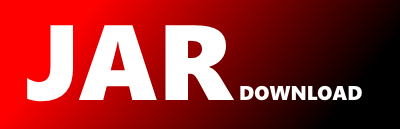
co.elastic.clients.elasticsearch.autoscaling.ElasticsearchAutoscalingAsyncClient Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.autoscaling;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the autoscaling namespace.
*/
public class ElasticsearchAutoscalingAsyncClient
extends
ApiClient {
public ElasticsearchAutoscalingAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchAutoscalingAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchAutoscalingAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchAutoscalingAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: autoscaling.delete_autoscaling_policy
/**
* Delete an autoscaling policy.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteAutoscalingPolicy(
DeleteAutoscalingPolicyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteAutoscalingPolicyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete an autoscaling policy.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteAutoscalingPolicyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteAutoscalingPolicy(
Function> fn) {
return deleteAutoscalingPolicy(fn.apply(new DeleteAutoscalingPolicyRequest.Builder()).build());
}
// ----- Endpoint: autoscaling.get_autoscaling_capacity
/**
* Get the autoscaling capacity.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* This API gets the current autoscaling capacity based on the configured
* autoscaling policy. It will return information to size the cluster
* appropriately to the current workload.
*
* The required_capacity
is calculated as the maximum of the
* required_capacity
result of all individual deciders that are
* enabled for the policy.
*
* The operator should verify that the current_nodes
match the
* operator’s knowledge of the cluster to avoid making autoscaling decisions
* based on stale or incomplete information.
*
* The response contains decider-specific information you can use to diagnose
* how and why autoscaling determined a certain capacity was required. This
* information is provided for diagnosis only. Do not use this information to
* make autoscaling decisions.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getAutoscalingCapacity(
GetAutoscalingCapacityRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetAutoscalingCapacityRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get the autoscaling capacity.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* This API gets the current autoscaling capacity based on the configured
* autoscaling policy. It will return information to size the cluster
* appropriately to the current workload.
*
* The required_capacity
is calculated as the maximum of the
* required_capacity
result of all individual deciders that are
* enabled for the policy.
*
* The operator should verify that the current_nodes
match the
* operator’s knowledge of the cluster to avoid making autoscaling decisions
* based on stale or incomplete information.
*
* The response contains decider-specific information you can use to diagnose
* how and why autoscaling determined a certain capacity was required. This
* information is provided for diagnosis only. Do not use this information to
* make autoscaling decisions.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetAutoscalingCapacityRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getAutoscalingCapacity(
Function> fn) {
return getAutoscalingCapacity(fn.apply(new GetAutoscalingCapacityRequest.Builder()).build());
}
/**
* Get the autoscaling capacity.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* This API gets the current autoscaling capacity based on the configured
* autoscaling policy. It will return information to size the cluster
* appropriately to the current workload.
*
* The required_capacity
is calculated as the maximum of the
* required_capacity
result of all individual deciders that are
* enabled for the policy.
*
* The operator should verify that the current_nodes
match the
* operator’s knowledge of the cluster to avoid making autoscaling decisions
* based on stale or incomplete information.
*
* The response contains decider-specific information you can use to diagnose
* how and why autoscaling determined a certain capacity was required. This
* information is provided for diagnosis only. Do not use this information to
* make autoscaling decisions.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getAutoscalingCapacity() {
return this.transport.performRequestAsync(new GetAutoscalingCapacityRequest.Builder().build(),
GetAutoscalingCapacityRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: autoscaling.get_autoscaling_policy
/**
* Get an autoscaling policy.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getAutoscalingPolicy(GetAutoscalingPolicyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetAutoscalingPolicyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get an autoscaling policy.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetAutoscalingPolicyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getAutoscalingPolicy(
Function> fn) {
return getAutoscalingPolicy(fn.apply(new GetAutoscalingPolicyRequest.Builder()).build());
}
// ----- Endpoint: autoscaling.put_autoscaling_policy
/**
* Create or update an autoscaling policy.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putAutoscalingPolicy(PutAutoscalingPolicyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutAutoscalingPolicyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create or update an autoscaling policy.
*
* NOTE: This feature is designed for indirect use by Elasticsearch Service,
* Elastic Cloud Enterprise, and Elastic Cloud on Kubernetes. Direct use is not
* supported.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutAutoscalingPolicyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putAutoscalingPolicy(
Function> fn) {
return putAutoscalingPolicy(fn.apply(new PutAutoscalingPolicyRequest.Builder()).build());
}
}