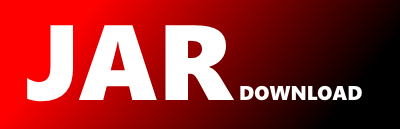
co.elastic.clients.elasticsearch.ccr.ElasticsearchCcrClient Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.ccr;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the ccr namespace.
*/
public class ElasticsearchCcrClient extends ApiClient {
public ElasticsearchCcrClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchCcrClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchCcrClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchCcrClient(this.transport, transportOptions);
}
// ----- Endpoint: ccr.delete_auto_follow_pattern
/**
* Deletes auto-follow patterns.
*
* @see Documentation
* on elastic.co
*/
public DeleteAutoFollowPatternResponse deleteAutoFollowPattern(DeleteAutoFollowPatternRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteAutoFollowPatternRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes auto-follow patterns.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteAutoFollowPatternRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteAutoFollowPatternResponse deleteAutoFollowPattern(
Function> fn)
throws IOException, ElasticsearchException {
return deleteAutoFollowPattern(fn.apply(new DeleteAutoFollowPatternRequest.Builder()).build());
}
// ----- Endpoint: ccr.follow
/**
* Creates a new follower index configured to follow the referenced leader
* index.
*
* @see Documentation
* on elastic.co
*/
public FollowResponse follow(FollowRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FollowRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a new follower index configured to follow the referenced leader
* index.
*
* @param fn
* a function that initializes a builder to create the
* {@link FollowRequest}
* @see Documentation
* on elastic.co
*/
public final FollowResponse follow(Function> fn)
throws IOException, ElasticsearchException {
return follow(fn.apply(new FollowRequest.Builder()).build());
}
// ----- Endpoint: ccr.follow_info
/**
* Retrieves information about all follower indices, including parameters and
* status for each follower index
*
* @see Documentation
* on elastic.co
*/
public FollowInfoResponse followInfo(FollowInfoRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FollowInfoRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves information about all follower indices, including parameters and
* status for each follower index
*
* @param fn
* a function that initializes a builder to create the
* {@link FollowInfoRequest}
* @see Documentation
* on elastic.co
*/
public final FollowInfoResponse followInfo(Function> fn)
throws IOException, ElasticsearchException {
return followInfo(fn.apply(new FollowInfoRequest.Builder()).build());
}
// ----- Endpoint: ccr.follow_stats
/**
* Retrieves follower stats. return shard-level stats about the following tasks
* associated with each shard for the specified indices.
*
* @see Documentation
* on elastic.co
*/
public FollowStatsResponse followStats(FollowStatsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FollowStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves follower stats. return shard-level stats about the following tasks
* associated with each shard for the specified indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link FollowStatsRequest}
* @see Documentation
* on elastic.co
*/
public final FollowStatsResponse followStats(
Function> fn)
throws IOException, ElasticsearchException {
return followStats(fn.apply(new FollowStatsRequest.Builder()).build());
}
// ----- Endpoint: ccr.forget_follower
/**
* Removes the follower retention leases from the leader.
*
* @see Documentation
* on elastic.co
*/
public ForgetFollowerResponse forgetFollower(ForgetFollowerRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ForgetFollowerRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Removes the follower retention leases from the leader.
*
* @param fn
* a function that initializes a builder to create the
* {@link ForgetFollowerRequest}
* @see Documentation
* on elastic.co
*/
public final ForgetFollowerResponse forgetFollower(
Function> fn)
throws IOException, ElasticsearchException {
return forgetFollower(fn.apply(new ForgetFollowerRequest.Builder()).build());
}
// ----- Endpoint: ccr.get_auto_follow_pattern
/**
* Gets configured auto-follow patterns. Returns the specified auto-follow
* pattern collection.
*
* @see Documentation
* on elastic.co
*/
public GetAutoFollowPatternResponse getAutoFollowPattern(GetAutoFollowPatternRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetAutoFollowPatternRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Gets configured auto-follow patterns. Returns the specified auto-follow
* pattern collection.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetAutoFollowPatternRequest}
* @see Documentation
* on elastic.co
*/
public final GetAutoFollowPatternResponse getAutoFollowPattern(
Function> fn)
throws IOException, ElasticsearchException {
return getAutoFollowPattern(fn.apply(new GetAutoFollowPatternRequest.Builder()).build());
}
/**
* Gets configured auto-follow patterns. Returns the specified auto-follow
* pattern collection.
*
* @see Documentation
* on elastic.co
*/
public GetAutoFollowPatternResponse getAutoFollowPattern() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetAutoFollowPatternRequest.Builder().build(),
GetAutoFollowPatternRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ccr.pause_auto_follow_pattern
/**
* Pauses an auto-follow pattern
*
* @see Documentation
* on elastic.co
*/
public PauseAutoFollowPatternResponse pauseAutoFollowPattern(PauseAutoFollowPatternRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PauseAutoFollowPatternRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Pauses an auto-follow pattern
*
* @param fn
* a function that initializes a builder to create the
* {@link PauseAutoFollowPatternRequest}
* @see Documentation
* on elastic.co
*/
public final PauseAutoFollowPatternResponse pauseAutoFollowPattern(
Function> fn)
throws IOException, ElasticsearchException {
return pauseAutoFollowPattern(fn.apply(new PauseAutoFollowPatternRequest.Builder()).build());
}
// ----- Endpoint: ccr.pause_follow
/**
* Pauses a follower index. The follower index will not fetch any additional
* operations from the leader index.
*
* @see Documentation
* on elastic.co
*/
public PauseFollowResponse pauseFollow(PauseFollowRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PauseFollowRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Pauses a follower index. The follower index will not fetch any additional
* operations from the leader index.
*
* @param fn
* a function that initializes a builder to create the
* {@link PauseFollowRequest}
* @see Documentation
* on elastic.co
*/
public final PauseFollowResponse pauseFollow(
Function> fn)
throws IOException, ElasticsearchException {
return pauseFollow(fn.apply(new PauseFollowRequest.Builder()).build());
}
// ----- Endpoint: ccr.put_auto_follow_pattern
/**
* Creates a new named collection of auto-follow patterns against a specified
* remote cluster. Newly created indices on the remote cluster matching any of
* the specified patterns will be automatically configured as follower indices.
*
* @see Documentation
* on elastic.co
*/
public PutAutoFollowPatternResponse putAutoFollowPattern(PutAutoFollowPatternRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutAutoFollowPatternRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a new named collection of auto-follow patterns against a specified
* remote cluster. Newly created indices on the remote cluster matching any of
* the specified patterns will be automatically configured as follower indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutAutoFollowPatternRequest}
* @see Documentation
* on elastic.co
*/
public final PutAutoFollowPatternResponse putAutoFollowPattern(
Function> fn)
throws IOException, ElasticsearchException {
return putAutoFollowPattern(fn.apply(new PutAutoFollowPatternRequest.Builder()).build());
}
// ----- Endpoint: ccr.resume_auto_follow_pattern
/**
* Resumes an auto-follow pattern that has been paused
*
* @see Documentation
* on elastic.co
*/
public ResumeAutoFollowPatternResponse resumeAutoFollowPattern(ResumeAutoFollowPatternRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ResumeAutoFollowPatternRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Resumes an auto-follow pattern that has been paused
*
* @param fn
* a function that initializes a builder to create the
* {@link ResumeAutoFollowPatternRequest}
* @see Documentation
* on elastic.co
*/
public final ResumeAutoFollowPatternResponse resumeAutoFollowPattern(
Function> fn)
throws IOException, ElasticsearchException {
return resumeAutoFollowPattern(fn.apply(new ResumeAutoFollowPatternRequest.Builder()).build());
}
// ----- Endpoint: ccr.resume_follow
/**
* Resumes a follower index that has been paused
*
* @see Documentation
* on elastic.co
*/
public ResumeFollowResponse resumeFollow(ResumeFollowRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ResumeFollowRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Resumes a follower index that has been paused
*
* @param fn
* a function that initializes a builder to create the
* {@link ResumeFollowRequest}
* @see Documentation
* on elastic.co
*/
public final ResumeFollowResponse resumeFollow(
Function> fn)
throws IOException, ElasticsearchException {
return resumeFollow(fn.apply(new ResumeFollowRequest.Builder()).build());
}
// ----- Endpoint: ccr.stats
/**
* Gets all stats related to cross-cluster replication.
*
* @see Documentation
* on elastic.co
*/
public CcrStatsResponse stats() throws IOException, ElasticsearchException {
return this.transport.performRequest(CcrStatsRequest._INSTANCE, CcrStatsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ccr.unfollow
/**
* Stops the following task associated with a follower index and removes index
* metadata and settings associated with cross-cluster replication.
*
* @see Documentation
* on elastic.co
*/
public UnfollowResponse unfollow(UnfollowRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UnfollowRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stops the following task associated with a follower index and removes index
* metadata and settings associated with cross-cluster replication.
*
* @param fn
* a function that initializes a builder to create the
* {@link UnfollowRequest}
* @see Documentation
* on elastic.co
*/
public final UnfollowResponse unfollow(Function> fn)
throws IOException, ElasticsearchException {
return unfollow(fn.apply(new UnfollowRequest.Builder()).build());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy