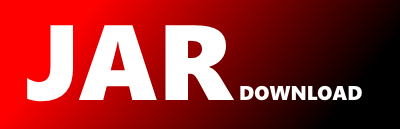
co.elastic.clients.elasticsearch.core.OpenPointInTimeRequest Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.core;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.elasticsearch._types.ExpandWildcard;
import co.elastic.clients.elasticsearch._types.RequestBase;
import co.elastic.clients.elasticsearch._types.Time;
import co.elastic.clients.elasticsearch._types.query_dsl.Query;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.endpoints.SimpleEndpoint;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import jakarta.json.stream.JsonGenerator;
import java.lang.Boolean;
import java.lang.String;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Collectors;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
// typedef: _global.open_point_in_time.Request
/**
* Open a point in time.
*
* A search request by default runs against the most recent visible data of the
* target indices, which is called point in time. Elasticsearch pit (point in
* time) is a lightweight view into the state of the data as it existed when
* initiated. In some cases, it’s preferred to perform multiple search requests
* using the same point in time. For example, if refreshes happen between
* search_after
requests, then the results of those requests might
* not be consistent as changes happening between searches are only visible to
* the more recent point in time.
*
* A point in time must be opened explicitly before being used in search
* requests. The keep_alive
parameter tells Elasticsearch how long
* it should persist.
*
* @see API
* specification
*/
@JsonpDeserializable
public class OpenPointInTimeRequest extends RequestBase implements JsonpSerializable {
@Nullable
private final Boolean allowPartialSearchResults;
private final List expandWildcards;
@Nullable
private final Boolean ignoreUnavailable;
private final List index;
@Nullable
private final Query indexFilter;
private final Time keepAlive;
@Nullable
private final String preference;
@Nullable
private final String routing;
// ---------------------------------------------------------------------------------------------
private OpenPointInTimeRequest(Builder builder) {
this.allowPartialSearchResults = builder.allowPartialSearchResults;
this.expandWildcards = ApiTypeHelper.unmodifiable(builder.expandWildcards);
this.ignoreUnavailable = builder.ignoreUnavailable;
this.index = ApiTypeHelper.unmodifiableRequired(builder.index, this, "index");
this.indexFilter = builder.indexFilter;
this.keepAlive = ApiTypeHelper.requireNonNull(builder.keepAlive, this, "keepAlive");
this.preference = builder.preference;
this.routing = builder.routing;
}
public static OpenPointInTimeRequest of(Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* If false
, creating a point in time request when a shard is
* missing or unavailable will throw an exception. If true
, the
* point in time will contain all the shards that are available at the time of
* the request.
*
* API name: {@code allow_partial_search_results}
*/
@Nullable
public final Boolean allowPartialSearchResults() {
return this.allowPartialSearchResults;
}
/**
* Type of index that wildcard patterns can match. If the request can target
* data streams, this argument determines whether wildcard expressions match
* hidden data streams. Supports comma-separated values, such as
* open,hidden
. Valid values are: all
,
* open
, closed
, hidden
,
* none
.
*
* API name: {@code expand_wildcards}
*/
public final List expandWildcards() {
return this.expandWildcards;
}
/**
* If false
, the request returns an error if it targets a missing
* or closed index.
*
* API name: {@code ignore_unavailable}
*/
@Nullable
public final Boolean ignoreUnavailable() {
return this.ignoreUnavailable;
}
/**
* Required - A comma-separated list of index names to open point in time; use
* _all
or empty string to perform the operation on all indices
*
* API name: {@code index}
*/
public final List index() {
return this.index;
}
/**
* Allows to filter indices if the provided query rewrites to
* match_none
on every shard.
*
* API name: {@code index_filter}
*/
@Nullable
public final Query indexFilter() {
return this.indexFilter;
}
/**
* Required - Extends the time to live of the corresponding point in time.
*
* API name: {@code keep_alive}
*/
public final Time keepAlive() {
return this.keepAlive;
}
/**
* Specifies the node or shard the operation should be performed on. Random by
* default.
*
* API name: {@code preference}
*/
@Nullable
public final String preference() {
return this.preference;
}
/**
* Custom value used to route operations to a specific shard.
*
* API name: {@code routing}
*/
@Nullable
public final String routing() {
return this.routing;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if (this.indexFilter != null) {
generator.writeKey("index_filter");
this.indexFilter.serialize(generator, mapper);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link OpenPointInTimeRequest}.
*/
public static class Builder extends RequestBase.AbstractBuilder
implements
ObjectBuilder {
@Nullable
private Boolean allowPartialSearchResults;
@Nullable
private List expandWildcards;
@Nullable
private Boolean ignoreUnavailable;
private List index;
@Nullable
private Query indexFilter;
private Time keepAlive;
@Nullable
private String preference;
@Nullable
private String routing;
/**
* If false
, creating a point in time request when a shard is
* missing or unavailable will throw an exception. If true
, the
* point in time will contain all the shards that are available at the time of
* the request.
*
* API name: {@code allow_partial_search_results}
*/
public final Builder allowPartialSearchResults(@Nullable Boolean value) {
this.allowPartialSearchResults = value;
return this;
}
/**
* Type of index that wildcard patterns can match. If the request can target
* data streams, this argument determines whether wildcard expressions match
* hidden data streams. Supports comma-separated values, such as
* open,hidden
. Valid values are: all
,
* open
, closed
, hidden
,
* none
.
*
* API name: {@code expand_wildcards}
*
* Adds all elements of list
to expandWildcards
.
*/
public final Builder expandWildcards(List list) {
this.expandWildcards = _listAddAll(this.expandWildcards, list);
return this;
}
/**
* Type of index that wildcard patterns can match. If the request can target
* data streams, this argument determines whether wildcard expressions match
* hidden data streams. Supports comma-separated values, such as
* open,hidden
. Valid values are: all
,
* open
, closed
, hidden
,
* none
.
*
* API name: {@code expand_wildcards}
*
* Adds one or more values to expandWildcards
.
*/
public final Builder expandWildcards(ExpandWildcard value, ExpandWildcard... values) {
this.expandWildcards = _listAdd(this.expandWildcards, value, values);
return this;
}
/**
* If false
, the request returns an error if it targets a missing
* or closed index.
*
* API name: {@code ignore_unavailable}
*/
public final Builder ignoreUnavailable(@Nullable Boolean value) {
this.ignoreUnavailable = value;
return this;
}
/**
* Required - A comma-separated list of index names to open point in time; use
* _all
or empty string to perform the operation on all indices
*
* API name: {@code index}
*
* Adds all elements of list
to index
.
*/
public final Builder index(List list) {
this.index = _listAddAll(this.index, list);
return this;
}
/**
* Required - A comma-separated list of index names to open point in time; use
* _all
or empty string to perform the operation on all indices
*
* API name: {@code index}
*
* Adds one or more values to index
.
*/
public final Builder index(String value, String... values) {
this.index = _listAdd(this.index, value, values);
return this;
}
/**
* Allows to filter indices if the provided query rewrites to
* match_none
on every shard.
*
* API name: {@code index_filter}
*/
public final Builder indexFilter(@Nullable Query value) {
this.indexFilter = value;
return this;
}
/**
* Allows to filter indices if the provided query rewrites to
* match_none
on every shard.
*
* API name: {@code index_filter}
*/
public final Builder indexFilter(Function> fn) {
return this.indexFilter(fn.apply(new Query.Builder()).build());
}
/**
* Required - Extends the time to live of the corresponding point in time.
*
* API name: {@code keep_alive}
*/
public final Builder keepAlive(Time value) {
this.keepAlive = value;
return this;
}
/**
* Required - Extends the time to live of the corresponding point in time.
*
* API name: {@code keep_alive}
*/
public final Builder keepAlive(Function> fn) {
return this.keepAlive(fn.apply(new Time.Builder()).build());
}
/**
* Specifies the node or shard the operation should be performed on. Random by
* default.
*
* API name: {@code preference}
*/
public final Builder preference(@Nullable String value) {
this.preference = value;
return this;
}
/**
* Custom value used to route operations to a specific shard.
*
* API name: {@code routing}
*/
public final Builder routing(@Nullable String value) {
this.routing = value;
return this;
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link OpenPointInTimeRequest}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public OpenPointInTimeRequest build() {
_checkSingleUse();
return new OpenPointInTimeRequest(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Json deserializer for {@link OpenPointInTimeRequest}
*/
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer
.lazy(Builder::new, OpenPointInTimeRequest::setupOpenPointInTimeRequestDeserializer);
protected static void setupOpenPointInTimeRequestDeserializer(
ObjectDeserializer op) {
op.add(Builder::indexFilter, Query._DESERIALIZER, "index_filter");
}
// ---------------------------------------------------------------------------------------------
/**
* Endpoint "{@code open_point_in_time}".
*/
public static final Endpoint _ENDPOINT = new SimpleEndpoint<>(
"es/open_point_in_time",
// Request method
request -> {
return "POST";
},
// Request path
request -> {
final int _index = 1 << 0;
int propsSet = 0;
propsSet |= _index;
if (propsSet == (_index)) {
StringBuilder buf = new StringBuilder();
buf.append("/");
SimpleEndpoint.pathEncode(request.index.stream().map(v -> v).collect(Collectors.joining(",")), buf);
buf.append("/_pit");
return buf.toString();
}
throw SimpleEndpoint.noPathTemplateFound("path");
},
// Path parameters
request -> {
Map params = new HashMap<>();
final int _index = 1 << 0;
int propsSet = 0;
propsSet |= _index;
if (propsSet == (_index)) {
params.put("index", request.index.stream().map(v -> v).collect(Collectors.joining(",")));
}
return params;
},
// Request parameters
request -> {
Map params = new HashMap<>();
if (request.routing != null) {
params.put("routing", request.routing);
}
if (request.allowPartialSearchResults != null) {
params.put("allow_partial_search_results", String.valueOf(request.allowPartialSearchResults));
}
if (request.ignoreUnavailable != null) {
params.put("ignore_unavailable", String.valueOf(request.ignoreUnavailable));
}
if (ApiTypeHelper.isDefined(request.expandWildcards)) {
params.put("expand_wildcards",
request.expandWildcards.stream().map(v -> v.jsonValue()).collect(Collectors.joining(",")));
}
if (request.preference != null) {
params.put("preference", request.preference);
}
params.put("keep_alive", request.keepAlive._toJsonString());
return params;
}, SimpleEndpoint.emptyMap(), true, OpenPointInTimeResponse._DESERIALIZER);
}