster:
*
* - shard count
* - document count
* - deleted document count
* - primary store size
* - total store size of all shards, including shard replicas
*
*
* These metrics are retrieved directly from Lucene, which Elasticsearch uses
* internally to power indexing and search. As a result, all document counts
* include hidden nested documents. To get an accurate count of Elasticsearch
* documents, use the cat count or count APIs.
*
* CAT APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use an index endpoint.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture indices() {
return this.transport.performRequestAsync(new IndicesRequest.Builder().build(), IndicesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.master
/**
* Returns information about the master node, including the ID, bound IP
* address, and name. IMPORTANT: cat APIs are only intended for human
* consumption using the command line or Kibana console. They are not intended
* for use by applications. For application consumption, use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture master() {
return this.transport.performRequestAsync(MasterRequest._INSTANCE, MasterRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.ml_data_frame_analytics
/**
* Get data frame analytics jobs. Returns configuration and usage information
* about data frame analytics jobs.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get data frame analytics jobs statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDataFrameAnalytics(MlDataFrameAnalyticsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get data frame analytics jobs. Returns configuration and usage information
* about data frame analytics jobs.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get data frame analytics jobs statistics API.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlDataFrameAnalytics(
Function> fn) {
return mlDataFrameAnalytics(fn.apply(new MlDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Get data frame analytics jobs. Returns configuration and usage information
* about data frame analytics jobs.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get data frame analytics jobs statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDataFrameAnalytics() {
return this.transport.performRequestAsync(new MlDataFrameAnalyticsRequest.Builder().build(),
MlDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.ml_datafeeds
/**
* Get datafeeds. Returns configuration and usage information about datafeeds.
* This API returns a maximum of 10,000 datafeeds. If the Elasticsearch security
* features are enabled, you must have monitor_ml
,
* monitor
, manage_ml
, or manage
cluster
* privileges to use this API.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get datafeed statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDatafeeds(MlDatafeedsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlDatafeedsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get datafeeds. Returns configuration and usage information about datafeeds.
* This API returns a maximum of 10,000 datafeeds. If the Elasticsearch security
* features are enabled, you must have monitor_ml
,
* monitor
, manage_ml
, or manage
cluster
* privileges to use this API.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get datafeed statistics API.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlDatafeedsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlDatafeeds(
Function> fn) {
return mlDatafeeds(fn.apply(new MlDatafeedsRequest.Builder()).build());
}
/**
* Get datafeeds. Returns configuration and usage information about datafeeds.
* This API returns a maximum of 10,000 datafeeds. If the Elasticsearch security
* features are enabled, you must have monitor_ml
,
* monitor
, manage_ml
, or manage
cluster
* privileges to use this API.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get datafeed statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDatafeeds() {
return this.transport.performRequestAsync(new MlDatafeedsRequest.Builder().build(),
MlDatafeedsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.ml_jobs
/**
* Get anomaly detection jobs. Returns configuration and usage information for
* anomaly detection jobs. This API returns a maximum of 10,000 jobs. If the
* Elasticsearch security features are enabled, you must have
* monitor_ml
, monitor
, manage_ml
, or
* manage
cluster privileges to use this API.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get anomaly detection job statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlJobs(MlJobsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlJobsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection jobs. Returns configuration and usage information for
* anomaly detection jobs. This API returns a maximum of 10,000 jobs. If the
* Elasticsearch security features are enabled, you must have
* monitor_ml
, monitor
, manage_ml
, or
* manage
cluster privileges to use this API.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get anomaly detection job statistics API.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlJobsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlJobs(
Function> fn) {
return mlJobs(fn.apply(new MlJobsRequest.Builder()).build());
}
/**
* Get anomaly detection jobs. Returns configuration and usage information for
* anomaly detection jobs. This API returns a maximum of 10,000 jobs. If the
* Elasticsearch security features are enabled, you must have
* monitor_ml
, monitor
, manage_ml
, or
* manage
cluster privileges to use this API.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get anomaly detection job statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlJobs() {
return this.transport.performRequestAsync(new MlJobsRequest.Builder().build(), MlJobsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.ml_trained_models
/**
* Get trained models. Returns configuration and usage information about
* inference trained models.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get trained models statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlTrainedModels(MlTrainedModelsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlTrainedModelsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get trained models. Returns configuration and usage information about
* inference trained models.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get trained models statistics API.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlTrainedModelsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlTrainedModels(
Function> fn) {
return mlTrainedModels(fn.apply(new MlTrainedModelsRequest.Builder()).build());
}
/**
* Get trained models. Returns configuration and usage information about
* inference trained models.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get trained models statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlTrainedModels() {
return this.transport.performRequestAsync(new MlTrainedModelsRequest.Builder().build(),
MlTrainedModelsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.nodeattrs
/**
* Returns information about custom node attributes. IMPORTANT: cat APIs are
* only intended for human consumption using the command line or Kibana console.
* They are not intended for use by applications. For application consumption,
* use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture nodeattrs() {
return this.transport.performRequestAsync(NodeattrsRequest._INSTANCE, NodeattrsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.nodes
/**
* Returns information about the nodes in a cluster. IMPORTANT: cat APIs are
* only intended for human consumption using the command line or Kibana console.
* They are not intended for use by applications. For application consumption,
* use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture nodes(NodesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) NodesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about the nodes in a cluster. IMPORTANT: cat APIs are
* only intended for human consumption using the command line or Kibana console.
* They are not intended for use by applications. For application consumption,
* use the nodes info API.
*
* @param fn
* a function that initializes a builder to create the
* {@link NodesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture nodes(
Function> fn) {
return nodes(fn.apply(new NodesRequest.Builder()).build());
}
/**
* Returns information about the nodes in a cluster. IMPORTANT: cat APIs are
* only intended for human consumption using the command line or Kibana console.
* They are not intended for use by applications. For application consumption,
* use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture nodes() {
return this.transport.performRequestAsync(new NodesRequest.Builder().build(), NodesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.pending_tasks
/**
* Returns cluster-level changes that have not yet been executed. IMPORTANT: cat
* APIs are only intended for human consumption using the command line or Kibana
* console. They are not intended for use by applications. For application
* consumption, use the pending cluster tasks API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture pendingTasks() {
return this.transport.performRequestAsync(PendingTasksRequest._INSTANCE, PendingTasksRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.plugins
/**
* Returns a list of plugins running on each node of a cluster. IMPORTANT: cat
* APIs are only intended for human consumption using the command line or Kibana
* console. They are not intended for use by applications. For application
* consumption, use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture plugins() {
return this.transport.performRequestAsync(PluginsRequest._INSTANCE, PluginsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.recovery
/**
* Returns information about ongoing and completed shard recoveries. Shard
* recovery is the process of initializing a shard copy, such as restoring a
* primary shard from a snapshot or syncing a replica shard from a primary
* shard. When a shard recovery completes, the recovered shard is available for
* search and indexing. For data streams, the API returns information about the
* stream’s backing indices. IMPORTANT: cat APIs are only intended for human
* consumption using the command line or Kibana console. They are not intended
* for use by applications. For application consumption, use the index recovery
* API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture recovery(RecoveryRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RecoveryRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about ongoing and completed shard recoveries. Shard
* recovery is the process of initializing a shard copy, such as restoring a
* primary shard from a snapshot or syncing a replica shard from a primary
* shard. When a shard recovery completes, the recovered shard is available for
* search and indexing. For data streams, the API returns information about the
* stream’s backing indices. IMPORTANT: cat APIs are only intended for human
* consumption using the command line or Kibana console. They are not intended
* for use by applications. For application consumption, use the index recovery
* API.
*
* @param fn
* a function that initializes a builder to create the
* {@link RecoveryRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture recovery(
Function> fn) {
return recovery(fn.apply(new RecoveryRequest.Builder()).build());
}
/**
* Returns information about ongoing and completed shard recoveries. Shard
* recovery is the process of initializing a shard copy, such as restoring a
* primary shard from a snapshot or syncing a replica shard from a primary
* shard. When a shard recovery completes, the recovered shard is available for
* search and indexing. For data streams, the API returns information about the
* stream’s backing indices. IMPORTANT: cat APIs are only intended for human
* consumption using the command line or Kibana console. They are not intended
* for use by applications. For application consumption, use the index recovery
* API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture recovery() {
return this.transport.performRequestAsync(new RecoveryRequest.Builder().build(), RecoveryRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.repositories
/**
* Returns the snapshot repositories for a cluster. IMPORTANT: cat APIs are only
* intended for human consumption using the command line or Kibana console. They
* are not intended for use by applications. For application consumption, use
* the get snapshot repository API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture repositories() {
return this.transport.performRequestAsync(RepositoriesRequest._INSTANCE, RepositoriesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.segments
/**
* Returns low-level information about the Lucene segments in index shards. For
* data streams, the API returns information about the backing indices.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the index segments API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture segments(SegmentsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SegmentsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns low-level information about the Lucene segments in index shards. For
* data streams, the API returns information about the backing indices.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the index segments API.
*
* @param fn
* a function that initializes a builder to create the
* {@link SegmentsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture segments(
Function> fn) {
return segments(fn.apply(new SegmentsRequest.Builder()).build());
}
/**
* Returns low-level information about the Lucene segments in index shards. For
* data streams, the API returns information about the backing indices.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the index segments API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture segments() {
return this.transport.performRequestAsync(new SegmentsRequest.Builder().build(), SegmentsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.shards
/**
* Returns information about the shards in a cluster. For data streams, the API
* returns information about the backing indices. IMPORTANT: cat APIs are only
* intended for human consumption using the command line or Kibana console. They
* are not intended for use by applications.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture shards(ShardsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ShardsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about the shards in a cluster. For data streams, the API
* returns information about the backing indices. IMPORTANT: cat APIs are only
* intended for human consumption using the command line or Kibana console. They
* are not intended for use by applications.
*
* @param fn
* a function that initializes a builder to create the
* {@link ShardsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture shards(
Function> fn) {
return shards(fn.apply(new ShardsRequest.Builder()).build());
}
/**
* Returns information about the shards in a cluster. For data streams, the API
* returns information about the backing indices. IMPORTANT: cat APIs are only
* intended for human consumption using the command line or Kibana console. They
* are not intended for use by applications.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture shards() {
return this.transport.performRequestAsync(new ShardsRequest.Builder().build(), ShardsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.snapshots
/**
* Returns information about the snapshots stored in one or more repositories. A
* snapshot is a backup of an index or running Elasticsearch cluster. IMPORTANT:
* cat APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the get snapshot API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture snapshots(SnapshotsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SnapshotsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about the snapshots stored in one or more repositories. A
* snapshot is a backup of an index or running Elasticsearch cluster. IMPORTANT:
* cat APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the get snapshot API.
*
* @param fn
* a function that initializes a builder to create the
* {@link SnapshotsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture snapshots(
Function> fn) {
return snapshots(fn.apply(new SnapshotsRequest.Builder()).build());
}
/**
* Returns information about the snapshots stored in one or more repositories. A
* snapshot is a backup of an index or running Elasticsearch cluster. IMPORTANT:
* cat APIs are only intended for human consumption using the command line or
* Kibana console. They are not intended for use by applications. For
* application consumption, use the get snapshot API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture snapshots() {
return this.transport.performRequestAsync(new SnapshotsRequest.Builder().build(), SnapshotsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.tasks
/**
* Returns information about tasks currently executing in the cluster.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the task management API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture tasks(TasksRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) TasksRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about tasks currently executing in the cluster.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the task management API.
*
* @param fn
* a function that initializes a builder to create the
* {@link TasksRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture tasks(
Function> fn) {
return tasks(fn.apply(new TasksRequest.Builder()).build());
}
/**
* Returns information about tasks currently executing in the cluster.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the task management API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture tasks() {
return this.transport.performRequestAsync(new TasksRequest.Builder().build(), TasksRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.templates
/**
* Returns information about index templates in a cluster. You can use index
* templates to apply index settings and field mappings to new indices at
* creation. IMPORTANT: cat APIs are only intended for human consumption using
* the command line or Kibana console. They are not intended for use by
* applications. For application consumption, use the get index template API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture templates(TemplatesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) TemplatesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about index templates in a cluster. You can use index
* templates to apply index settings and field mappings to new indices at
* creation. IMPORTANT: cat APIs are only intended for human consumption using
* the command line or Kibana console. They are not intended for use by
* applications. For application consumption, use the get index template API.
*
* @param fn
* a function that initializes a builder to create the
* {@link TemplatesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture templates(
Function> fn) {
return templates(fn.apply(new TemplatesRequest.Builder()).build());
}
/**
* Returns information about index templates in a cluster. You can use index
* templates to apply index settings and field mappings to new indices at
* creation. IMPORTANT: cat APIs are only intended for human consumption using
* the command line or Kibana console. They are not intended for use by
* applications. For application consumption, use the get index template API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture templates() {
return this.transport.performRequestAsync(new TemplatesRequest.Builder().build(), TemplatesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.thread_pool
/**
* Returns thread pool statistics for each node in a cluster. Returned
* information includes all built-in thread pools and custom thread pools.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture threadPool(ThreadPoolRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ThreadPoolRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns thread pool statistics for each node in a cluster. Returned
* information includes all built-in thread pools and custom thread pools.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the nodes info API.
*
* @param fn
* a function that initializes a builder to create the
* {@link ThreadPoolRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture threadPool(
Function> fn) {
return threadPool(fn.apply(new ThreadPoolRequest.Builder()).build());
}
/**
* Returns thread pool statistics for each node in a cluster. Returned
* information includes all built-in thread pools and custom thread pools.
* IMPORTANT: cat APIs are only intended for human consumption using the command
* line or Kibana console. They are not intended for use by applications. For
* application consumption, use the nodes info API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture threadPool() {
return this.transport.performRequestAsync(new ThreadPoolRequest.Builder().build(), ThreadPoolRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.transforms
/**
* Get transforms. Returns configuration and usage information about transforms.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get transform statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture transforms(TransformsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) TransformsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get transforms. Returns configuration and usage information about transforms.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get transform statistics API.
*
* @param fn
* a function that initializes a builder to create the
* {@link TransformsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture transforms(
Function> fn) {
return transforms(fn.apply(new TransformsRequest.Builder()).build());
}
/**
* Get transforms. Returns configuration and usage information about transforms.
*
* CAT APIs are only intended for human consumption using the Kibana console or
* command line. They are not intended for use by applications. For application
* consumption, use the get transform statistics API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture transforms() {
return this.transport.performRequestAsync(new TransformsRequest.Builder().build(), TransformsRequest._ENDPOINT,
this.transportOptions);
}
}