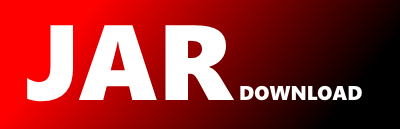
co.elastic.clients.elasticsearch.eql.HitsEvent Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.eql;
import co.elastic.clients.json.JsonData;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.JsonpSerializer;
import co.elastic.clients.json.JsonpUtils;
import co.elastic.clients.json.NamedDeserializer;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import co.elastic.clients.util.WithJsonObjectBuilderBase;
import jakarta.json.stream.JsonGenerator;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Supplier;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
// typedef: eql._types.HitsEvent
/**
*
* @see API
* specification
*/
@JsonpDeserializable
public class HitsEvent implements JsonpSerializable {
private final String index;
private final String id;
private final TEvent source;
@Nullable
private final Boolean missing;
private final Map> fields;
@Nullable
private final JsonpSerializer tEventSerializer;
// ---------------------------------------------------------------------------------------------
private HitsEvent(Builder builder) {
this.index = ApiTypeHelper.requireNonNull(builder.index, this, "index");
this.id = ApiTypeHelper.requireNonNull(builder.id, this, "id");
this.source = ApiTypeHelper.requireNonNull(builder.source, this, "source");
this.missing = builder.missing;
this.fields = ApiTypeHelper.unmodifiable(builder.fields);
this.tEventSerializer = builder.tEventSerializer;
}
public static HitsEvent of(Function, ObjectBuilder>> fn) {
return fn.apply(new Builder<>()).build();
}
/**
* Required - Name of the index containing the event.
*
* API name: {@code _index}
*/
public final String index() {
return this.index;
}
/**
* Required - Unique identifier for the event. This ID is only unique within the
* index.
*
* API name: {@code _id}
*/
public final String id() {
return this.id;
}
/**
* Required - Original JSON body passed for the event at index time.
*
* API name: {@code _source}
*/
public final TEvent source() {
return this.source;
}
/**
* Set to true
for events in a timespan-constrained sequence that
* do not meet a given condition.
*
* API name: {@code missing}
*/
@Nullable
public final Boolean missing() {
return this.missing;
}
/**
* API name: {@code fields}
*/
public final Map> fields() {
return this.fields;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
generator.writeKey("_index");
generator.write(this.index);
generator.writeKey("_id");
generator.write(this.id);
generator.writeKey("_source");
JsonpUtils.serialize(this.source, generator, tEventSerializer, mapper);
if (this.missing != null) {
generator.writeKey("missing");
generator.write(this.missing);
}
if (ApiTypeHelper.isDefined(this.fields)) {
generator.writeKey("fields");
generator.writeStartObject();
for (Map.Entry> item0 : this.fields.entrySet()) {
generator.writeKey(item0.getKey());
generator.writeStartArray();
if (item0.getValue() != null) {
for (JsonData item1 : item0.getValue()) {
item1.serialize(generator, mapper);
}
}
generator.writeEnd();
}
generator.writeEnd();
}
}
@Override
public String toString() {
return JsonpUtils.toString(this);
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link HitsEvent}.
*/
public static class Builder extends WithJsonObjectBuilderBase>
implements
ObjectBuilder> {
private String index;
private String id;
private TEvent source;
@Nullable
private Boolean missing;
@Nullable
private Map> fields;
@Nullable
private JsonpSerializer tEventSerializer;
/**
* Required - Name of the index containing the event.
*
* API name: {@code _index}
*/
public final Builder index(String value) {
this.index = value;
return this;
}
/**
* Required - Unique identifier for the event. This ID is only unique within the
* index.
*
* API name: {@code _id}
*/
public final Builder id(String value) {
this.id = value;
return this;
}
/**
* Required - Original JSON body passed for the event at index time.
*
* API name: {@code _source}
*/
public final Builder source(TEvent value) {
this.source = value;
return this;
}
/**
* Set to true
for events in a timespan-constrained sequence that
* do not meet a given condition.
*
* API name: {@code missing}
*/
public final Builder missing(@Nullable Boolean value) {
this.missing = value;
return this;
}
/**
* API name: {@code fields}
*
* Adds all entries of map
to fields
.
*/
public final Builder fields(Map> map) {
this.fields = _mapPutAll(this.fields, map);
return this;
}
/**
* API name: {@code fields}
*
* Adds an entry to fields
.
*/
public final Builder fields(String key, List value) {
this.fields = _mapPut(this.fields, key, value);
return this;
}
/**
* Serializer for TEvent. If not set, an attempt will be made to find a
* serializer from the JSON context.
*/
public final Builder tEventSerializer(@Nullable JsonpSerializer value) {
this.tEventSerializer = value;
return this;
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link HitsEvent}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public HitsEvent build() {
_checkSingleUse();
return new HitsEvent(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Create a JSON deserializer for HitsEvent
*/
public static JsonpDeserializer> createHitsEventDeserializer(
JsonpDeserializer tEventDeserializer) {
return ObjectBuilderDeserializer.createForObject((Supplier>) Builder::new,
op -> HitsEvent.setupHitsEventDeserializer(op, tEventDeserializer));
};
/**
* Json deserializer for {@link HitsEvent} based on named deserializers provided
* by the calling {@code JsonMapper}.
*/
public static final JsonpDeserializer> _DESERIALIZER = JsonpDeserializer
.lazy(() -> createHitsEventDeserializer(
new NamedDeserializer<>("co.elastic.clients:Deserializer:eql._types.HitsEvent.TEvent")));
protected static void setupHitsEventDeserializer(ObjectDeserializer> op,
JsonpDeserializer tEventDeserializer) {
op.add(Builder::index, JsonpDeserializer.stringDeserializer(), "_index");
op.add(Builder::id, JsonpDeserializer.stringDeserializer(), "_id");
op.add(Builder::source, tEventDeserializer, "_source");
op.add(Builder::missing, JsonpDeserializer.booleanDeserializer(), "missing");
op.add(Builder::fields,
JsonpDeserializer.stringMapDeserializer(JsonpDeserializer.arrayDeserializer(JsonData._DESERIALIZER)),
"fields");
}
}