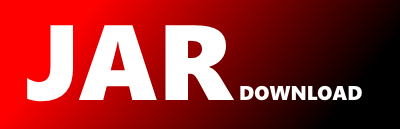
co.elastic.clients.elasticsearch.connector.ElasticsearchConnectorAsyncClient Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.connector;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the connector namespace.
*/
public class ElasticsearchConnectorAsyncClient
extends
ApiClient {
public ElasticsearchConnectorAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchConnectorAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchConnectorAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchConnectorAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: connector.check_in
/**
* Check in a connector.
*
* Update the last_seen
field in the connector and set it to the
* current timestamp.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture checkIn(CheckInRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CheckInRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Check in a connector.
*
* Update the last_seen
field in the connector and set it to the
* current timestamp.
*
* @param fn
* a function that initializes a builder to create the
* {@link CheckInRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture checkIn(
Function> fn) {
return checkIn(fn.apply(new CheckInRequest.Builder()).build());
}
// ----- Endpoint: connector.delete
/**
* Delete a connector.
*
* Removes a connector and associated sync jobs. This is a destructive action
* that is not recoverable. NOTE: This action doesn’t delete any API keys,
* ingest pipelines, or data indices associated with the connector. These need
* to be removed manually.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture delete(DeleteConnectorRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteConnectorRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a connector.
*
* Removes a connector and associated sync jobs. This is a destructive action
* that is not recoverable. NOTE: This action doesn’t delete any API keys,
* ingest pipelines, or data indices associated with the connector. These need
* to be removed manually.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteConnectorRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture delete(
Function> fn) {
return delete(fn.apply(new DeleteConnectorRequest.Builder()).build());
}
// ----- Endpoint: connector.get
/**
* Get a connector.
*
* Get the details about a connector.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture get(GetConnectorRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetConnectorRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get a connector.
*
* Get the details about a connector.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetConnectorRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture get(
Function> fn) {
return get(fn.apply(new GetConnectorRequest.Builder()).build());
}
// ----- Endpoint: connector.list
/**
* Get all connectors.
*
* Get information about all connectors.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture list(ListRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ListRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get all connectors.
*
* Get information about all connectors.
*
* @param fn
* a function that initializes a builder to create the
* {@link ListRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture list(Function> fn) {
return list(fn.apply(new ListRequest.Builder()).build());
}
/**
* Get all connectors.
*
* Get information about all connectors.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture list() {
return this.transport.performRequestAsync(new ListRequest.Builder().build(), ListRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: connector.post
/**
* Create a connector.
*
* Connectors are Elasticsearch integrations that bring content from third-party
* data sources, which can be deployed on Elastic Cloud or hosted on your own
* infrastructure. Elastic managed connectors (Native connectors) are a managed
* service on Elastic Cloud. Self-managed connectors (Connector clients) are
* self-managed on your infrastructure.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture post(PostRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PostRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create a connector.
*
* Connectors are Elasticsearch integrations that bring content from third-party
* data sources, which can be deployed on Elastic Cloud or hosted on your own
* infrastructure. Elastic managed connectors (Native connectors) are a managed
* service on Elastic Cloud. Self-managed connectors (Connector clients) are
* self-managed on your infrastructure.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture post(Function> fn) {
return post(fn.apply(new PostRequest.Builder()).build());
}
/**
* Create a connector.
*
* Connectors are Elasticsearch integrations that bring content from third-party
* data sources, which can be deployed on Elastic Cloud or hosted on your own
* infrastructure. Elastic managed connectors (Native connectors) are a managed
* service on Elastic Cloud. Self-managed connectors (Connector clients) are
* self-managed on your infrastructure.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture post() {
return this.transport.performRequestAsync(new PostRequest.Builder().build(), PostRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: connector.put
/**
* Create or update a connector.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture put(PutRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create or update a connector.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture put(Function> fn) {
return put(fn.apply(new PutRequest.Builder()).build());
}
/**
* Create or update a connector.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture put() {
return this.transport.performRequestAsync(new PutRequest.Builder().build(), PutRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: connector.sync_job_cancel
/**
* Cancel a connector sync job.
*
* Cancel a connector sync job, which sets the status to cancelling and updates
* cancellation_requested_at
to the current time. The connector
* service is then responsible for setting the status of connector sync jobs to
* cancelled.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture syncJobCancel(SyncJobCancelRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SyncJobCancelRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Cancel a connector sync job.
*
* Cancel a connector sync job, which sets the status to cancelling and updates
* cancellation_requested_at
to the current time. The connector
* service is then responsible for setting the status of connector sync jobs to
* cancelled.
*
* @param fn
* a function that initializes a builder to create the
* {@link SyncJobCancelRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture syncJobCancel(
Function> fn) {
return syncJobCancel(fn.apply(new SyncJobCancelRequest.Builder()).build());
}
// ----- Endpoint: connector.sync_job_delete
/**
* Delete a connector sync job.
*
* Remove a connector sync job and its associated data. This is a destructive
* action that is not recoverable.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture syncJobDelete(SyncJobDeleteRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SyncJobDeleteRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a connector sync job.
*
* Remove a connector sync job and its associated data. This is a destructive
* action that is not recoverable.
*
* @param fn
* a function that initializes a builder to create the
* {@link SyncJobDeleteRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture syncJobDelete(
Function> fn) {
return syncJobDelete(fn.apply(new SyncJobDeleteRequest.Builder()).build());
}
// ----- Endpoint: connector.sync_job_get
/**
* Get a connector sync job.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture syncJobGet(SyncJobGetRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SyncJobGetRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get a connector sync job.
*
* @param fn
* a function that initializes a builder to create the
* {@link SyncJobGetRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture syncJobGet(
Function> fn) {
return syncJobGet(fn.apply(new SyncJobGetRequest.Builder()).build());
}
// ----- Endpoint: connector.sync_job_list
/**
* Get all connector sync jobs.
*
* Get information about all stored connector sync jobs listed by their creation
* date in ascending order.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture syncJobList(SyncJobListRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SyncJobListRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get all connector sync jobs.
*
* Get information about all stored connector sync jobs listed by their creation
* date in ascending order.
*
* @param fn
* a function that initializes a builder to create the
* {@link SyncJobListRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture syncJobList(
Function> fn) {
return syncJobList(fn.apply(new SyncJobListRequest.Builder()).build());
}
/**
* Get all connector sync jobs.
*
* Get information about all stored connector sync jobs listed by their creation
* date in ascending order.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture syncJobList() {
return this.transport.performRequestAsync(new SyncJobListRequest.Builder().build(),
SyncJobListRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: connector.sync_job_post
/**
* Create a connector sync job.
*
* Create a connector sync job document in the internal index and initialize its
* counters and timestamps with default values.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture syncJobPost(SyncJobPostRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SyncJobPostRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create a connector sync job.
*
* Create a connector sync job document in the internal index and initialize its
* counters and timestamps with default values.
*
* @param fn
* a function that initializes a builder to create the
* {@link SyncJobPostRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture syncJobPost(
Function> fn) {
return syncJobPost(fn.apply(new SyncJobPostRequest.Builder()).build());
}
// ----- Endpoint: connector.update_active_filtering
/**
* Activate the connector draft filter.
*
* Activates the valid draft filtering for a connector.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateActiveFiltering(
UpdateActiveFilteringRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateActiveFilteringRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Activate the connector draft filter.
*
* Activates the valid draft filtering for a connector.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateActiveFilteringRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateActiveFiltering(
Function> fn) {
return updateActiveFiltering(fn.apply(new UpdateActiveFilteringRequest.Builder()).build());
}
// ----- Endpoint: connector.update_api_key_id
/**
* Update the connector API key ID.
*
* Update the api_key_id
and api_key_secret_id
fields
* of a connector. You can specify the ID of the API key used for authorization
* and the ID of the connector secret where the API key is stored. The connector
* secret ID is required only for Elastic managed (native) connectors.
* Self-managed connectors (connector clients) do not use this field.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateApiKeyId(UpdateApiKeyIdRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateApiKeyIdRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector API key ID.
*
* Update the api_key_id
and api_key_secret_id
fields
* of a connector. You can specify the ID of the API key used for authorization
* and the ID of the connector secret where the API key is stored. The connector
* secret ID is required only for Elastic managed (native) connectors.
* Self-managed connectors (connector clients) do not use this field.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateApiKeyIdRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateApiKeyId(
Function> fn) {
return updateApiKeyId(fn.apply(new UpdateApiKeyIdRequest.Builder()).build());
}
// ----- Endpoint: connector.update_configuration
/**
* Update the connector configuration.
*
* Update the configuration field in the connector document.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateConfiguration(UpdateConfigurationRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateConfigurationRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector configuration.
*
* Update the configuration field in the connector document.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateConfigurationRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateConfiguration(
Function> fn) {
return updateConfiguration(fn.apply(new UpdateConfigurationRequest.Builder()).build());
}
// ----- Endpoint: connector.update_error
/**
* Update the connector error field.
*
* Set the error field for the connector. If the error provided in the request
* body is non-null, the connector’s status is updated to error. Otherwise, if
* the error is reset to null, the connector status is updated to connected.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateError(UpdateErrorRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateErrorRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector error field.
*
* Set the error field for the connector. If the error provided in the request
* body is non-null, the connector’s status is updated to error. Otherwise, if
* the error is reset to null, the connector status is updated to connected.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateErrorRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateError(
Function> fn) {
return updateError(fn.apply(new UpdateErrorRequest.Builder()).build());
}
// ----- Endpoint: connector.update_filtering
/**
* Update the connector filtering.
*
* Update the draft filtering configuration of a connector and marks the draft
* validation state as edited. The filtering draft is activated once validated
* by the running Elastic connector service. The filtering property is used to
* configure sync rules (both basic and advanced) for a connector.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateFiltering(UpdateFilteringRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateFilteringRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector filtering.
*
* Update the draft filtering configuration of a connector and marks the draft
* validation state as edited. The filtering draft is activated once validated
* by the running Elastic connector service. The filtering property is used to
* configure sync rules (both basic and advanced) for a connector.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateFilteringRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateFiltering(
Function> fn) {
return updateFiltering(fn.apply(new UpdateFilteringRequest.Builder()).build());
}
// ----- Endpoint: connector.update_filtering_validation
/**
* Update the connector draft filtering validation.
*
* Update the draft filtering validation info for a connector.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateFilteringValidation(
UpdateFilteringValidationRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateFilteringValidationRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector draft filtering validation.
*
* Update the draft filtering validation info for a connector.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateFilteringValidationRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateFilteringValidation(
Function> fn) {
return updateFilteringValidation(fn.apply(new UpdateFilteringValidationRequest.Builder()).build());
}
// ----- Endpoint: connector.update_index_name
/**
* Update the connector index name.
*
* Update the index_name
field of a connector, specifying the index
* where the data ingested by the connector is stored.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateIndexName(UpdateIndexNameRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateIndexNameRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector index name.
*
* Update the index_name
field of a connector, specifying the index
* where the data ingested by the connector is stored.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateIndexNameRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateIndexName(
Function> fn) {
return updateIndexName(fn.apply(new UpdateIndexNameRequest.Builder()).build());
}
// ----- Endpoint: connector.update_name
/**
* Update the connector name and description.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateName(UpdateNameRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateNameRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector name and description.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateNameRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateName(
Function> fn) {
return updateName(fn.apply(new UpdateNameRequest.Builder()).build());
}
// ----- Endpoint: connector.update_native
/**
* Update the connector is_native flag.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateNative(UpdateNativeRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateNativeRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector is_native flag.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateNativeRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateNative(
Function> fn) {
return updateNative(fn.apply(new UpdateNativeRequest.Builder()).build());
}
// ----- Endpoint: connector.update_pipeline
/**
* Update the connector pipeline.
*
* When you create a new connector, the configuration of an ingest pipeline is
* populated with default settings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updatePipeline(UpdatePipelineRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdatePipelineRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector pipeline.
*
* When you create a new connector, the configuration of an ingest pipeline is
* populated with default settings.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdatePipelineRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updatePipeline(
Function> fn) {
return updatePipeline(fn.apply(new UpdatePipelineRequest.Builder()).build());
}
// ----- Endpoint: connector.update_scheduling
/**
* Update the connector scheduling.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateScheduling(UpdateSchedulingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateSchedulingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector scheduling.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateSchedulingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateScheduling(
Function> fn) {
return updateScheduling(fn.apply(new UpdateSchedulingRequest.Builder()).build());
}
// ----- Endpoint: connector.update_service_type
/**
* Update the connector service type.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateServiceType(UpdateServiceTypeRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateServiceTypeRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector service type.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateServiceTypeRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateServiceType(
Function> fn) {
return updateServiceType(fn.apply(new UpdateServiceTypeRequest.Builder()).build());
}
// ----- Endpoint: connector.update_status
/**
* Update the connector status.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture updateStatus(UpdateStatusRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateStatusRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update the connector status.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateStatusRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture updateStatus(
Function> fn) {
return updateStatus(fn.apply(new UpdateStatusRequest.Builder()).build());
}
}