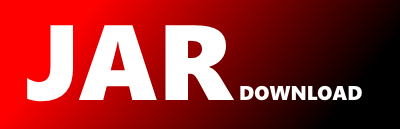
co.elastic.clients.elasticsearch.cluster.ElasticsearchClusterAsyncClient Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.cluster;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.BooleanResponse;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the cluster namespace.
*/
public class ElasticsearchClusterAsyncClient
extends
ApiClient {
public ElasticsearchClusterAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchClusterAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchClusterAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchClusterAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: cluster.allocation_explain
/**
* Provides explanations for shard allocations in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture allocationExplain(AllocationExplainRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AllocationExplainRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Provides explanations for shard allocations in the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link AllocationExplainRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture allocationExplain(
Function> fn) {
return allocationExplain(fn.apply(new AllocationExplainRequest.Builder()).build());
}
/**
* Provides explanations for shard allocations in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture allocationExplain() {
return this.transport.performRequestAsync(new AllocationExplainRequest.Builder().build(),
AllocationExplainRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.delete_component_template
/**
* Delete component templates. Deletes component templates. Component templates
* are building blocks for constructing index templates that specify index
* mappings, settings, and aliases.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteComponentTemplate(
DeleteComponentTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteComponentTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete component templates. Deletes component templates. Component templates
* are building blocks for constructing index templates that specify index
* mappings, settings, and aliases.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteComponentTemplate(
Function> fn) {
return deleteComponentTemplate(fn.apply(new DeleteComponentTemplateRequest.Builder()).build());
}
// ----- Endpoint: cluster.delete_voting_config_exclusions
/**
* Clears cluster voting config exclusions.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteVotingConfigExclusions(
DeleteVotingConfigExclusionsRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) DeleteVotingConfigExclusionsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clears cluster voting config exclusions.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteVotingConfigExclusionsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteVotingConfigExclusions(
Function> fn) {
return deleteVotingConfigExclusions(fn.apply(new DeleteVotingConfigExclusionsRequest.Builder()).build());
}
/**
* Clears cluster voting config exclusions.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteVotingConfigExclusions() {
return this.transport.performRequestAsync(new DeleteVotingConfigExclusionsRequest.Builder().build(),
DeleteVotingConfigExclusionsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.exists_component_template
/**
* Check component templates. Returns information about whether a particular
* component template exists.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture existsComponentTemplate(ExistsComponentTemplateRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) ExistsComponentTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Check component templates. Returns information about whether a particular
* component template exists.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExistsComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture existsComponentTemplate(
Function> fn) {
return existsComponentTemplate(fn.apply(new ExistsComponentTemplateRequest.Builder()).build());
}
// ----- Endpoint: cluster.get_component_template
/**
* Get component templates. Retrieves information about component templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getComponentTemplate(GetComponentTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetComponentTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get component templates. Retrieves information about component templates.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getComponentTemplate(
Function> fn) {
return getComponentTemplate(fn.apply(new GetComponentTemplateRequest.Builder()).build());
}
/**
* Get component templates. Retrieves information about component templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getComponentTemplate() {
return this.transport.performRequestAsync(new GetComponentTemplateRequest.Builder().build(),
GetComponentTemplateRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.get_settings
/**
* Returns cluster-wide settings. By default, it returns only settings that have
* been explicitly defined.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getSettings(GetClusterSettingsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetClusterSettingsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns cluster-wide settings. By default, it returns only settings that have
* been explicitly defined.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetClusterSettingsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getSettings(
Function> fn) {
return getSettings(fn.apply(new GetClusterSettingsRequest.Builder()).build());
}
/**
* Returns cluster-wide settings. By default, it returns only settings that have
* been explicitly defined.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getSettings() {
return this.transport.performRequestAsync(new GetClusterSettingsRequest.Builder().build(),
GetClusterSettingsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.health
/**
* The cluster health API returns a simple status on the health of the cluster.
* You can also use the API to get the health status of only specified data
* streams and indices. For data streams, the API retrieves the health status of
* the stream’s backing indices. The cluster health status is: green, yellow or
* red. On the shard level, a red status indicates that the specific shard is
* not allocated in the cluster, yellow means that the primary shard is
* allocated but replicas are not, and green means that all shards are
* allocated. The index level status is controlled by the worst shard status.
* The cluster status is controlled by the worst index status.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture health(HealthRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HealthRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* The cluster health API returns a simple status on the health of the cluster.
* You can also use the API to get the health status of only specified data
* streams and indices. For data streams, the API retrieves the health status of
* the stream’s backing indices. The cluster health status is: green, yellow or
* red. On the shard level, a red status indicates that the specific shard is
* not allocated in the cluster, yellow means that the primary shard is
* allocated but replicas are not, and green means that all shards are
* allocated. The index level status is controlled by the worst shard status.
* The cluster status is controlled by the worst index status.
*
* @param fn
* a function that initializes a builder to create the
* {@link HealthRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture health(
Function> fn) {
return health(fn.apply(new HealthRequest.Builder()).build());
}
/**
* The cluster health API returns a simple status on the health of the cluster.
* You can also use the API to get the health status of only specified data
* streams and indices. For data streams, the API retrieves the health status of
* the stream’s backing indices. The cluster health status is: green, yellow or
* red. On the shard level, a red status indicates that the specific shard is
* not allocated in the cluster, yellow means that the primary shard is
* allocated but replicas are not, and green means that all shards are
* allocated. The index level status is controlled by the worst shard status.
* The cluster status is controlled by the worst index status.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture health() {
return this.transport.performRequestAsync(new HealthRequest.Builder().build(), HealthRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.info
/**
* Get cluster info. Returns basic information about the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture info(ClusterInfoRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClusterInfoRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get cluster info. Returns basic information about the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClusterInfoRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture info(
Function> fn) {
return info(fn.apply(new ClusterInfoRequest.Builder()).build());
}
// ----- Endpoint: cluster.pending_tasks
/**
* Returns cluster-level changes (such as create index, update mapping, allocate
* or fail shard) that have not yet been executed. NOTE: This API returns a list
* of any pending updates to the cluster state. These are distinct from the
* tasks reported by the Task Management API which include periodic tasks and
* tasks initiated by the user, such as node stats, search queries, or create
* index requests. However, if a user-initiated task such as a create index
* command causes a cluster state update, the activity of this task might be
* reported by both task api and pending cluster tasks API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture pendingTasks(PendingTasksRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PendingTasksRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns cluster-level changes (such as create index, update mapping, allocate
* or fail shard) that have not yet been executed. NOTE: This API returns a list
* of any pending updates to the cluster state. These are distinct from the
* tasks reported by the Task Management API which include periodic tasks and
* tasks initiated by the user, such as node stats, search queries, or create
* index requests. However, if a user-initiated task such as a create index
* command causes a cluster state update, the activity of this task might be
* reported by both task api and pending cluster tasks API.
*
* @param fn
* a function that initializes a builder to create the
* {@link PendingTasksRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture pendingTasks(
Function> fn) {
return pendingTasks(fn.apply(new PendingTasksRequest.Builder()).build());
}
/**
* Returns cluster-level changes (such as create index, update mapping, allocate
* or fail shard) that have not yet been executed. NOTE: This API returns a list
* of any pending updates to the cluster state. These are distinct from the
* tasks reported by the Task Management API which include periodic tasks and
* tasks initiated by the user, such as node stats, search queries, or create
* index requests. However, if a user-initiated task such as a create index
* command causes a cluster state update, the activity of this task might be
* reported by both task api and pending cluster tasks API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture pendingTasks() {
return this.transport.performRequestAsync(new PendingTasksRequest.Builder().build(),
PendingTasksRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.post_voting_config_exclusions
/**
* Updates the cluster voting config exclusions by node ids or node names.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture postVotingConfigExclusions(PostVotingConfigExclusionsRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) PostVotingConfigExclusionsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Updates the cluster voting config exclusions by node ids or node names.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostVotingConfigExclusionsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture postVotingConfigExclusions(
Function> fn) {
return postVotingConfigExclusions(fn.apply(new PostVotingConfigExclusionsRequest.Builder()).build());
}
/**
* Updates the cluster voting config exclusions by node ids or node names.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture postVotingConfigExclusions() {
return this.transport.performRequestAsync(new PostVotingConfigExclusionsRequest.Builder().build(),
PostVotingConfigExclusionsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.put_component_template
/**
* Create or update a component template. Creates or updates a component
* template. Component templates are building blocks for constructing index
* templates that specify index mappings, settings, and aliases.
*
* An index template can be composed of multiple component templates. To use a
* component template, specify it in an index template’s
* composed_of
list. Component templates are only applied to new
* data streams and indices as part of a matching index template.
*
* Settings and mappings specified directly in the index template or the create
* index request override any settings or mappings specified in a component
* template.
*
* Component templates are only used during index creation. For data streams,
* this includes data stream creation and the creation of a stream’s backing
* indices. Changes to component templates do not affect existing indices,
* including a stream’s backing indices.
*
* You can use C-style /* *\/
block comments in component
* templates. You can include comments anywhere in the request body except
* before the opening curly bracket.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putComponentTemplate(PutComponentTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutComponentTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create or update a component template. Creates or updates a component
* template. Component templates are building blocks for constructing index
* templates that specify index mappings, settings, and aliases.
*
* An index template can be composed of multiple component templates. To use a
* component template, specify it in an index template’s
* composed_of
list. Component templates are only applied to new
* data streams and indices as part of a matching index template.
*
* Settings and mappings specified directly in the index template or the create
* index request override any settings or mappings specified in a component
* template.
*
* Component templates are only used during index creation. For data streams,
* this includes data stream creation and the creation of a stream’s backing
* indices. Changes to component templates do not affect existing indices,
* including a stream’s backing indices.
*
* You can use C-style /* *\/
block comments in component
* templates. You can include comments anywhere in the request body except
* before the opening curly bracket.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putComponentTemplate(
Function> fn) {
return putComponentTemplate(fn.apply(new PutComponentTemplateRequest.Builder()).build());
}
// ----- Endpoint: cluster.put_settings
/**
* Updates the cluster settings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putSettings(PutClusterSettingsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutClusterSettingsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Updates the cluster settings.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutClusterSettingsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putSettings(
Function> fn) {
return putSettings(fn.apply(new PutClusterSettingsRequest.Builder()).build());
}
/**
* Updates the cluster settings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putSettings() {
return this.transport.performRequestAsync(new PutClusterSettingsRequest.Builder().build(),
PutClusterSettingsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.remote_info
/**
* The cluster remote info API allows you to retrieve all of the configured
* remote cluster information. It returns connection and endpoint information
* keyed by the configured remote cluster alias.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture remoteInfo() {
return this.transport.performRequestAsync(RemoteInfoRequest._INSTANCE, RemoteInfoRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.reroute
/**
* Allows to manually change the allocation of individual shards in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture reroute(RerouteRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RerouteRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Allows to manually change the allocation of individual shards in the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link RerouteRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture reroute(
Function> fn) {
return reroute(fn.apply(new RerouteRequest.Builder()).build());
}
/**
* Allows to manually change the allocation of individual shards in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture reroute() {
return this.transport.performRequestAsync(new RerouteRequest.Builder().build(), RerouteRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.state
/**
* Returns a comprehensive information about the state of the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture state(StateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns a comprehensive information about the state of the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link StateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture state(
Function> fn) {
return state(fn.apply(new StateRequest.Builder()).build());
}
/**
* Returns a comprehensive information about the state of the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture state() {
return this.transport.performRequestAsync(new StateRequest.Builder().build(), StateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.stats
/**
* Returns cluster statistics. It returns basic index metrics (shard numbers,
* store size, memory usage) and information about the current nodes that form
* the cluster (number, roles, os, jvm versions, memory usage, cpu and installed
* plugins).
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture stats(ClusterStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClusterStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns cluster statistics. It returns basic index metrics (shard numbers,
* store size, memory usage) and information about the current nodes that form
* the cluster (number, roles, os, jvm versions, memory usage, cpu and installed
* plugins).
*
* @param fn
* a function that initializes a builder to create the
* {@link ClusterStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture stats(
Function> fn) {
return stats(fn.apply(new ClusterStatsRequest.Builder()).build());
}
/**
* Returns cluster statistics. It returns basic index metrics (shard numbers,
* store size, memory usage) and information about the current nodes that form
* the cluster (number, roles, os, jvm versions, memory usage, cpu and installed
* plugins).
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture stats() {
return this.transport.performRequestAsync(new ClusterStatsRequest.Builder().build(),
ClusterStatsRequest._ENDPOINT, this.transportOptions);
}
}